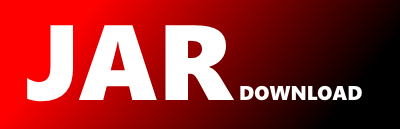
net.anotheria.anoprise.inmemorymirror.InMemoryMirrorImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ano-prise Show documentation
Show all versions of ano-prise Show documentation
Collection of utils for different enterprise class projects. Among other stuff contains
Caches, Mocking, DualCrud, MetaFactory and SessionDistributorService. Visit https://opensource.anotheria.net for details.
package net.anotheria.anoprise.inmemorymirror;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.locks.ReadWriteLock;
import java.util.concurrent.locks.ReentrantReadWriteLock;
public class InMemoryMirrorImpl> implements InMemoryMirror{
private InMemorySupport support;
private volatile Map cache = null;
private ReadWriteLock lock = new ReentrantReadWriteLock();
public InMemoryMirrorImpl(String configName, InMemorySupport aSupport) {
support = aSupport;
}
@Override
public Collection getAll() throws InMemoryMirrorException{
getCache();
try{
lock.readLock().lock();
return new ArrayList(cache.values());
}finally{
lock.readLock().unlock();
}
}
private Map getCache() throws InMemoryMirrorException{
try{
lock.readLock().lock();
if (cache!=null)
return cache;
}finally{
lock.readLock().unlock();
}
try{
lock.writeLock().lock();
if (cache!=null)
return cache;
Collection fromSupport = support.readAll();
HashMap map = new HashMap(fromSupport.size());
for (V v : fromSupport)
map.put(v.getKey(), v);
cache = map;
return cache;
}finally{
lock.writeLock().unlock();
}
}
@Override
public V get(K key) throws InMemoryMirrorException{
V ret = getCache().get(key);
if (ret==null)
throw new ElementNotFoundException(key.toString());
return ret;
}
@Override
public V remove(K key) throws InMemoryMirrorException{
return remove(key, false);
}
@Override
public V removeLocalOnly(K id) throws InMemoryMirrorException {
return remove(id, true);
}
protected V remove(K key, boolean localOnly) throws InMemoryMirrorException{
try {
lock.writeLock().lock();
if (!localOnly) {
support.remove(key);
}
return getCache().remove(key);
} finally {
lock.writeLock().unlock();
}
}
@Override
public void update(V element) throws InMemoryMirrorException{
update(element, false);
}
@Override
public void updateLocalOnly(V element) throws InMemoryMirrorException, ElementNotFoundException {
update(element, true);
}
protected void update(V element, boolean localOnly) throws InMemoryMirrorException{
try{
lock.writeLock().lock();
if(!localOnly) {
support.update(element);
}
getCache().put(element.getKey(), element);
}finally{
lock.writeLock().unlock();
}
}
@Override
public V create(V element) throws InMemoryMirrorException {
return create(element, false);
}
@Override
public V createLocalOnly(V element) throws InMemoryMirrorException {
return create(element, true);
}
protected V create(V element, boolean localOnly) throws InMemoryMirrorException {
try{
lock.writeLock().lock();
V created = element;
if(!localOnly) {
created = support.create(element);
}
getCache().put(created.getKey(), created);
return created;
}catch(Exception exception){
throw new InMemoryMirrorException("create(" + element + ")", exception);
}finally{
lock.writeLock().unlock();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy