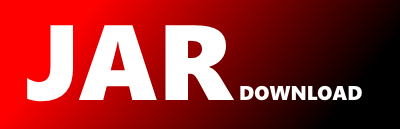
net.anotheria.anosite.photoserver.api.upload.UploadStatusAO Maven / Gradle / Ivy
The newest version!
package net.anotheria.anosite.photoserver.api.upload;
import net.anotheria.util.NumberUtils;
import org.json.simple.JSONObject;
/**
* Status for a current upload.
*
* @author otoense
* @version $Id: $Id
*/
public class UploadStatusAO {
/** Constant STATUS_NOT_STARTED=2
*/
public static final int STATUS_NOT_STARTED = 2;
/** Constant STATUS_UPLOADING=1
*/
public static final int STATUS_UPLOADING = 1;
/** Constant STATUS_FINISHED=0
*/
public static final int STATUS_FINISHED = 0;
/** Constant STATUS_ERROR_MAX_FILESIZE_EXCEEDED=-1
*/
public static final int STATUS_ERROR_MAX_FILESIZE_EXCEEDED = -1;
/** Constant STATUS_ERROR_UPLOADEXCEPTION=-2
*/
public static final int STATUS_ERROR_UPLOADEXCEPTION = -2;
/** Constant STATUS_ERROR_REJECTED=-3
*/
public static final int STATUS_ERROR_REJECTED = -3;
/** Constant STATUS_ERROR_NOTREGISTERED=-4
*/
public static final int STATUS_ERROR_NOTREGISTERED = -4;
private int progress;
private int status;
private String size;
private String filename;
private String id;
/**
* Constructor for UploadStatusAO.
*
* @param status a int.
*/
public UploadStatusAO(int status) {
this.status = status;
}
/**
* Getter for the field progress
.
*
* @return a int.
*/
public int getProgress() {
return progress;
}
/**
* Setter for the field progress
.
*
* @param progress a int.
*/
public void setProgress(int progress) {
this.progress = progress;
}
/**
* Getter for the field status
.
*
* @return a int.
*/
public int getStatus() {
return status;
}
/**
* Setter for the field status
.
*
* @param status a int.
*/
public void setStatus(int status) {
this.status = status;
}
/**
* Getter for the field size
.
*
* @return a {@link java.lang.String} object.
*/
public String getSize() {
return size;
}
/**
* Setter for the field size
.
*
* @param size a long.
*/
public void setSize(long size) {
this.size = NumberUtils.makeSizeString(size);
}
/**
* Getter for the field filename
.
*
* @return a {@link java.lang.String} object.
*/
public String getFilename() {
return filename;
}
/**
* Setter for the field filename
.
*
* @param filename a {@link java.lang.String} object.
*/
public void setFilename(String filename) {
this.filename = filename;
}
/**
* Getter for the field id
.
*
* @return a {@link java.lang.String} object.
*/
public String getId() {
return id;
}
/**
* Setter for the field id
.
*
* @param id a {@link java.lang.String} object.
*/
public void setId(String id) {
this.id = id;
}
/**
* toJSONString.
*
* @return a {@link java.lang.String} object.
*/
@SuppressWarnings("unchecked")
public String toJSONString() {
JSONObject json = new JSONObject();
json.put("progress", progress);
json.put("status", status);
json.put("size", size);
json.put("filename", filename);
json.put("id", id);
return json.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy