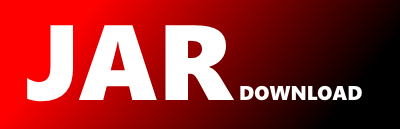
net.anotheria.access.impl.SecurityBox Maven / Gradle / Ivy
The newest version!
package net.anotheria.access.impl;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import net.anotheria.access.Role;
import net.anotheria.anoprise.dualcrud.CrudSaveable;
/**
* A {@link SecurityBox} holds information about roles granted to a specific security object owner.
*
* @author Leon Rosenberg, Alexandr Bolbat
*/
public class SecurityBox implements CrudSaveable {
/**
* Basic serialVersionUID variable.
*/
private static final long serialVersionUID = 6151326668037727657L;
/**
* Unique owner id.
*/
private String ownerId;
/**
* Assigned roles.
*/
private Map roles;
/**
* Default constructor.
*/
public SecurityBox() {
roles = new HashMap();
}
/**
* Public constructor.
*
* @param aOwnerId
* - unique owner id
*/
public SecurityBox(final String aOwnerId) {
this();
this.ownerId = aOwnerId;
}
public String getOwnerId() {
return ownerId;
}
public void setOwnerId(final String aOwnerId) {
this.ownerId = aOwnerId;
}
/**
* Has {@link Role}.
*
* @param roleName
* - role name
* @return true
if has or false
*/
public boolean hasRole(final String roleName) {
return roles.containsKey(roleName);
}
/**
* Add {@link Role}.
*
* @param role
* - {@link Role} to add, can't be null
*/
public void addRole(Role role) {
if (role == null)
throw new IllegalArgumentException("Can't add an empty role");
roles.put(role.getName(), role);
}
/**
* Remove {@link Role}.
*
* @param roleName
* - {@link Role} name
*/
public void removeRole(final String roleName) {
roles.remove(roleName);
}
/**
* Get owned roles names.
*
* @return {@link List} of {@link String}
*/
public List getOwnedRoles() {
return new ArrayList(roles.keySet());
}
/**
* Get owned roles.
*
* @return {@link List} of {@link Role}
*/
public List getRoles() {
return new ArrayList(roles.values());
}
/**
* Get user id, the same as owner id.
*
* @return {@link String}
*/
public String getUserId() {
return ownerId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy