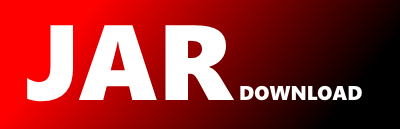
net.anotheria.anoprise.dualcrud.CrudServiceFixture Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ano-prise Show documentation
Show all versions of ano-prise Show documentation
Collection of utils for different enterprise class projects. Among other stuff contains
Caches, Mocking, DualCrud, MetaFactory and SessionDistributorService. Visit https://opensource.anotheria.net for details.
package net.anotheria.anoprise.dualcrud;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.ConcurrentHashMap;
public class CrudServiceFixture implements CrudService {
private final ConcurrentHashMap holder;
public CrudServiceFixture() {
holder = new ConcurrentHashMap();
}
@Override
public T create(T t) throws CrudServiceException {
if (exists(t))
throw new CrudServiceException("Object already exist. Owner id: " + t.getOwnerId());
return holder.put(t.getOwnerId(), t);
}
@Override
public T read(String ownerId) throws CrudServiceException, ItemNotFoundException {
if (!exist(ownerId))
throw new ItemNotFoundException(ownerId);
return holder.get(ownerId);
}
@Override
public T update(T t) throws CrudServiceException {
if (!exists(t))
throw new ItemNotFoundException(t.getOwnerId());
return holder.put(t.getOwnerId(), t);
}
@Override
public void delete(T t) throws CrudServiceException {
holder.remove(t.getOwnerId());
}
@Override
public T save(T t) throws CrudServiceException {
return holder.put(t.getOwnerId(), t);
}
@Override
public boolean exists(T t) throws CrudServiceException {
return exist(t.getOwnerId());
}
/**
* Object existence check.
*
* @param ownerId
* - owner id
* @return true
if object exist or false
*/
private boolean exist(String ownerId) {
return holder.containsKey(ownerId);
}
@Override
public List query(Query q) throws CrudServiceException {
return new ArrayList();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy