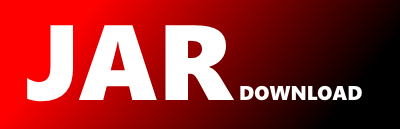
net.anotheria.anoprise.sessiondistributor.DistributedSessionVO Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ano-prise Show documentation
Show all versions of ano-prise Show documentation
Collection of utils for different enterprise class projects. Among other stuff contains
Caches, Mocking, DualCrud, MetaFactory and SessionDistributorService. Visit https://opensource.anotheria.net for details.
package net.anotheria.anoprise.sessiondistributor;
import net.anotheria.util.StringUtils;
import java.io.Serializable;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* DistributedSession value object used in SessionDistributorService.
*
* @author lrosenberg
* @version 1.0, 2010/01/03
*/
public class DistributedSessionVO implements Serializable {
/**
* Basic serialVersionUID variable.
*/
private static final long serialVersionUID = -2764699143075615769L;
/**
* SessionDistributorServiceConfig instance.
*/
private static SessionDistributorServiceConfig serviceConfig;
/**
* Static initialization block.
*/
static {
serviceConfig = SessionDistributorServiceConfig.getInstance();
}
/**
* DistributedSessionVO 'name'.
* Actually id of associated session.
*/
private String name;
/**
* DistributedSessionVO 'lastChangeTime'.
* Last session change time.
* First will be initialized in constructor.
*/
private long lastChangeTime;
/**
* DistributedSessionVO 'userId'.
*/
private String userId;
/**
* DistributedSessionVO 'editorId'.
*/
private String editorId;
/**
* DistributedSessionVO 'distributedAttributes'.
*/
private Map distributedAttributes;
/**
* Default constructor.
*
* @param aName - holder name
*/
public DistributedSessionVO(String aName) {
name = aName;
distributedAttributes = new ConcurrentHashMap();
lastChangeTime = System.currentTimeMillis();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public long getLastChangeTime() {
return lastChangeTime;
}
public void setLastChangeTime(long lastChangeTime) {
this.lastChangeTime = lastChangeTime;
}
public String getUserId() {
return userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
public String getEditorId() {
return editorId;
}
public void setEditorId(String editorId) {
this.editorId = editorId;
}
public Map getDistributedAttributes() {
return distributedAttributes;
}
public void setDistributedAttributes(Map aDistributedAttributes) {
if (aDistributedAttributes == null)
throw new IllegalArgumentException("distributedAttributes can't be null");
this.distributedAttributes = aDistributedAttributes;
}
public void addDistributedAttribute(DistributedSessionAttribute attribute) {
if (attribute == null)
throw new IllegalArgumentException("Distributed attribute can't be null");
distributedAttributes.put(attribute.getName(), attribute);
}
public void removeDistributedAttribute(String attributeName) {
if (StringUtils.isEmpty(attributeName))
throw new IllegalArgumentException("Distributed attribute name is illegal");
if (distributedAttributes.containsKey(attributeName))
distributedAttributes.remove(attributeName);
}
@Override
public String toString() {
return "DistributedSessionVO{" +
"distributedAttributes size=" + distributedAttributes.size() +
", name='" + name + '\'' +
", lastChangeTime=" + ((System.currentTimeMillis() - lastChangeTime) / 1000) + " seconds ago" +
", userId='" + userId + '\'' +
", editorId='" + editorId + '\'' +
'}';
}
/**
* Return true if session is expired. False otherwise.
*
* @return boolean value
*/
public boolean isExpired() {
return System.currentTimeMillis() - getLastChangeTime() > serviceConfig.getDistributedSessionMaxAge();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy