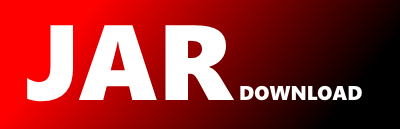
net.anotheria.anosite.gen.ascustomaction.service.ASCustomActionServiceImpl Maven / Gradle / Ivy
The newest version!
/**
********************************************************************************
*** ASCustomActionServiceImpl.java ***
*** The implementation of the IASCustomActionService. ***
*** generated by AnoSiteGenerator (ASG), Version: 4.2.2 ***
*** Copyright (C) 2005 - 2025 Anotheria.net, www.anotheria.net ***
*** All Rights Reserved. ***
********************************************************************************
*** Don't edit this code, if you aren't sure ***
*** that you do exactly know what you are doing! ***
*** It's better to invest time in the generator, as into the generated code. ***
********************************************************************************
*/
package net.anotheria.anosite.gen.ascustomaction.service;
import java.nio.charset.Charset;
import java.util.List;
import java.util.ArrayList;
import java.util.Set;
import net.anotheria.anodoc.data.Module;
import net.anotheria.anodoc.data.Property;
import net.anotheria.anodoc.data.NoSuchPropertyException;
import net.anotheria.util.sorter.SortType;
import net.anotheria.util.sorter.StaticQuickSorter;
import net.anotheria.util.slicer.Segment;
import net.anotheria.util.slicer.Slicer;
import net.anotheria.anosite.gen.ascustomaction.data.ModuleASCustomAction;
import net.anotheria.anosite.gen.shared.service.BasicCMSService;
import net.anotheria.anodoc.query2.DocumentQuery;
import net.anotheria.anodoc.query2.QueryResult;
import net.anotheria.anodoc.query2.QueryResultEntry;
import net.anotheria.anodoc.query2.QueryProperty;
import net.anotheria.util.StringUtils;
import net.anotheria.util.xml.XMLNode;
import net.anotheria.util.xml.XMLAttribute;
import net.anotheria.asg.util.listener.IModuleListener;
import org.codehaus.jettison.json.JSONObject;
import org.codehaus.jettison.json.JSONArray;
import org.codehaus.jettison.json.JSONException;
import com.fasterxml.jackson.core.JsonProcessingException;
import java.io.IOException;
import net.anotheria.anodoc.util.mapper.ObjectMapperUtil;
import net.anotheria.anosite.gen.shared.util.DocumentName;
import net.anotheria.anosite.gen.ascustomaction.data.CustomActionDef;
import net.anotheria.anosite.gen.ascustomaction.data.CustomActionDefXMLHelper;
import net.anotheria.anosite.gen.ascustomaction.data.CustomActionDefDocument;
import net.anotheria.anosite.gen.anoaccessconfiguration.service.AnoAccessConfigurationServiceException;
import net.anotheria.anosite.gen.ascustomaction.data.ActionMappingDef;
import net.anotheria.anosite.gen.ascustomaction.data.ActionMappingDefXMLHelper;
import net.anotheria.anosite.gen.ascustomaction.data.ActionMappingDefDocument;
import net.anotheria.anosite.gen.aswebdata.service.ASWebDataServiceException;
import net.anotheria.anosite.gen.asresourcedata.service.ASResourceDataServiceException;
import net.anotheria.anosite.gen.asaction.service.ASActionServiceException;
public class ASCustomActionServiceImpl extends BasicCMSService implements IASCustomActionService, IModuleListener{
// Generated by: class net.anotheria.asg.generator.model.docs.CMSBasedServiceGenerator.generateImplementation
private static ASCustomActionServiceImpl instance;
private ASCustomActionServiceImpl(){
addServiceListener(new net.anotheria.anosite.cms.listener.CRUDLogListener());
addModuleListener(ModuleASCustomAction.MODULE_ID, this);
}
static final ASCustomActionServiceImpl getInstance(){
if (instance==null){
instance = new ASCustomActionServiceImpl();
}
return instance;
}
private ModuleASCustomAction _getModuleASCustomAction(){
return (ModuleASCustomAction) getModule(ModuleASCustomAction.MODULE_ID);
}
@Override
public void moduleLoaded(Module module){
firePersistenceChangedEvent();
}
@Override
public List getCustomActionDefs(){
List customactiondefs = new ArrayList<>();
customactiondefs.addAll(_getModuleASCustomAction().getCustomActionDefs());
return customactiondefs;
}
@Override
public List getCustomActionDefs(SortType sortType){
return StaticQuickSorter.sort(getCustomActionDefs(), sortType);
}
/**
* Returns the CustomActionDef objects with the specified ids.
*/
public List getCustomActionDefs(List ids){
if (ids==null || ids.size()==0)
return new ArrayList<>(0);
List all = getCustomActionDefs();
List ret = new ArrayList<>();
for (CustomActionDef customactiondef : all){
if(ids.contains(customactiondef.getId())){
ret.add(customactiondef);
}
}
return ret;
}
/**
* Returns the CustomActionDef objects with the specified ids, sorted by given sorttype.
*/
public List getCustomActionDefs(List ids, SortType sortType){
return StaticQuickSorter.sort(getCustomActionDefs(ids), sortType);
}
@Override
public void deleteCustomActionDef(CustomActionDef customactiondef){
deleteCustomActionDef(customactiondef.getId());
if (hasServiceListeners()){
fireObjectDeletedEvent(customactiondef);
}
}
@Override
public void deleteCustomActionDef(String id){
ModuleASCustomAction module = _getModuleASCustomAction();
CustomActionDef varValue = hasServiceListeners()?module.getCustomActionDef(id):null;
module.deleteCustomActionDef(id);
updateModule(module);
if(varValue!=null){
fireObjectDeletedEvent(varValue);
}
}
@Override
public void deleteCustomActionDefs(List list){
ModuleASCustomAction module = _getModuleASCustomAction();
for (CustomActionDef customactiondef : list){
module.deleteCustomActionDef(customactiondef.getId());
}
updateModule(module);
if (hasServiceListeners()){
for (int t=0; t importCustomActionDefs(List list){
ModuleASCustomAction module = _getModuleASCustomAction();
List ret = new ArrayList<>();
for (CustomActionDef customactiondef : list){
CustomActionDef imported = module.importCustomActionDef((CustomActionDefDocument)customactiondef);
ret.add(imported);
}
updateModule(module);
if (hasServiceListeners()){
for (CustomActionDef customactiondef : ret)
fireObjectImportedEvent(customactiondef);
}
return ret;
}
@Override
public CustomActionDef createCustomActionDef(CustomActionDef customactiondef){
ModuleASCustomAction module = _getModuleASCustomAction();
module.createCustomActionDef((CustomActionDefDocument)customactiondef);
updateModule(module);
fireObjectCreatedEvent(customactiondef);
return customactiondef;
}
@Override
/**
* Creates multiple new CustomActionDef objects.
* Returns the created versions.
*/
public List createCustomActionDefs(List list){
ModuleASCustomAction module = _getModuleASCustomAction();
List ret = new ArrayList<>();
for (CustomActionDef customactiondef : list){
CustomActionDef created = module.createCustomActionDef((CustomActionDefDocument)customactiondef);
ret.add(created);
}
updateModule(module);
if (hasServiceListeners()){
for (CustomActionDef customactiondef : ret)
fireObjectCreatedEvent(customactiondef);
}
return ret;
}
@Override
public CustomActionDef updateCustomActionDef(CustomActionDef customactiondef){
CustomActionDef oldVersion = null;
ModuleASCustomAction module = _getModuleASCustomAction();
if (hasServiceListeners())
oldVersion = module.getCustomActionDef(customactiondef.getId());
module.updateCustomActionDef((CustomActionDefDocument)customactiondef);
updateModule(module);
if (oldVersion != null){
fireObjectUpdatedEvent(oldVersion, customactiondef);
}
return customactiondef;
}
@Override
public List updateCustomActionDefs(List list){
List oldList = null;
if (hasServiceListeners())
oldList = new ArrayList<>(list.size());
ModuleASCustomAction module = _getModuleASCustomAction();
for (CustomActionDef customactiondef : list){
if (oldList!=null)
oldList.add(module.getCustomActionDef(customactiondef.getId()));
module.updateCustomActionDef((CustomActionDefDocument)customactiondef);
}
updateModule(module);
if (oldList!=null){
for (int t=0; t getCustomActionDefsByProperty(String propertyName, Object value){
List allCustomActionDefs = getCustomActionDefs();
List ret = new ArrayList<>();
for (int i=0; i getCustomActionDefsByProperty(String propertyName, Object value, SortType sortType){
return StaticQuickSorter.sort(getCustomActionDefsByProperty(propertyName, value), sortType);
}
/**
* Executes a query on CustomActionDefs
*/
public QueryResult executeQueryOnCustomActionDefs(DocumentQuery query){
List allCustomActionDefs = getCustomActionDefs();
QueryResult result = new QueryResult();
for (int i=0; i partialResult = query.match(allCustomActionDefs.get(i));
result.add(partialResult);
}
return result;
}
/**
* Returns all CustomActionDef objects, where property matches.
*/
public List getCustomActionDefsByProperty(QueryProperty... property){
//first the slow version, the fast version is a todo.
List ret = new ArrayList<>();
List src = getCustomActionDefs();
for ( CustomActionDef customactiondef : src){
boolean mayPass = true;
for (QueryProperty qp : property){
mayPass = mayPass && qp.doesMatch(customactiondef.getPropertyValue(qp.getName()));
}
if (mayPass)
ret.add(customactiondef);
}
return ret;
}
/**
* Returns all CustomActionDef objects, where property matches, sorted
*/
public List getCustomActionDefsByProperty(SortType sortType, QueryProperty... property){
return StaticQuickSorter.sort(getCustomActionDefsByProperty(property), sortType);
}
/**
* Returns CustomActionDef objects count.
*/
public int getCustomActionDefsCount() {
return _getModuleASCustomAction().getCustomActionDefs().size();
}
/**
* Returns CustomActionDef objects segment.
*/
public List getCustomActionDefs(Segment aSegment) {
return Slicer.slice(aSegment, getCustomActionDefs()).getSliceData();
}
/**
* Returns CustomActionDef objects segment, where property matched.
*/
public List getCustomActionDefsByProperty(Segment aSegment, QueryProperty... property) {
int pLimit = aSegment.getElementsPerSlice();
int pOffset = aSegment.getSliceNumber() * aSegment.getElementsPerSlice() - aSegment.getElementsPerSlice();
List ret = new ArrayList<>();
List src = getCustomActionDefs();
for (CustomActionDef customactiondef : src) {
boolean mayPass = true;
for (QueryProperty qp : property) {
mayPass = mayPass && qp.doesMatch(customactiondef.getPropertyValue(qp.getName()));
}
if (mayPass)
ret.add(customactiondef);
if (ret.size() > pOffset + pLimit)
break;
}
return Slicer.slice(aSegment, ret).getSliceData();
}
/**
* Returns CustomActionDef objects segment, where property matched, sorted.
*/
public List getCustomActionDefsByProperty(Segment aSegment, SortType aSortType, QueryProperty... aProperty){
return StaticQuickSorter.sort(getCustomActionDefsByProperty(aSegment, aProperty), aSortType);
}
@Override
public void fetchCustomActionDef(final String id, Set addedDocuments, JSONArray data) throws ASCustomActionServiceException {
if (id.isEmpty() || addedDocuments.contains("CustomActionDef" + id))
return;
try {
final CustomActionDefDocument customactiondef = _getModuleASCustomAction().getCustomActionDef(id);
addedDocuments.add("CustomActionDef" + id);
if (!StringUtils.isEmpty(customactiondef.getAccessOperation()))
getAnoAccessConfigurationService().fetchAccessOperation(customactiondef.getAccessOperation(), addedDocuments, data);
JSONObject dataObject = new JSONObject();
String jsonObject = ObjectMapperUtil.getMapperInstance().writeValueAsString(customactiondef);
dataObject.put("object", jsonObject);
dataObject.put("service", "ASCustomAction");
dataObject.put("document", "ASCustomAction_CustomActionDef");
data.put(dataObject);
}catch(AnoAccessConfigurationServiceException e){
throw new ASCustomActionServiceException("Problem with getting document from AnoAccessConfiguration" + e.getMessage());
}catch(IOException e){
throw new ASCustomActionServiceException ("Problem with fetching data for this CustomActionDef instance object:" + e.getMessage());
}catch(JSONException e){
throw new ASCustomActionServiceException ("Problem with fetching data for this CustomActionDef instance in json :" + e.getMessage());
}
}
private void saveTransferredCustomActionDef(final JSONObject data) throws ASCustomActionServiceException {
try {
String objectData = data.getString("object");
CustomActionDef customactiondef = ObjectMapperUtil.getMapperInstance().readValue(objectData.getBytes(Charset.forName("UTF-8")), CustomActionDefDocument.class);
try {
updateCustomActionDef(customactiondef);
}catch(Exception e){
importCustomActionDef(customactiondef);
}
}catch(JSONException e){
throw new ASCustomActionServiceException("Problem with getting data from json CustomActionDef instance :" + e.getMessage());
}catch(IOException e){
throw new ASCustomActionServiceException("Problem with parsing data for this CustomActionDef instance :" + e.getMessage());
}
}
@Override
public List getActionMappingDefs(){
List actionmappingdefs = new ArrayList<>();
actionmappingdefs.addAll(_getModuleASCustomAction().getActionMappingDefs());
return actionmappingdefs;
}
@Override
public List getActionMappingDefs(SortType sortType){
return StaticQuickSorter.sort(getActionMappingDefs(), sortType);
}
/**
* Returns the ActionMappingDef objects with the specified ids.
*/
public List getActionMappingDefs(List ids){
if (ids==null || ids.size()==0)
return new ArrayList<>(0);
List all = getActionMappingDefs();
List ret = new ArrayList<>();
for (ActionMappingDef actionmappingdef : all){
if(ids.contains(actionmappingdef.getId())){
ret.add(actionmappingdef);
}
}
return ret;
}
/**
* Returns the ActionMappingDef objects with the specified ids, sorted by given sorttype.
*/
public List getActionMappingDefs(List ids, SortType sortType){
return StaticQuickSorter.sort(getActionMappingDefs(ids), sortType);
}
@Override
public void deleteActionMappingDef(ActionMappingDef actionmappingdef){
deleteActionMappingDef(actionmappingdef.getId());
if (hasServiceListeners()){
fireObjectDeletedEvent(actionmappingdef);
}
}
@Override
public void deleteActionMappingDef(String id){
ModuleASCustomAction module = _getModuleASCustomAction();
ActionMappingDef varValue = hasServiceListeners()?module.getActionMappingDef(id):null;
module.deleteActionMappingDef(id);
updateModule(module);
if(varValue!=null){
fireObjectDeletedEvent(varValue);
}
}
@Override
public void deleteActionMappingDefs(List list){
ModuleASCustomAction module = _getModuleASCustomAction();
for (ActionMappingDef actionmappingdef : list){
module.deleteActionMappingDef(actionmappingdef.getId());
}
updateModule(module);
if (hasServiceListeners()){
for (int t=0; t importActionMappingDefs(List list){
ModuleASCustomAction module = _getModuleASCustomAction();
List ret = new ArrayList<>();
for (ActionMappingDef actionmappingdef : list){
ActionMappingDef imported = module.importActionMappingDef((ActionMappingDefDocument)actionmappingdef);
ret.add(imported);
}
updateModule(module);
if (hasServiceListeners()){
for (ActionMappingDef actionmappingdef : ret)
fireObjectImportedEvent(actionmappingdef);
}
return ret;
}
@Override
public ActionMappingDef createActionMappingDef(ActionMappingDef actionmappingdef){
ModuleASCustomAction module = _getModuleASCustomAction();
module.createActionMappingDef((ActionMappingDefDocument)actionmappingdef);
updateModule(module);
fireObjectCreatedEvent(actionmappingdef);
return actionmappingdef;
}
@Override
/**
* Creates multiple new ActionMappingDef objects.
* Returns the created versions.
*/
public List createActionMappingDefs(List list){
ModuleASCustomAction module = _getModuleASCustomAction();
List ret = new ArrayList<>();
for (ActionMappingDef actionmappingdef : list){
ActionMappingDef created = module.createActionMappingDef((ActionMappingDefDocument)actionmappingdef);
ret.add(created);
}
updateModule(module);
if (hasServiceListeners()){
for (ActionMappingDef actionmappingdef : ret)
fireObjectCreatedEvent(actionmappingdef);
}
return ret;
}
@Override
public ActionMappingDef updateActionMappingDef(ActionMappingDef actionmappingdef){
ActionMappingDef oldVersion = null;
ModuleASCustomAction module = _getModuleASCustomAction();
if (hasServiceListeners())
oldVersion = module.getActionMappingDef(actionmappingdef.getId());
module.updateActionMappingDef((ActionMappingDefDocument)actionmappingdef);
updateModule(module);
if (oldVersion != null){
fireObjectUpdatedEvent(oldVersion, actionmappingdef);
}
return actionmappingdef;
}
@Override
public List updateActionMappingDefs(List list){
List oldList = null;
if (hasServiceListeners())
oldList = new ArrayList<>(list.size());
ModuleASCustomAction module = _getModuleASCustomAction();
for (ActionMappingDef actionmappingdef : list){
if (oldList!=null)
oldList.add(module.getActionMappingDef(actionmappingdef.getId()));
module.updateActionMappingDef((ActionMappingDefDocument)actionmappingdef);
}
updateModule(module);
if (oldList!=null){
for (int t=0; t getActionMappingDefsByProperty(String propertyName, Object value){
List allActionMappingDefs = getActionMappingDefs();
List ret = new ArrayList<>();
for (int i=0; i getActionMappingDefsByProperty(String propertyName, Object value, SortType sortType){
return StaticQuickSorter.sort(getActionMappingDefsByProperty(propertyName, value), sortType);
}
/**
* Executes a query on ActionMappingDefs
*/
public QueryResult executeQueryOnActionMappingDefs(DocumentQuery query){
List allActionMappingDefs = getActionMappingDefs();
QueryResult result = new QueryResult();
for (int i=0; i partialResult = query.match(allActionMappingDefs.get(i));
result.add(partialResult);
}
return result;
}
/**
* Returns all ActionMappingDef objects, where property matches.
*/
public List getActionMappingDefsByProperty(QueryProperty... property){
//first the slow version, the fast version is a todo.
List ret = new ArrayList<>();
List src = getActionMappingDefs();
for ( ActionMappingDef actionmappingdef : src){
boolean mayPass = true;
for (QueryProperty qp : property){
mayPass = mayPass && qp.doesMatch(actionmappingdef.getPropertyValue(qp.getName()));
}
if (mayPass)
ret.add(actionmappingdef);
}
return ret;
}
/**
* Returns all ActionMappingDef objects, where property matches, sorted
*/
public List getActionMappingDefsByProperty(SortType sortType, QueryProperty... property){
return StaticQuickSorter.sort(getActionMappingDefsByProperty(property), sortType);
}
/**
* Returns ActionMappingDef objects count.
*/
public int getActionMappingDefsCount() {
return _getModuleASCustomAction().getActionMappingDefs().size();
}
/**
* Returns ActionMappingDef objects segment.
*/
public List getActionMappingDefs(Segment aSegment) {
return Slicer.slice(aSegment, getActionMappingDefs()).getSliceData();
}
/**
* Returns ActionMappingDef objects segment, where property matched.
*/
public List getActionMappingDefsByProperty(Segment aSegment, QueryProperty... property) {
int pLimit = aSegment.getElementsPerSlice();
int pOffset = aSegment.getSliceNumber() * aSegment.getElementsPerSlice() - aSegment.getElementsPerSlice();
List ret = new ArrayList<>();
List src = getActionMappingDefs();
for (ActionMappingDef actionmappingdef : src) {
boolean mayPass = true;
for (QueryProperty qp : property) {
mayPass = mayPass && qp.doesMatch(actionmappingdef.getPropertyValue(qp.getName()));
}
if (mayPass)
ret.add(actionmappingdef);
if (ret.size() > pOffset + pLimit)
break;
}
return Slicer.slice(aSegment, ret).getSliceData();
}
/**
* Returns ActionMappingDef objects segment, where property matched, sorted.
*/
public List getActionMappingDefsByProperty(Segment aSegment, SortType aSortType, QueryProperty... aProperty){
return StaticQuickSorter.sort(getActionMappingDefsByProperty(aSegment, aProperty), aSortType);
}
@Override
public void fetchActionMappingDef(final String id, Set addedDocuments, JSONArray data) throws ASCustomActionServiceException {
if (id.isEmpty() || addedDocuments.contains("ActionMappingDef" + id))
return;
try {
final ActionMappingDefDocument actionmappingdef = _getModuleASCustomAction().getActionMappingDef(id);
addedDocuments.add("ActionMappingDef" + id);
if (!StringUtils.isEmpty(actionmappingdef.getAction()))
getASActionService().fetchActionDef(actionmappingdef.getAction(), addedDocuments, data);
if (!StringUtils.isEmpty(actionmappingdef.getPage()))
getASWebDataService().fetchPagex(actionmappingdef.getPage(), addedDocuments, data);
if (!actionmappingdef.getLocalizationBundles().isEmpty()) {
for (String aLocalizationBundlesId: actionmappingdef.getLocalizationBundles()) {
getASResourceDataService().fetchLocalizationBundle(aLocalizationBundlesId, addedDocuments, data);
}
}
JSONObject dataObject = new JSONObject();
String jsonObject = ObjectMapperUtil.getMapperInstance().writeValueAsString(actionmappingdef);
dataObject.put("object", jsonObject);
dataObject.put("service", "ASCustomAction");
dataObject.put("document", "ASCustomAction_ActionMappingDef");
data.put(dataObject);
}catch(ASWebDataServiceException e){
throw new ASCustomActionServiceException("Problem with getting document from ASWebData" + e.getMessage());
}catch(ASResourceDataServiceException e){
throw new ASCustomActionServiceException("Problem with getting document from ASResourceData" + e.getMessage());
}catch(ASActionServiceException e){
throw new ASCustomActionServiceException("Problem with getting document from ASAction" + e.getMessage());
}catch(IOException e){
throw new ASCustomActionServiceException ("Problem with fetching data for this ActionMappingDef instance object:" + e.getMessage());
}catch(JSONException e){
throw new ASCustomActionServiceException ("Problem with fetching data for this ActionMappingDef instance in json :" + e.getMessage());
}
}
private void saveTransferredActionMappingDef(final JSONObject data) throws ASCustomActionServiceException {
try {
String objectData = data.getString("object");
ActionMappingDef actionmappingdef = ObjectMapperUtil.getMapperInstance().readValue(objectData.getBytes(Charset.forName("UTF-8")), ActionMappingDefDocument.class);
try {
updateActionMappingDef(actionmappingdef);
}catch(Exception e){
importActionMappingDef(actionmappingdef);
}
}catch(JSONException e){
throw new ASCustomActionServiceException("Problem with getting data from json ActionMappingDef instance :" + e.getMessage());
}catch(IOException e){
throw new ASCustomActionServiceException("Problem with parsing data for this ActionMappingDef instance :" + e.getMessage());
}
}
public void executeParsingForDocument (final DocumentName documentName, final JSONObject data) throws ASCustomActionServiceException {
switch(documentName) {
case DOCUMENT_ASCUSTOMACTION_CUSTOMACTIONDEF:
saveTransferredCustomActionDef(data);
break;
case DOCUMENT_ASCUSTOMACTION_ACTIONMAPPINGDEF:
saveTransferredActionMappingDef(data);
break;
default:
log.info("There is no correct document: " + documentName + "in this service");
throw new ASCustomActionServiceException("No such document");
}
}
/**
* Executes a query on all data objects (documents, vo) which are part of this module and managed by this service
*/
public QueryResult executeQueryOnAllObjects(DocumentQuery query){
QueryResult ret = new QueryResult();
ret.add(executeQueryOnCustomActionDefs(query).getEntries());
ret.add(executeQueryOnActionMappingDefs(query).getEntries());
return ret;
} //executeQueryOnAllObjects
public XMLNode exportCustomActionDefsToXML(){
XMLNode ret = new XMLNode("CustomActionDefs");
List list = getCustomActionDefs();
ret.addAttribute(new XMLAttribute("count", list.size()));
for (CustomActionDef object : list)
ret.addChildNode(CustomActionDefXMLHelper.toXML(object));
return ret;
}
public XMLNode exportCustomActionDefsToXML(List list){
XMLNode ret = new XMLNode("CustomActionDefs");
ret.addAttribute(new XMLAttribute("count", list.size()));
for (CustomActionDef object : list)
ret.addChildNode(CustomActionDefXMLHelper.toXML(object));
return ret;
}
public XMLNode exportActionMappingDefsToXML(){
XMLNode ret = new XMLNode("ActionMappingDefs");
List list = getActionMappingDefs();
ret.addAttribute(new XMLAttribute("count", list.size()));
for (ActionMappingDef object : list)
ret.addChildNode(ActionMappingDefXMLHelper.toXML(object));
return ret;
}
public XMLNode exportActionMappingDefsToXML(List list){
XMLNode ret = new XMLNode("ActionMappingDefs");
ret.addAttribute(new XMLAttribute("count", list.size()));
for (ActionMappingDef object : list)
ret.addChildNode(ActionMappingDefXMLHelper.toXML(object));
return ret;
}
public XMLNode exportToXML(){
XMLNode ret = new XMLNode("ASCustomAction");
ret.addChildNode(exportCustomActionDefsToXML());
ret.addChildNode(exportActionMappingDefsToXML());
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy