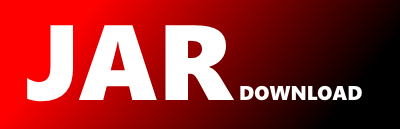
net.anotheria.anoprise.cache.Caches Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ano-prise Show documentation
Show all versions of ano-prise Show documentation
Collection of utils for different enterprise class projects. Among other stuff contains
Caches, Mocking, DualCrud, MetaFactory and SessionDistributorService. Visit https://opensource.anotheria.net for details.
package net.anotheria.anoprise.cache;
import net.anotheria.moskito.core.logging.DefaultStatsLogger;
import net.anotheria.moskito.core.logging.IntervalStatsLogger;
import net.anotheria.moskito.core.logging.SL4JLogOutput;
import net.anotheria.moskito.core.stats.DefaultIntervals;
import org.configureme.ConfigurationManager;
import org.slf4j.LoggerFactory;
/**
* Utility for cache creation.
*
* @author lrosenberg
*/
public final class Caches {
/**
* Creates a new softreference cache.
*
* @param
* @param
* @param name
* @return
*/
public static Cache createSoftReferenceCache(String name) {
return new RoundRobinSoftReferenceCache(name);
}
/**
* Creates a new soft reference cache with given name, start and max size.
*
* @param type used as key in the cache.
* @param type used as value in the cache.
* @param name name of the cache.
* @param startSize starting size of the cache.
* @param maxSize max size of the cache.
* @return
*/
public static Cache createSoftReferenceCache(String name, int startSize, int maxSize) {
return new RoundRobinSoftReferenceCache(name, startSize, maxSize);
}
/**
* Creates a new hardwired cache with given name and default size.
* @param name
* @return
*/
public static Cache createHardwiredCache(String name) {
return new RoundRobinHardwiredCache(name);
}
/**
* Creates a new hardwired cache with given name and size.
* @param name
* @param startSize
* @param maxSize
* @return
*/
public static Cache createHardwiredCache(String name, int startSize, int maxSize) {
return new RoundRobinHardwiredCache(name, startSize, maxSize);
}
public static Cache createHardwiredExpiringCache(String name, int startSize, int maxSize, int expirationTime) {
Cache> underlyingCache = createHardwiredCache(name, startSize, maxSize);
return new ExpiringCache(name, expirationTime, underlyingCache);
}
public static Cache createSoftReferenceExpiringCache(String name, int startSize, int maxSize, int expirationTime) {
Cache> underlyingCache = createSoftReferenceCache(name, startSize, maxSize);
return new ExpiringCache(name, expirationTime, underlyingCache);
}
public static Cache createConfigurableSoftReferenceCache(String name) {
CacheFactory factory = new RoundRobinSoftReferenceCacheFactory();
CacheController controller = new CacheController(name, factory);
ConfigurationManager.INSTANCE.configureAs(controller, name);
return controller;
}
public static Cache createConfigurableSoftReferenceExpiringCache(String name) {
CacheFactory factory = new RoundRobinSoftReferenceCacheFactory();
CacheController controller = new CacheController(name, factory);
ConfigurationManager.INSTANCE.configureAs(controller, name);
return controller;
}
public static Cache createConfigurableHardwiredCache(String name) {
CacheFactory factory = new RoundRobinHardwiredCacheFactory();
CacheController controller = new CacheController(name, factory);
ConfigurationManager.INSTANCE.configureAs(controller, name);
return controller;
}
public static Cache createConfigurableCache(String name) {
CacheController controller = new CacheController(name);
ConfigurationManager.INSTANCE.configureAs(controller, name);
return controller;
}
/**
* Creates a new soft reference cache with failover node support cache with given params
*
* @param name name of the cache.
* @param startSize starting size of the cache.
* @param maxSize max size of the cache.
* @param instanceAmount number of the cache instances in order
* @param currentInstanceNumber current number of cache instance
* @param modableTypeHandler instance of the ModableTypeHandler to calculate modable value, can be null if use primitive type the key in the cache.
* @param type used as key in the cache.
* @param type used as value in the cache.
* @return
*/
public static Cache createSoftReferenceFailoverSupportCache(String name, int startSize, int maxSize, int instanceAmount, int currentInstanceNumber, ModableTypeHandler modableTypeHandler) {
Cache underlyingCache = createSoftReferenceCache(name, startSize, maxSize);
return new FailoverCache(name, instanceAmount, currentInstanceNumber, modableTypeHandler, underlyingCache);
}
/**
* Creates a new soft reference expiring cache with failover node support cache with given params
*
* @param name name of the cache.
* @param startSize starting size of the cache.
* @param maxSize max size of the cache.
* @param expirationTime expiration time of the cache entry
* @param instanceAmount number of the cache instances in order
* @param currentInstanceNumber current number of cache instance
* @param modableTypeHandler instance of the ModableTypeHandler to calculate modable value, can be null if use primitive type the key in the cache.
* @param type used as key in the cache.
* @param type used as value in the cache.
* @return created cahe
*/
public static Cache createSoftReferenceExpiringFailoverSupportCache(String name, int startSize, int maxSize, int expirationTime, int instanceAmount, int currentInstanceNumber, ModableTypeHandler modableTypeHandler) {
Cache underlyingCache = createSoftReferenceExpiringCache(name, startSize, maxSize, expirationTime);
return new FailoverCache(name, instanceAmount, currentInstanceNumber, modableTypeHandler, underlyingCache);
}
/**
* Creates a new configurable soft reference cache with failover node support cache with given params
*
* @param name configurable params (json file)
* @param modableTypeHandler instance of the ModableTypeHandler to calculate modable value, can be null if use primitive type the key in the cache.
* @param ype used as key in the cache.
* @param type used as value in the cache.
* @return
*/
public static Cache createConfigurableSoftReferenceCacheFailoverSupportCache(String name, int instanceAmount, int currentInstanceNumber, ModableTypeHandler modableTypeHandler) {
CacheFactory factory = new RoundRobinSoftReferenceCacheFactory();
CacheController controller = new CacheController(name, factory, instanceAmount, currentInstanceNumber, modableTypeHandler);
ConfigurationManager.INSTANCE.configureAs(controller, name);
return controller;
}
/**
* Creates a new configurable soft reference expiring cache with failover node support cache with given params
*
* @param name configurable params (json file)
* @param modableTypeHandler instance of the ModableTypeHandler to calculate modable value, can be null if use primitive type the key in the cache.
* @param ype used as key in the cache.
* @param type used as value in the cache.
* @return
*/
public static Cache createConfigurableSoftReferenceExpiringCacheFailoverSupportCache(String name, int instanceAmount, int currentInstanceNumber, ModableTypeHandler modableTypeHandler) {
CacheFactory factory = new RoundRobinSoftReferenceCacheFactory();
CacheController controller = new CacheController(name, factory, instanceAmount, currentInstanceNumber, modableTypeHandler);
ConfigurationManager.INSTANCE.configureAs(controller, name);
return controller;
}
public static void attachCacheToMoskitoLoggers(Cache, ?> cache, String producerId, String category, String subsystem) {
CacheProducerWrapper cacheWrapper = new CacheProducerWrapper(cache, producerId, category, subsystem);
new DefaultStatsLogger(cacheWrapper, new SL4JLogOutput(LoggerFactory.getLogger("moskito.custom.default")));
new IntervalStatsLogger(cacheWrapper, DefaultIntervals.FIVE_MINUTES, new SL4JLogOutput(LoggerFactory.getLogger("moskito.custom.5m")));
new IntervalStatsLogger(cacheWrapper, DefaultIntervals.FIFTEEN_MINUTES, new SL4JLogOutput(LoggerFactory.getLogger("moskito.custom.15m")));
new IntervalStatsLogger(cacheWrapper, DefaultIntervals.ONE_HOUR, new SL4JLogOutput(LoggerFactory.getLogger("moskito.custom.1h")));
new IntervalStatsLogger(cacheWrapper, DefaultIntervals.ONE_DAY, new SL4JLogOutput(LoggerFactory.getLogger("moskito.custom.1d")));
}
/*
public static final Cache createConfigurableHardwiredExpiringCache(String name){
Cache> underlyingCache = createHardwiredCache(name, startSize, maxSize);
return new ExpiringCache(name, expirationTime, underlyingCache);
}
public static final Cache createConfigurableSoftReferenceExpiringCache(String name){
Cache> underlyingCache = createSoftReferenceCache(name, startSize, maxSize);
return new ExpiringCache(name, expirationTime, underlyingCache);
}
*/
private Caches() {
//protect from instantiation.
}
public static enum Strategy {
ROUNDROBIN,
EXPIRATION
}
public static enum Wiring {
HARDWIRED,
SOFTREFERENCE,
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy