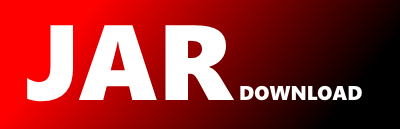
net.anotheria.anosite.photoserver.shared.vo.PhotoVO Maven / Gradle / Ivy
The newest version!
package net.anotheria.anosite.photoserver.shared.vo;
import net.anotheria.anosite.photoserver.shared.ApprovalStatus;
import java.io.File;
import java.io.Serializable;
/**
* User photo information.
*
* @author Alexandr Bolbat
* @version $Id: $Id
*/
public class PhotoVO implements Serializable, Cloneable {
/**
* Basic serialVersionUID variable.
*/
private static final long serialVersionUID = 611077253349059505L;
/**
* Unique photo id.
*/
private long id;
/**
* User id.
*/
private String userId;
/**
* Album id.
*/
private long albumId;
/**
* Flag that shows where this photo is subject for access control.
*/
private boolean restricted;
/**
* Full path to folder where photo file stored.
*/
private String fileLocation;
/**
* Path to photo in ceph storage.
*/
private String fileLocationCeph;
/**
* Extension of the photo file.
*/
private String extension;
/**
* Custom photo name from the user. Like: I'm with my friends in USA.
*/
private String name;
/**
* Custom photo description from the user. Free text with more detailed information then in name.
*/
private String description;
/**
* Modification time.
*/
private long modificationTime;
/**
* Preview settings.
*/
private PreviewSettingsVO previewSettings;
/**
* Photo approval status. WAITING_APPROVAL by default.
*/
private ApprovalStatus approvalStatus = ApprovalStatus.WAITING_APPROVAL;
/**
* Setter for the field id
.
*
* @param id a long.
*/
public void setId(long id) {
this.id = id;
}
/**
* Getter for the field id
.
*
* @return a long.
*/
public long getId() {
return id;
}
/**
* Setter for the field userId
.
*
* @param userId a {@link java.lang.String} object.
*/
public void setUserId(String userId) {
this.userId = userId;
}
/**
* Getter for the field userId
.
*
* @return a {@link java.lang.String} object.
*/
public String getUserId() {
return userId;
}
/**
* Setter for the field albumId
.
*
* @param albumId a long.
*/
public void setAlbumId(long albumId) {
this.albumId = albumId;
}
/**
* Getter for the field albumId
.
*
* @return a long.
*/
public long getAlbumId() {
return albumId;
}
/**
* isRestricted.
*
* @return a boolean.
*/
public boolean isRestricted() {
return restricted;
}
/**
* Setter for the field restricted
.
*
* @param restricted a boolean.
*/
public void setRestricted(boolean restricted) {
this.restricted = restricted;
}
/**
* Setter for the field fileLocation
.
*
* @param aFileLocation a {@link java.lang.String} object.
*/
public void setFileLocation(String aFileLocation) {
this.fileLocation = aFileLocation;
}
/**
* Getter for the field fileLocation
.
*
* @return a {@link java.lang.String} object.
*/
public String getFileLocation() {
return fileLocation != null ? fileLocation : "";
}
public String getFileLocationCeph() {
return fileLocationCeph;
}
public void setFileLocationCeph(String fileLocationCeph) {
this.fileLocationCeph = fileLocationCeph;
}
/**
* Setter for the field name
.
*
* @param aName a {@link java.lang.String} object.
*/
public void setName(String aName) {
this.name = aName;
}
/**
* Getter for the field name
.
*
* @return a {@link java.lang.String} object.
*/
public String getName() {
return name != null ? name : "";
}
/**
* Setter for the field description
.
*
* @param aDescription a {@link java.lang.String} object.
*/
public void setDescription(String aDescription) {
this.description = aDescription;
}
/**
* Getter for the field description
.
*
* @return a {@link java.lang.String} object.
*/
public String getDescription() {
return description != null ? description : "";
}
/**
* Setter for the field modificationTime
.
*
* @param aModificationTime a long.
*/
public void setModificationTime(long aModificationTime) {
this.modificationTime = aModificationTime;
}
/**
* Getter for the field modificationTime
.
*
* @return a long.
*/
public long getModificationTime() {
return modificationTime;
}
/**
* Setter for the field previewSettings
.
*
* @param aPreviewSettings a {@link net.anotheria.anosite.photoserver.shared.vo.PreviewSettingsVO} object.
*/
public void setPreviewSettings(PreviewSettingsVO aPreviewSettings) {
this.previewSettings = aPreviewSettings;
}
/**
* Getter for the field previewSettings
.
*
* @return a {@link net.anotheria.anosite.photoserver.shared.vo.PreviewSettingsVO} object.
*/
public PreviewSettingsVO getPreviewSettings() {
return previewSettings;
}
/**
* Get full photo file path.
*
* @return {@link java.lang.String} photo file name with full path
*/
public String getFilePath() {
return getFileLocation() + File.separator + getId() + getExtension();
}
/**
* Setter for the field extension
.
*
* @param aExtension a {@link java.lang.String} object.
*/
public void setExtension(String aExtension) {
this.extension = aExtension;
}
/**
* Getter for the field extension
.
*
* @return a {@link java.lang.String} object.
*/
public String getExtension() {
return extension != null ? extension : "";
}
/**
* Setter for the field approvalStatus
.
*
* @param approvalStatus a {@link net.anotheria.anosite.photoserver.shared.ApprovalStatus} object.
*/
public void setApprovalStatus(ApprovalStatus approvalStatus) {
this.approvalStatus = approvalStatus;
}
/**
* Getter for the field approvalStatus
.
*
* @return a {@link net.anotheria.anosite.photoserver.shared.ApprovalStatus} object.
*/
public ApprovalStatus getApprovalStatus() {
return approvalStatus;
}
@Override
public String toString() {
return "PhotoVO{" +
"id=" + id +
", userId='" + userId + '\'' +
", albumId=" + albumId +
", restricted=" + restricted +
", fileLocation='" + fileLocation + '\'' +
", fileLocationCeph='" + fileLocationCeph + '\'' +
", extension='" + extension + '\'' +
", name='" + name + '\'' +
", description='" + description + '\'' +
", modificationTime=" + modificationTime +
", previewSettings=" + previewSettings +
", approvalStatus=" + approvalStatus +
'}';
}
/** {@inheritDoc} */
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + (int) (id ^ (id >>> 32));
return result;
}
/** {@inheritDoc} */
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
PhotoVO other = (PhotoVO) obj;
if (id != other.id)
return false;
return true;
}
/** {@inheritDoc} */
@Override
public Object clone() {
try {
return super.clone();
} catch (CloneNotSupportedException e) {
throw new RuntimeException("PhotoVO should be cloneable!");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy