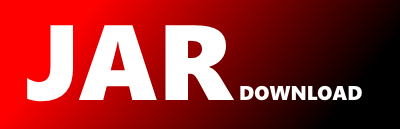
net.anotheria.asg.generator.model.DataFacadeGenerator Maven / Gradle / Ivy
package net.anotheria.asg.generator.model;
import java.util.ArrayList;
import java.util.List;
import net.anotheria.asg.generator.Context;
import net.anotheria.asg.generator.FileEntry;
import net.anotheria.asg.generator.GeneratedClass;
import net.anotheria.asg.generator.GeneratorDataRegistry;
import net.anotheria.asg.generator.IGenerateable;
import net.anotheria.asg.generator.IGenerator;
import net.anotheria.asg.generator.TypeOfClass;
import net.anotheria.asg.generator.meta.MetaContainerProperty;
import net.anotheria.asg.generator.meta.MetaDocument;
import net.anotheria.asg.generator.meta.MetaGenericProperty;
import net.anotheria.asg.generator.meta.MetaListProperty;
import net.anotheria.asg.generator.meta.MetaProperty;
import net.anotheria.asg.generator.meta.MetaTableProperty;
import net.anotheria.util.StringUtils;
/**
* This generator generates the data facade - the interface which defines the behaviour of the document and its attributes. It also generates the
* sort type and the builder.
* @author another
*
*/
public class DataFacadeGenerator extends AbstractDataObjectGenerator implements IGenerator{
public static final String PROPERTY_DECLARATION = "public static final String ";
/**
* The id property.
*/
private final MetaProperty id = new MetaProperty("id",MetaProperty.Type.STRING);
public List generate(IGenerateable gdoc){
MetaDocument doc = (MetaDocument)gdoc;
//System.out.println(ret);
List ret = new ArrayList();
ret.add(new FileEntry(generateDocument(doc)));
ret.add(new FileEntry(generateSortType(doc)));
ret.add(new FileEntry(generateXMLHelper(doc)));
ret.add(new FileEntry(generateBuilder(doc)));
return ret;
}
public String getDocumentName(MetaDocument doc){
return doc.getName();
}
public static String getSortTypeName(MetaDocument doc){
return doc.getName()+"SortType";
}
public static String getXMLHelperName(MetaDocument doc){
return doc.getName()+"XMLHelper";
}
private GeneratedClass generateBuilder(MetaDocument doc){
GeneratedClass clazz = new GeneratedClass();
startNewJob(clazz);
clazz.setPackageName(getPackageName(doc));
clazz.setName(getDocumentBuilderName(doc));
clazz.addInterface("Builder<"+doc.getName()+">");
clazz.addImport(net.anotheria.asg.data.Builder.class);
startClassBody();
for (int i=0; i properties = extractSortableProperties(doc);
if (properties.size()==0)
return null;
GeneratedClass clazz = new GeneratedClass();
startNewJob(clazz);
clazz.setPackageName(getPackageName(doc));
clazz.addImport("net.anotheria.util.sorter.SortType");
clazz.setName(getSortTypeName(doc));
clazz.setParent("SortType");
startClassBody();
int lastIndex = 1;
for (int i=0; i0 )
langArray.append(",");
langArray.append(quote(l));
}
appendStatement("public static final String[] LANGUAGES = new String[]{",langArray.toString(),"}");
}
//generates generic to xml method
if (GeneratorDataRegistry.getInstance().getContext().areLanguagesSupported()){
appendString("private static XMLNode _toXML("+doc.getName()+" object, String[] languages){");
}else{
appendString("private static XMLNode _toXML("+doc.getName()+" object){");
}
increaseIdent();
appendStatement("XMLNode ret = new XMLNode("+quote(doc.getName())+")");
appendStatement("ret.addAttribute(new XMLAttribute("+quote("id")+", object.getId()))");
emptyline();
for (MetaProperty p : doc.getProperties()){
generatePropertyToXMLMethod(p);
}
emptyline();
for (MetaProperty p : doc.getLinks()){
generatePropertyToXMLMethod(p);
}
emptyline();
if (GeneratorDataRegistry.getInstance().getContext().areLanguagesSupported()){
appendString("if(object instanceof MultilingualObject){");
increaseIdent();
appendStatement("MultilingualObject multilangDoc = (MultilingualObject) object");
appendStatement("ret.addChildNode(XMLHelper.createXMLNodeForBooleanValue("+quote("multilingualDisabled")+", null, multilangDoc.isMultilingualDisabledInstance()))");
closeBlockNEW();
}
appendStatement("return ret");
closeBlockNEW();
emptyline();
appendString("public static XMLNode toXML("+doc.getName()+" object){");
increaseIdent();
if (GeneratorDataRegistry.getInstance().getContext().areLanguagesSupported()){
appendStatement("return _toXML(object, LANGUAGES)");
}else{
appendStatement("return _toXML(object)");
}
closeBlockNEW();
emptyline();
if (GeneratorDataRegistry.getInstance().getContext().areLanguagesSupported()){
//generates toXML method for a single language
appendString("public static XMLNode toXML("+doc.getName()+" object, String... languages){");
increaseIdent();
appendString("if (languages==null || languages.length==0)");
appendIncreasedStatement("return toXML(object)");
appendStatement("return _toXML(object, languages)");
closeBlockNEW();
emptyline();
}
appendString("public static "+doc.getName()+" fromXML(XMLNode node){");
increaseIdent();
appendStatement("return null");
closeBlockNEW();
emptyline();
//ret += generatePropertyAccessMethods(doc);
//ret += generateAdditionalMethods(doc);
return clazz;
}
private void generatePropertyToXMLMethod(MetaProperty p){
if (p instanceof MetaTableProperty){
generateTablePropertyGetterMethods((MetaTableProperty)p);
return;
}
if (p instanceof MetaListProperty){
generateListPropertyToXMLMethods((MetaListProperty)p);
return;
}
if (GeneratorDataRegistry.getInstance().getContext().areLanguagesSupported() && p.isMultilingual()){
generatePropertyToXMLMethodMultilingual(p);
return;
}
appendStatement("ret.addChildNode(XMLHelper.createXMLNodeFor"+StringUtils.capitalize(p.getType().getName())+"Value("+quote(p.getName())+", null, object.get"+p.getAccesserName()+"() ))");
}
private void generatePropertyToXMLMethodMultilingual(MetaProperty p){
String callArr = "";
for (String l : GeneratorDataRegistry.getInstance().getContext().getLanguages()){
if (callArr.length()>0)
callArr += ", ";
callArr += "object.get"+p.getAccesserName(l)+"()";
}
appendStatement("ret.addChildNode(XMLHelper.createXMLNodeFor"+StringUtils.capitalize(p.getType().getName())+"Value("+quote(p.getName())+", languages , "+callArr+" ))");
}
private void generateListPropertyToXMLMethods(MetaListProperty p){
appendStatement("ret.addChildNode(XMLHelper.createXMLNodeForListValue("+quote(p.getName())+", " + quote(p.getContainedProperty().getType().getName()) + ", object.get"+p.getAccesserName()+"()))");
//Alternative variant to maintain current approach:
//XMLHelper.createXMLNodeForXXXValue methods to XMLHelper must be added
// MetaProperty tmp = new MetaGenericProperty(p.getName(), "list" + StringUtils.capitalize(p.getType()), p.getContainedProperty());
// if (p.isMultilingual())
// tmp.setMultilingual(true);
// generatePropertyToXMLMethod(tmp);
// MetaProperty tmp = new MetaGenericProperty(p.getName(), "list", p.getContainedProperty());
// if (p.isMultilingual())
// tmp.setMultilingual(true);
// generatePropertyToXMLMethod(tmp);
}
/*
private String generatePropertyAccessMethods(MetaDocument doc){
String ret = "";
ret += _generatePropertyAccessMethods(doc.getProperties());
ret += _generatePropertyAccessMethods(doc.getLinks());
return ret;
}
private String _generatePropertyAccessMethods(List properties){
String ret = "";
for (int i=0; i propertyList){
for (int i=0; i columns = ((MetaTableProperty)p).getColumns();
for (int t=0; t properties){
for (int i=0; i properties){
for (int i=0; i columns = p.getColumns();
for (int t=0; t columns = p.getColumns();
for (int t=0; t columns = p.getColumns();
for (int t=0; t columns = p.getColumns();
for (int t=0; t properties = doc.getProperties();
for (MetaProperty p : properties){
if (p instanceof MetaContainerProperty)
generateContainerMethods((MetaContainerProperty)p);
if (p instanceof MetaTableProperty)
generateTableMethods((MetaTableProperty)p);
if (p instanceof MetaListProperty)
generateListMethods((MetaListProperty)p);
}
}
private void generateContainerMethods(MetaContainerProperty container){
if (container.isMultilingual())
generateContainerMethodsMultilingual(container);
appendComment("Returns the number of elements in the \""+container.getName()+"\" container");
appendString("public int "+getContainerSizeGetterName(container)+"();");
emptyline();
}
private void generateContainerMethodsMultilingual(MetaContainerProperty container){
for (String l : GeneratorDataRegistry.getInstance().getContext().getLanguages()){
appendComment("Returns the number of elements in the \""+container.getName()+"\" container");
appendString("public int "+getContainerSizeGetterName(container, l)+"();");
emptyline();
}
}
private void generateListMethods(MetaListProperty list){
if (list.isMultilingual())
generateListMethodsMultilingual(list);
MetaProperty c = list.getContainedProperty();
appendComment("Adds a new element to the list.");
String decl = "public void "+getContainerEntryAdderName(list)+"(";
decl += c.toJavaType()+" "+c.getName();
decl += ");";
appendString(decl);
emptyline();
appendComment("Removes the element at position index from the list.");
appendString("public void "+getContainerEntryDeleterName(list)+"(int index);");
emptyline();
appendComment("Swaps elements at positions index1 and index2 in the list.");
appendString("public void "+getContainerEntrySwapperName(list)+"(int index1, int index2);");
emptyline();
appendComment("Returns the element at the position index in the list.");
appendString("public "+c.toJavaType()+ " "+getListElementGetterName(list)+"(int index);");
emptyline();
}
private void generateListMethodsMultilingual(MetaListProperty list){
for (String l : GeneratorDataRegistry.getInstance().getContext().getLanguages()){
MetaProperty c = list.getContainedProperty();
appendComment("Adds a new element to the list.");
String decl = "public void "+getContainerEntryAdderName(list, l)+"(";
decl += c.toJavaType()+" "+c.getName();
decl += ");";
appendString(decl);
emptyline();
appendComment("Removes the element at position index from the list.");
appendString("public void "+getContainerEntryDeleterName(list, l)+"(int index);");
emptyline();
appendComment("Swaps elements at positions index1 and index2 in the list.");
appendString("public void "+getContainerEntrySwapperName(list, l)+"(int index1, int index2);");
emptyline();
appendComment("Returns the element at the position index in the list.");
appendString("public "+c.toJavaType()+ " "+getListElementGetterName(list, l)+"(int index);");
emptyline();
}
}
private void generateTableMethods(MetaTableProperty table){
List columns = table.getColumns();
String decl = "public void "+getContainerEntryAdderName(table)+"(";
for (int i =0; i get"+StringUtils.capitalize(table.getName())+"Row(int index);");
emptyline();
appendString("public List> "+getTableGetterName(table)+"();");
emptyline();
}
public static String getContainerSizeGetterName(MetaContainerProperty p){
return "get"+StringUtils.capitalize(p.getName())+"Size";
}
public static String getContainerSizeGetterName(MetaContainerProperty p, String language){
return "get"+StringUtils.capitalize(p.getName(language))+"Size";
}
public static String getTableGetterName(MetaTableProperty p){
return "get"+StringUtils.capitalize(p.getName())+"Table";
}
public static String getContainerEntryAdderName(MetaContainerProperty p){
return "add"+StringUtils.capitalize(p.getName())+p.getContainerEntryName();
}
public static String getContainerEntryAdderName(MetaContainerProperty p, String language){
return "add"+StringUtils.capitalize(p.getName(language))+p.getContainerEntryName();
}
public static String getContainerEntryDeleterName(MetaContainerProperty p){
return "remove"+StringUtils.capitalize(p.getName())+p.getContainerEntryName();
}
public static String getContainerEntryDeleterName(MetaContainerProperty p, String language){
return "remove"+StringUtils.capitalize(p.getName(language))+p.getContainerEntryName();
}
public static String getContainerEntrySwapperName(MetaContainerProperty p){
return "swap"+StringUtils.capitalize(p.getName())+p.getContainerEntryName();
}
public static String getContainerEntrySwapperName(MetaContainerProperty p, String language){
return "swap"+StringUtils.capitalize(p.getName(language))+p.getContainerEntryName();
}
public static String getListElementGetterName(MetaListProperty list){
return "get"+StringUtils.capitalize(list.getName())+list.getContainerEntryName();
}
public static String getListElementGetterName(MetaListProperty list, String language){
return "get"+StringUtils.capitalize(list.getName(language))+list.getContainerEntryName();
}
public static String getDocumentFactoryName(MetaDocument doc){
return doc.getName()+"Factory";
}
public static final String getDocumentFactoryImport(Context context, MetaDocument doc){
return context.getDataPackageName(doc)+"."+getDocumentFactoryName(doc);
}
public String getDataObjectImplName(MetaDocument doc){
throw new AssertionError("Shouln't be called, since the facade has no impl");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy