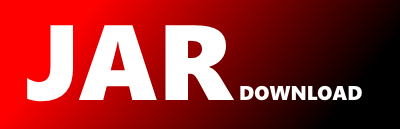
net.anotheria.anoprise.processor.QueuedMultiProcessorBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ano-prise Show documentation
Show all versions of ano-prise Show documentation
Collection of utils for different enterprise class projects. Among other stuff contains
Caches, Mocking, DualCrud, MetaFactory and SessionDistributorService. Visit https://opensource.anotheria.net for details.
package net.anotheria.anoprise.processor;
import net.anotheria.anoprise.queue.BoundedFifoQueueFactory;
import net.anotheria.anoprise.queue.EnterpriseQueueFactory;
import org.slf4j.Logger;
import java.util.List;
/**
*
* Use this class to build new QueuedMultiProcessor. If some processor
* parameters are not set during the building default values will be used.
*
* Can be build processors with two types of workers:
*
* - ElementWorker to perform work on a single element at one processing cycle
*
* - PackageWorker to perform work on a package of element at one processing cycle
*
*
* @author dmetelin
*
*/
public class QueuedMultiProcessorBuilder{
/**
* Default time to sleep.
*/
public static final long DEF_SLEEP_TIME = 50;
/**
* Default queue size.
*/
public static final int DEF_QUEUE_SIZE = 1000;
public static final Class> DEF_QUEUE_FACTORY_CLASS = BoundedFifoQueueFactory.class;
public static final int DEF_PROCESSOR_CHANNELS = 10;
private long sleepTime;
private int queueSize;
private Class> queueFactoryClass;
private int processorChannels;
private boolean attachMoskitoLoggers = false;
private String moskitoProducerId;
private String moskitoCategory;
private String moskitoSubsystem;
private Logger processingLog;
/**
* Creates new builder
*/
@SuppressWarnings("unchecked")
public QueuedMultiProcessorBuilder() {
queueSize = DEF_QUEUE_SIZE;
processorChannels = DEF_PROCESSOR_CHANNELS;
sleepTime = DEF_SLEEP_TIME;
queueFactoryClass = (Class>) DEF_QUEUE_FACTORY_CLASS;
processingLog = null;
}
/**
* Builds processor to perform work under single element at one processing
* cycle: single element is dequeued and sent to the element worker.
*
* @param
* @param name
* (identifier) for this processor
* @param worker
* for the package of elements
* @return processor
*/
public QueuedMultiProcessor build(String name, ElementWorker worker) {
return build(name, new PackageWorkerAdapter(worker));
}
/**
* Builds processor to perform work under elements package at one processing
* cycle: elements from the queue are gathered to the package and sent to
* the package worker
*
* @param
* @param name
* (identifier) for this processor
* @param worker
* for the package of elements
* @return processor
*/
public QueuedMultiProcessor build(String name, PackageWorker worker) {
try {
EnterpriseQueueFactory queueFactory = queueFactoryClass.newInstance();
QueuedMultiProcessor ret = new QueuedMultiProcessor(name, worker, queueFactory, queueSize, processorChannels, sleepTime, processingLog);
if(attachMoskitoLoggers)
new QueuedMultiProcessorProducerWrapper(ret, moskitoProducerId, moskitoCategory, moskitoSubsystem).attachToMoskitoLoggers();
return ret;
} catch (Exception e) {
throw new RuntimeException("Could not build QueuedMultiProcessor with name " + name + ": ", e);
}
}
/**
* Sets the maximal size of the processor Queue
*
* @param queueSize
* @return
*/
public QueuedMultiProcessorBuilder setQueueSize(int queueSize) {
this.queueSize = queueSize;
return this;
}
/**
* Sets factory to be used for creating Queue
*
* @param queueFactoryClass
* @return
*/
public QueuedMultiProcessorBuilder setQueueFactoryClass(Class> queueFactoryClass) {
this.queueFactoryClass = queueFactoryClass;
return this;
}
/**
* Sets number of async channels of processor in which work is performed.
*
* @param processorChannels
* @return builder
*/
public QueuedMultiProcessorBuilder setProcessorChannels(int processorChannels) {
this.processorChannels = processorChannels;
return this;
}
public QueuedMultiProcessorBuilder setProcessingLog(Logger processingLog) {
this.processingLog = processingLog;
return this;
}
/**
* Sets sleep time to wait a new element arriving if queue is empty.
*
* @param sleepTime
* @return builder
*/
public QueuedMultiProcessorBuilder setSleepTime(long sleepTime) {
this.sleepTime = sleepTime;
return this;
}
public QueuedMultiProcessorBuilder attachMoskitoLoggers(String producerId, String category, String subsystem){
attachMoskitoLoggers = true;
moskitoProducerId = producerId;
moskitoCategory = category;
moskitoSubsystem = subsystem;
return this;
}
/**
* Adapts ElementWorker to PackageWorker for single element processing.
*
* @author dmetelin
*
* @param
*/
private class PackageWorkerAdapter implements PackageWorker {
private ElementWorker elementWorker;
public PackageWorkerAdapter(ElementWorker anElementWorker) {
elementWorker = anElementWorker;
}
@Override
public void doWork(List workingPackage) throws Exception {
elementWorker.doWork(workingPackage.get(0));
}
@Override
public int packageCapacity() {
return 1;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy