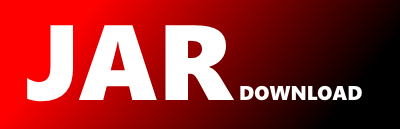
net.anotheria.asg.generator.parser.XMLDataParser Maven / Gradle / Ivy
package net.anotheria.asg.generator.parser;
import java.io.IOException;
import java.io.StringReader;
import java.util.ArrayList;
import java.util.List;
import net.anotheria.asg.generator.meta.FederatedDocumentMapping;
import net.anotheria.asg.generator.meta.MetaDocument;
import net.anotheria.asg.generator.meta.MetaEnumerationProperty;
import net.anotheria.asg.generator.meta.MetaFederationModule;
import net.anotheria.asg.generator.meta.MetaLink;
import net.anotheria.asg.generator.meta.MetaListProperty;
import net.anotheria.asg.generator.meta.MetaModule;
import net.anotheria.asg.generator.meta.MetaProperty;
import net.anotheria.asg.generator.meta.MetaTableProperty;
import net.anotheria.asg.generator.meta.ModuleParameter;
import net.anotheria.asg.generator.meta.StorageType;
import net.anotheria.util.StringUtils;
import org.jdom.Document;
import org.jdom.Element;
import org.jdom.JDOMException;
import org.jdom.input.SAXBuilder;
/**
* XMLParser for the data definition files.
* @author another
*/
public final class XMLDataParser {
@SuppressWarnings("unchecked")
public static final List parseModules(String content){
SAXBuilder reader = new SAXBuilder();
reader.setValidation(false);
List ret = new ArrayList();
try{
Document doc = reader.build(new StringReader(content));
Element root = doc.getRootElement();
List modules = root.getChildren("module");
for (int i=0; i childs = m.getChildren("document");
for (int i=0; i listeners = m.getChildren("listener");
for (int i=0; i federated = m.getChildren("federatedmodule");
for (Element e: federated){
((MetaFederationModule)mod).addFederatedModule(e.getAttributeValue("key"), e.getAttributeValue("name"));
}
@SuppressWarnings("unchecked")List mappings = m.getChildren("mapping");
for (Element e : mappings){
String sourceDocumentName = e.getAttributeValue("sourceDocument");
String targetDocument = e.getAttributeValue("targetDocument");
String t[] = StringUtils.tokenize(targetDocument, '.');
FederatedDocumentMapping mapping = new FederatedDocumentMapping();
mapping.setSourceDocument(sourceDocumentName);
mapping.setTargetKey(t[0]);
mapping.setTargetDocument(t[1]);
((MetaFederationModule)mod).addMapping(mapping);
}
}
//parse parameters
@SuppressWarnings("unchecked")List parameters = m.getChildren("parameter");
for (Element p : parameters){
ModuleParameter param = new ModuleParameter(p.getAttributeValue("name"), p.getAttributeValue("value"));
System.out.println("Parsed module parameter "+param+" for module "+mod.getName());
mod.addModuleParameter(param);
}
Element optionsEleemnt = m.getChild("options");
if (optionsEleemnt!=null)
mod.setModuleOptions(OptionsParser.parseOptions(optionsEleemnt));
return mod;
}
/**
* Parses a document from a document tag.
* @param d
* @return
*/
private static final MetaDocument parseDocument(Element d){
MetaDocument doc = new MetaDocument(d.getAttributeValue("name"));
@SuppressWarnings("unchecked")List properties = d.getChildren("property");
@SuppressWarnings("unchecked")List links = d.getChildren("link");
for (int i=0; i>"+e.getAttributeValue("name")+"<<-");
if (e.getName().equals("property"))
return parseProperty(e);
if (e.getName().equals("link"))
return parseLink(e);
throw new RuntimeException("Unknown attribute type:"+e.getName());
}
/**
* Parses a property. May call subsequent methods depending on property type.
* @param p
* @return
*/
private static final MetaProperty parseProperty(Element p){
String name = p.getAttributeValue("name");
String typeStr = p.getAttributeValue("type");
if (typeStr.equals("table"))
return parseTable(p);
if (typeStr.equals("list"))
return parseList(p);
if (typeStr.equals("enumeration"))
return parseEnumeration(p);
MetaProperty.Type type = MetaProperty.Type.findTypeByName(typeStr);
if(type == null)
throw new IllegalArgumentException("Uknown type <" + typeStr + "> for property def " + p);
MetaProperty ret = new MetaProperty(name, type);
String multilingual = p.getAttributeValue("multilingual");
if (multilingual!=null && multilingual.length()>0 && multilingual.equalsIgnoreCase("true"))
ret.setMultilingual(true);
// String validatorName = p.getAttributeValue("validator");
// if (validatorName != null ) {
// MetaValidator validator = GeneratorDataRegistry.getInstance().getValidator(validatorName);
// if (validator == null) {
// throw new IllegalArgumentException("Uknown validator <" + validatorName + "> for property def " + p+
// ". Check that you have validators-def.xml in classpath and validator is present there.");
// }
// ret.setValidator(validator);
// }
return ret;
}
/**
* Parser for enumeration properties.
* @param p
* @return
*/
private static final MetaEnumerationProperty parseEnumeration(Element p){
String name = p.getAttributeValue("name");
String enumeration = p.getAttributeValue("enumeration");
MetaEnumerationProperty ret = new MetaEnumerationProperty(name, MetaProperty.Type.INT);
ret.setEnumeration(enumeration);
return ret;
}
/**
* Parses table properties.
* @param p
* @return
*/
private static final MetaProperty parseTable(Element p){
String name = p.getAttributeValue("name");
MetaTableProperty ret = new MetaTableProperty(name);
@SuppressWarnings("unchecked")List columns = p.getChildren("column");
for (int i=0; i0 && multilingual.equalsIgnoreCase("true"))
ret.setMultilingual(true);
return ret;
}
/**
* Parses a link.
* @param p
* @return
*/
private static final MetaLink parseLink(Element p){
String name = p.getAttributeValue("name");
String linkType = p.getAttributeValue("type");
String target = p.getAttributeValue("target");
String decorationStr = p.getAttributeValue("decoration");
List decoration;
if(StringUtils.isEmpty(decorationStr)){
decoration = new ArrayList();
decoration.add("name");
}else{
decoration = StringUtils.tokenize2list(decorationStr, ',');
}
MetaLink l = new MetaLink(name);
l.setLinkTarget(target);
l.setLinkType(linkType);
l.setLinkDecoration(decoration);
String multilingual = p.getAttributeValue("multilingual");
if (multilingual!=null && multilingual.length()>0 && multilingual.equalsIgnoreCase("true"))
l.setMultilingual(true);
return l;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy