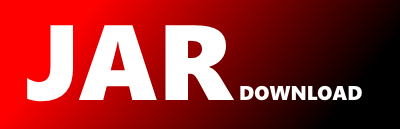
net.anotheria.anosite.photoserver.shared.vo.AlbumVO Maven / Gradle / Ivy
The newest version!
package net.anotheria.anosite.photoserver.shared.vo;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
/**
* Album meta information.
*
* @author Alexandr Bolbat
* @version $Id: $Id
*/
public class AlbumVO implements Serializable, Cloneable {
/**
* Basic serialVersionUID variable.
*/
private static final long serialVersionUID = -5297438096611917019L;
/**
* Album id. Unique.
*/
private long id;
/**
* User id.
*/
private String userId;
/**
* Id default album.
*/
private boolean isDefault;
/**
* Album name.
*/
private String name;
/**
* Album description.
*/
private String description;
/**
* Album photos order. This list can contain not all album pictures. Don't use this list as base for loading album photos. Only for implementing sorting for
* presentation.
*/
private List photosOrder = new ArrayList();
/**
* Getter for the field id
.
*
* @return a long.
*/
public long getId() {
return id;
}
/**
* Setter for the field id
.
*
* @param aId a long.
*/
public void setId(long aId) {
this.id = aId;
}
/**
* Getter for the field userId
.
*
* @return a {@link java.lang.String} object.
*/
public String getUserId() {
return userId;
}
/**
* Setter for the field userId
.
*
* @param aUserId a {@link java.lang.String} object.
*/
public void setUserId(String aUserId) {
this.userId = aUserId;
}
/**
* isDefault.
*
* @return a boolean.
*/
public boolean isDefault() {
return isDefault;
}
/**
* setDefault.
*
* @param aIsDefault a boolean.
*/
public void setDefault(boolean aIsDefault) {
this.isDefault = aIsDefault;
}
/**
* Getter for the field name
.
*
* @return a {@link java.lang.String} object.
*/
public String getName() {
return name != null ? name : "";
}
/**
* Setter for the field name
.
*
* @param aName a {@link java.lang.String} object.
*/
public void setName(String aName) {
this.name = aName;
}
/**
* Setter for the field description
.
*
* @param aDescription a {@link java.lang.String} object.
*/
public void setDescription(String aDescription) {
this.description = aDescription;
}
/**
* Getter for the field description
.
*
* @return a {@link java.lang.String} object.
*/
public String getDescription() {
return description != null ? description : "";
}
/**
* Setter for the field photosOrder
.
*
* @param aPhotosOrder a {@link java.util.List} object.
*/
public void setPhotosOrder(List aPhotosOrder) {
if (aPhotosOrder == null)
throw new IllegalArgumentException("Null photos argument.");
this.photosOrder = aPhotosOrder;
}
/**
* Getter for the field photosOrder
.
*
* @return a {@link java.util.List} object.
*/
public List getPhotosOrder() {
return new ArrayList(photosOrder);
}
/**
* getPhotosCount.
*
* @return a int.
*/
public int getPhotosCount() {
return photosOrder.size();
}
/**
* addPhotoToPhotoOrder.
*
* @param photoId a long.
*/
public void addPhotoToPhotoOrder(long photoId) {
photosOrder.add(photoId);
}
/**
* removePhotofromPhotoOrder.
*
* @param photoId a long.
*/
public void removePhotofromPhotoOrder(long photoId) {
List newOrder = new ArrayList();
for (Long photo : photosOrder)
if (photo != photoId)
newOrder.add(photo);
this.photosOrder = newOrder;
}
/** {@inheritDoc} */
@Override
public String toString() {
return "AlbumVO [id=" + id + ", userId=" + userId + ", isDefault=" + isDefault + ", name=" + name + ", description=" + description + ", photosOrder="
+ photosOrder + "]";
}
/** {@inheritDoc} */
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + (int) (id ^ (id >>> 32));
return result;
}
/** {@inheritDoc} */
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
AlbumVO other = (AlbumVO) obj;
if (id != other.id)
return false;
return true;
}
/** {@inheritDoc} */
@Override
public Object clone() {
try {
AlbumVO cloned = (AlbumVO) super.clone();
cloned.setPhotosOrder(getPhotosOrder());
return cloned;
} catch (CloneNotSupportedException e) {
throw new RuntimeException("AlbumVO should be cloneable!");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy