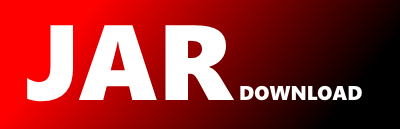
net.anotheria.asg.generator.view.jsp.JspGenerator Maven / Gradle / Ivy
package net.anotheria.asg.generator.view.jsp;
import net.anotheria.asg.generator.*;
import net.anotheria.asg.generator.forms.meta.*;
import net.anotheria.asg.generator.meta.*;
import net.anotheria.asg.generator.view.CMSMappingsConfiguratorGenerator;
import net.anotheria.asg.generator.view.action.ModuleActionsGenerator;
import net.anotheria.asg.generator.view.meta.*;
import net.anotheria.util.StringUtils;
import java.util.ArrayList;
import java.util.List;
/**
* Generates the jsps for the edit view.
* @author another
*/
public class JspGenerator extends AbstractJSPGenerator implements IGenerator{
/**
* Currently generated section.
*/
private MetaSection currentSection;
/* (non-Javadoc)
* @see net.anotheria.anodoc.generator.IGenerator#generate(net.anotheria.anodoc.generator.IGenerateable, net.anotheria.anodoc.generator.Context)
*/
public List generate(IGenerateable g) {
try{
List files = new ArrayList();
MetaView view = (MetaView)g;
files.add(new FileEntry(generateMenu(view)));
files.add(new FileEntry(generateFooter(view, FOOTER_SELECTION_CMS, getFooterName(view))));
files.add(new FileEntry(generateSearchPage()));
FileEntry versionInfoPage = new FileEntry(generateVersionInfoPage());
versionInfoPage.setType(".jsp");
files.add(versionInfoPage);
for (int i=0; i dialogs = section.getDialogs();
for (int d=0; d");
increaseIdent();
openTR();
appendString("");
appendString("");
increaseIdent();
appendString("");
appendIncreasedString(" ");
appendString(" ");
appendString("");
appendIncreasedString("\"> ");
appendString(" ");
decreaseIdent();
appendString(" ");
decreaseIdent();
appendString(" ");
closeTR();
decreaseIdent();
appendString("");
append(getBaseJSPFooter());
return jsp;
}
private GeneratedJSPFile generateContainerPage(MetaModuleSection section, MetaDocument doc, MetaContainerProperty p){
if (p instanceof MetaListProperty)
return new ListPageJspGenerator().generate(section, doc, (MetaListProperty)p);
if (p instanceof MetaTableProperty)
return new TablePageJspGenerator().generate(section, doc, (MetaTableProperty)p);
throw new RuntimeException("Unsupported container: "+p);
}
private GeneratedJSPFile generateCSVExport(MetaModuleSection section, MetaView view){
GeneratedJSPFile jsp = new GeneratedJSPFile();
startNewJob(jsp);
jsp.setName(getExportAsCSVPageName(section.getDocument()));
jsp.setPackage(getContext().getJspPackageName(section.getModule()));
ident = 0;
append(getBaseCSVHeader());
currentSection = section;
MetaDocument doc = section.getDocument();
String entryName = doc.getName().toLowerCase();
List elements = createMultilingualList(section.getElements(),doc);
String headerLine = "";
for (MetaViewElement element : elements){
String lang = getElementLanguage(element);
boolean multilangEl = element instanceof MultilingualFieldElement;
String tag = multilangEl && lang != null ? doc.getField(element.getName()).getName(lang) : generateTag(element);
if (tag==null)
continue;
headerLine += tag+";";
}
appendString(headerLine);
appendString("<%--");
String bodyLine = "--%>";
for (MetaViewElement element : elements) {
String lang = getElementLanguage(element);
boolean multilangEl = element instanceof MultilingualFieldElement;
String tag = multilangEl && lang != null ? doc.getField(element.getName()).getName(lang) : generateTag(element);
if (tag==null)
continue;
bodyLine += " ;";
}
appendString(bodyLine);
appendString(" ");
return jsp;
}
private String generateTag(MetaViewElement elem){
if (!(elem instanceof MetaFieldElement))
return null;
return ((MetaFieldElement)elem).getName();
}
private GeneratedJSPFile generateXMLExport(MetaModuleSection section, MetaView view){
GeneratedJSPFile jsp = new GeneratedJSPFile();
startNewJob(jsp);
jsp.setName(getExportAsXMLPageName(section.getDocument()));
jsp.setPackage(getContext().getJspPackageName(section.getModule()));
ident = 0;
append(getBaseXMLHeader());
currentSection = section;
MetaDocument doc = section.getDocument();
appendString("");
appendString(" ");
return jsp;
}
private GeneratedJSPFile generateSearchPage(){
GeneratedJSPFile jsp = new GeneratedJSPFile();
startNewJob(jsp);
jsp.setName(getSearchResultPageName());
jsp.setPackage(GeneratorDataRegistry.getInstance().getContext().getPackageName(MetaModule.SHARED)+".jsp");
ident = 0;
append(getBaseJSPHeader());
appendString("");
appendString("");
increaseIdent();
appendString("");
increaseIdent();
appendString("Search result ");
generatePragmas();
appendString("");
appendString("");
appendString("");
decreaseIdent();
appendString("");
appendString("");
appendString("");
appendString("");
increaseIdent();
appendString("");
increaseIdent();
appendString("");
increaseIdent();
appendString("");
appendString("");
appendString("");
increaseIdent();
appendString("");
decreaseIdent();
// SAVE AND CLOSE BUTTONS SHOULD BE HERE
appendString("");
decreaseIdent();
appendString("");
appendString("");
appendString("");
appendString("");
appendString("");
appendString("");
appendString("Search result:
");
appendString("");
appendString("");
appendString("");
appendString("");
appendString("");
appendString("");
decreaseIdent();
appendString("");
appendString("");
append(getBaseJSPFooter());
return jsp;
}
private GeneratedJSPFile generateVersionInfoPage(){
GeneratedJSPFile jsp = new GeneratedJSPFile();
startNewJob(jsp);
jsp.setName(getVersionInfoPageName());
jsp.setPackage(GeneratorDataRegistry.getInstance().getContext().getPackageName(MetaModule.SHARED)+".jsp");
ident = 0;
append(getBaseJSPHeader());
appendString("");
appendString("");
increaseIdent();
appendString("");
increaseIdent();
appendString("VersionInfo for ");
//appendString("");
generatePragmas();
appendString("");
decreaseIdent();
appendString("");
appendString("");
increaseIdent();
//appendString("");
int colspan = 2;
appendString("");
increaseIdent();
appendString("");
increaseIdent();
appendString("
");
decreaseIdent();
appendString(" ");
//write header
appendString("");
increaseIdent();
appendString("VersionInfo for document ");
decreaseIdent();
appendString(" ");
appendString("");
appendIncreasedString("Document name: ");
appendIncreasedString(" ");
appendString(" ");
appendString("");
appendIncreasedString("Document type: ");
appendIncreasedString(" ");
appendString(" ");
appendString("");
appendIncreasedString("Last update: ");
appendIncreasedString(" ");
appendString(" ");
appendString("");
increaseIdent();
appendString(" ");
decreaseIdent();
appendString(" ");
appendString("");
appendIncreasedString(" ");
appendIncreasedString("Back ");
appendString(" ");
decreaseIdent();
appendString("
");
decreaseIdent();
//appendString("");
appendString("");
decreaseIdent();
appendString("");
appendString("");
append(getBaseJSPFooter());
return jsp;
}
public String getFormIncludePageName(MetaForm form){
return StringUtils.capitalize(form.getId())+"AutoForm";
}
private String generateFormField(MetaFormSingleField field, String className){
return generateFormField(field.getName(), field, className);
}
private String generateFormField(String name, MetaFormSingleField field, String className){
if (field.getType().equals("boolean"))
return "";
if (field.getType().equals("text"))
return "";
if (field.getType().equals("string"))
return "";
if (field.getType().equals("password"))
return "";
if (field.getType().equals("spacer"))
return "";
throw new RuntimeException("Unsupported field type: "+field.getType());
}
public String generateFormInclude(MetaForm form){
System.out.println("generating form "+form);
String ret = "";
resetIdent();
String formName = CMSMappingsConfiguratorGenerator.getFormName(form);
ret += getBaseJSPHeader();
appendString("");
appendString("");
increaseIdent();
appendString("");
decreaseIdent();
appendString("
");
ret += getBaseJSPFooter();
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy