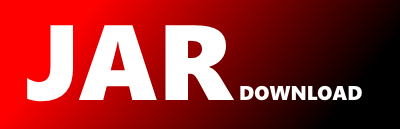
net.anotheria.portalkit.services.account.AccountQuery Maven / Gradle / Ivy
package net.anotheria.portalkit.services.account;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import net.anotheria.portalkit.services.common.AccountId;
/**
* Query parameters.
*
* @author Alexandr Bolbat
*/
public final class AccountQuery implements Serializable {
/**
* Basic serialVersionUID variable.
*/
private static final long serialVersionUID = -559248313830424493L;
/**
* Account identifiers.
*/
private final List ids;
/**
* Account name mask.
*/
private final String nameMask;
/**
* Account email mask.
*/
private final String emailMask;
/**
* Account identifier mask.
*/
private final String idMask;
/**
* Included types.
*/
private final List typesIncluded;
/**
* Excluded types.
*/
private final List typesExcluded;
/**
* Included statuses.
*/
private final List statusesIncluded;
/**
* Excluded statuses.
*/
private final List statusesExcluded;
/**
* Registered from.
*/
private final Long registeredFrom;
/**
* Registered till.
*/
private final Long registeredTill;
/**
* {@link List} of {@link String} tenants.
*/
private final List tenants;
/**
* User brand.
*/
private final String brand;
private AccountQuery(Builder builder) {
this.ids = builder.getIds();
this.nameMask = builder.getNameMask();
this.emailMask = builder.getEmailMask();
this.idMask = builder.getIdMask();
this.typesIncluded = builder.getTypesIncluded();
this.typesExcluded = builder.getTypesExcluded();
this.statusesIncluded = builder.getStatusesIncluded();
this.statusesExcluded = builder.getStatusesExcluded();
this.registeredFrom = builder.getRegisteredFrom();
this.registeredTill = builder.getRegisteredTill();
this.tenants = builder.getTenants();
this.brand = builder.getBrand();
}
public List getIds() {
return ids;
}
public String getNameMask() {
return nameMask;
}
public String getEmailMask() {
return emailMask;
}
public String getIdMask() {
return idMask;
}
public List getTypesIncluded() {
return typesIncluded;
}
public List getTypesExcluded() {
return typesExcluded;
}
public List getStatusesIncluded() {
return statusesIncluded;
}
public List getStatusesExcluded() {
return statusesExcluded;
}
public Long getRegisteredFrom() {
return registeredFrom;
}
public Long getRegisteredTill() {
return registeredTill;
}
public List getTenants() {
return tenants;
}
public String getBrand() {
return brand;
}
@Override
public String toString() {
return "AccountQuery{" +
"ids=" + ids +
", nameMask='" + nameMask + '\'' +
", emailMask='" + emailMask + '\'' +
", idMask='" + idMask + '\'' +
", typesIncluded=" + typesIncluded +
", typesExcluded=" + typesExcluded +
", statusesIncluded=" + statusesIncluded +
", statusesExcluded=" + statusesExcluded +
", registeredFrom=" + registeredFrom +
", registeredTill=" + registeredTill +
", tenants=" + tenants +
", brand='" + brand + '\'' +
'}';
}
/**
* Builder for {@link AccountQuery}.
*/
public static class Builder {
private List ids = new ArrayList<>();
private String nameMask;
private String emailMask;
private String idMask;
private List typesIncluded = new ArrayList<>();
private List typesExcluded = new ArrayList<>();
private List statusesIncluded = new ArrayList<>();
private List statusesExcluded = new ArrayList<>();
private Long registeredFrom;
private Long registeredTill;
private List tenants = new ArrayList<>();
private String brand;
/**
* Default constructor of builder.
*/
public Builder() {}
/**
* Build {@link AccountQuery}.
*
* @return constructed {@link AccountQuery}
*/
public AccountQuery build() {
return new AccountQuery(this);
}
public Builder setIds(List ids) {
this.ids = ids;
return this;
}
public Builder setNameMask(String nameMask) {
this.nameMask = nameMask;
return this;
}
public Builder setEmailMask(String emailMask) {
this.emailMask = emailMask;
return this;
}
public Builder setIdMask(String idMask) {
this.idMask = idMask;
return this;
}
public Builder setTypesIncluded(List typesIncluded) {
this.typesIncluded = typesIncluded;
return this;
}
public Builder setTypesExcluded(List typesExcluded) {
this.typesExcluded = typesExcluded;
return this;
}
public Builder setStatusesIncluded(List statusesIncluded) {
this.statusesIncluded = statusesIncluded;
return this;
}
public Builder setStatusesExcluded(List statusesExcluded) {
this.statusesExcluded = statusesExcluded;
return this;
}
public Builder setRegisteredFrom(Long registeredFrom) {
this.registeredFrom = registeredFrom;
return this;
}
public Builder setRegisteredTill(Long registeredTill) {
this.registeredTill = registeredTill;
return this;
}
public Builder addTenants(List tenants) {
this.tenants.addAll(tenants);
return this;
}
public Builder addTenant(String tenant) {
tenants.add(tenant);
return this;
}
public Builder setBrand(String brand) {
this.brand = brand;
return this;
}
List getIds() {
return ids;
}
String getNameMask() {
return nameMask;
}
String getEmailMask() {
return emailMask;
}
String getIdMask() {
return idMask;
}
List getTypesIncluded() {
return typesIncluded;
}
List getTypesExcluded() {
return typesExcluded;
}
List getStatusesIncluded() {
return statusesIncluded;
}
List getStatusesExcluded() {
return statusesExcluded;
}
Long getRegisteredFrom() {
return registeredFrom;
}
Long getRegisteredTill() {
return registeredTill;
}
List getTenants() {
return tenants;
}
public String getBrand() {
return brand;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy