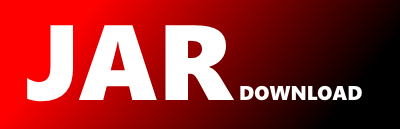
net.anotheria.anosite.gen.asfederateddata.service.ASFederatedDataServiceImpl Maven / Gradle / Ivy
The newest version!
/**
*************************************************************************************************************************************************
*** ASFederatedDataServiceImpl.java ***
*** The implementation of the IASFederatedDataServiceas a federated service layer: Federated modules: ASGenericData as G, ASCustomData as C, ***
*** generated by AnoSiteGenerator (ASG), Version: 4.2.2 ***
*** Copyright (C) 2005 - 2025 Anotheria.net, www.anotheria.net ***
*** All Rights Reserved. ***
*************************************************************************************************************************************************
*** Don't edit this code, if you aren't sure ***
*** that you do exactly know what you are doing! ***
*** It's better to invest time in the generator, as into the generated code. ***
*************************************************************************************************************************************************
*/
package net.anotheria.anosite.gen.asfederateddata.service;
import java.nio.charset.Charset;
import java.util.List;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Set;
import java.io.IOException;
import net.anotheria.util.sorter.SortType;
import net.anotheria.util.sorter.StaticQuickSorter;
import net.anotheria.util.StringUtils;
import net.anotheria.util.slicer.Segment;
import org.codehaus.jettison.json.JSONObject;
import org.codehaus.jettison.json.JSONArray;
import org.codehaus.jettison.json.JSONException;
import net.anotheria.anosite.gen.shared.util.DocumentName;
import net.anotheria.anosite.gen.shared.service.BasicService;
import net.anotheria.anosite.gen.asgenericdata.service.IASGenericDataService;
import net.anotheria.anosite.gen.asgenericdata.service.ASGenericDataServiceException;
import net.anotheria.anosite.gen.asgenericdata.service.ASGenericDataServiceFactory;
import net.anotheria.anosite.gen.ascustomdata.service.IASCustomDataService;
import net.anotheria.anosite.gen.ascustomdata.service.ASCustomDataServiceException;
import net.anotheria.anosite.gen.ascustomdata.service.ASCustomDataServiceFactory;
import net.anotheria.anosite.gen.asfederateddata.data.BoxType;
import net.anotheria.anosite.gen.asfederateddata.data.BoxTypeVO;
import net.anotheria.anosite.gen.asfederateddata.data.BoxTypeFactory;
import net.anotheria.anosite.gen.asgenericdata.data.GenericBoxType;
import net.anotheria.anosite.gen.ascustomdata.data.CustomBoxType;
import net.anotheria.anosite.gen.asfederateddata.data.BoxHandlerDef;
import net.anotheria.anosite.gen.asfederateddata.data.BoxHandlerDefVO;
import net.anotheria.anosite.gen.asfederateddata.data.BoxHandlerDefFactory;
import net.anotheria.anosite.gen.asgenericdata.data.GenericBoxHandlerDef;
import net.anotheria.anosite.gen.ascustomdata.data.CustomBoxHandlerDef;
import net.anotheria.anosite.gen.asfederateddata.data.GuardDef;
import net.anotheria.anosite.gen.asfederateddata.data.GuardDefVO;
import net.anotheria.anosite.gen.asfederateddata.data.GuardDefFactory;
import net.anotheria.anosite.gen.asgenericdata.data.GenericGuardDef;
import net.anotheria.anosite.gen.ascustomdata.data.CustomGuardDef;
import net.anotheria.anodoc.query2.DocumentQuery;
import net.anotheria.anodoc.query2.QueryResult;
import net.anotheria.anodoc.query2.QueryResultEntry;
import net.anotheria.anodoc.query2.QueryProperty;
import net.anotheria.util.xml.XMLNode;
public class ASFederatedDataServiceImpl extends BasicService implements IASFederatedDataService{
// Generated by: class net.anotheria.asg.generator.model.federation.FederationServiceGenerator.generateImplementation
private static ASFederatedDataServiceImpl instance;
// Federated services:
public static final char ID_DELIMITER = '-';
IASGenericDataService federatedG;
public static final String ID_PREFIX_G = "G"+ID_DELIMITER;
IASCustomDataService federatedC;
public static final String ID_PREFIX_C = "C"+ID_DELIMITER;
private HashMap federatedServiceMap;
private ASFederatedDataServiceImpl(){
addServiceListener(new net.anotheria.anosite.cms.listener.CRUDLogListener());
federatedServiceMap = new HashMap(2);
federatedG = ASGenericDataServiceFactory.createASGenericDataService();
federatedServiceMap.put("G", federatedG);
federatedC = ASCustomDataServiceFactory.createASCustomDataService();
federatedServiceMap.put("C", federatedC);
}
static final ASFederatedDataServiceImpl getInstance(){
if (instance==null){
instance = new ASFederatedDataServiceImpl();
}
return instance;
}
private BoxType copy(GenericBoxType d){
BoxType ret = BoxTypeFactory.createBoxType(ID_PREFIX_G+d.getId());
ret.setName(d.getName());
ret.setRendererpage(d.getRendererpage());
((BoxTypeVO)ret).setLastUpdateTimestamp(d.getLastUpdateTimestamp());
return ret;
}
private List copyBoxTypeListG(List list){
List ret = new ArrayList(list.size());
for (GenericBoxType d : list)
ret.add(copy(d));
return ret;
}
private BoxType copy(CustomBoxType d){
BoxType ret = BoxTypeFactory.createBoxType(ID_PREFIX_C+d.getId());
ret.setName(d.getName());
ret.setRendererpage(d.getRendererpage());
((BoxTypeVO)ret).setLastUpdateTimestamp(d.getLastUpdateTimestamp());
return ret;
}
private List copyBoxTypeListC(List list){
List ret = new ArrayList(list.size());
for (CustomBoxType d : list)
ret.add(copy(d));
return ret;
}
public List getBoxTypes() throws ASFederatedDataServiceException {
List boxtypes = new ArrayList();
try{
List sourceG = federatedG.getGenericBoxTypes();
boxtypes.addAll(copyBoxTypeListG(sourceG));
}catch(ASGenericDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
try{
List sourceC = federatedC.getCustomBoxTypes();
boxtypes.addAll(copyBoxTypeListC(sourceC));
}catch(ASCustomDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
return boxtypes;
}
public List getBoxTypes(SortType sortType) throws ASFederatedDataServiceException {
return StaticQuickSorter.sort(getBoxTypes(), sortType);
}
public void deleteBoxType(BoxType boxtype) throws ASFederatedDataServiceException {
deleteBoxType(boxtype.getId());
}
public void deleteBoxType(String id) throws ASFederatedDataServiceException {
throw new RuntimeException("not implemented.");
}
/**
* Deletes multiple BoxType objects.
*/
public void deleteBoxTypes(List list) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
public BoxType getBoxType(String id) throws ASFederatedDataServiceException {
String tokens[] = StringUtils.tokenize(id, ID_DELIMITER);
if (tokens[0].equals("G")){
try{
return copy(federatedG.getGenericBoxType(tokens[1]));
}catch(ASGenericDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
}
if (tokens[0].equals("C")){
try{
return copy(federatedC.getCustomBoxType(tokens[1]));
}catch(ASCustomDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
}
throw new RuntimeException("Unknown federated key: "+tokens[0]+" in "+id);
}
public BoxType importBoxType(BoxType boxtype) throws ASFederatedDataServiceException {
throw new RuntimeException("no import in federated services.");
}
public List importBoxTypes(List list) throws ASFederatedDataServiceException {
throw new RuntimeException("no import in federated services.");
}
public BoxType createBoxType(BoxType boxtype) throws ASFederatedDataServiceException {
throw new AssertionError("not implemented.");
}
/**
* Creates multiple new BoxType objects.
* Returns the created versions.
*/
public List createBoxTypes(List list) throws ASFederatedDataServiceException {
throw new AssertionError("not implemented.");
}
/**
* Updates multiple new BoxType objects.
* Returns the updated versions.
*/
public List updateBoxTypes(List list) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
public BoxType updateBoxType(BoxType boxtype) throws ASFederatedDataServiceException {
throw new AssertionError("not implemented.");
}
public List getBoxTypesByProperty(String propertyName, Object value) throws ASFederatedDataServiceException {
List boxtypes = new ArrayList();
try{
List sourceG = federatedG.getGenericBoxTypesByProperty(propertyName, value);
boxtypes.addAll(copyBoxTypeListG(sourceG));
}catch(ASGenericDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
try{
List sourceC = federatedC.getCustomBoxTypesByProperty(propertyName, value);
boxtypes.addAll(copyBoxTypeListC(sourceC));
}catch(ASCustomDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
return boxtypes;
}
public List getBoxTypesByProperty(String propertyName, Object value, SortType sortType) throws ASFederatedDataServiceException {
return StaticQuickSorter.sort(getBoxTypesByProperty(propertyName, value), sortType);
}
/**
* Executes a query on BoxTypes
*/
public QueryResult executeQueryOnBoxTypes(DocumentQuery query) throws ASFederatedDataServiceException {
List allBoxTypes = getBoxTypes();
QueryResult result = new QueryResult();
for (int i=0; i partialResult = query.match(allBoxTypes.get(i));
result.add(partialResult);
}
return result;
}
/**
* Returns all BoxType objects, where property matches.
*/
public List getBoxTypesByProperty(QueryProperty... property) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
/**
* Returns all BoxType objects, where property matches, sorted
*/
public List getBoxTypesByProperty(SortType sortType, QueryProperty... property) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
/**
* Returns BoxType objects count.
*/
public int getBoxTypesCount() throws ASFederatedDataServiceException {
int pCount = 0;
try {
pCount = pCount + federatedG.getGenericBoxTypes().size();
} catch (ASGenericDataServiceException e) {
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
try {
pCount = pCount + federatedC.getCustomBoxTypes().size();
} catch (ASCustomDataServiceException e) {
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
return pCount;
}
/**
* Returns BoxType objects segment.
*/
public List getBoxTypes(Segment aSegment) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
/**
* Returns BoxType objects segment, where property matched.
*/
public List getBoxTypesByProperty(Segment aSegment, QueryProperty... aProperty) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
/**
* Returns BoxType objects segment, where property matched, sorted.
*/
public List getBoxTypesByProperty(Segment aSegment, SortType aSortType, QueryProperty... aProperty) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
public XMLNode exportBoxTypesToXML(List listBoxTypes){;
return new XMLNode("unimplemented_federated_export_ASFederatedData");
}
public XMLNode exportBoxTypesToXML(String[] languages,List listBoxTypes) throws ASFederatedDataServiceException {
return new XMLNode("unimplemented_federated_export_ASFederatedData");
}
@Override
public void fetchBoxType(final String id, Set addedDocuments, JSONArray data) throws ASFederatedDataServiceException {
if (id.isEmpty() || addedDocuments.contains("BoxType" + id))
return;
try {
BoxType aBoxType = getBoxType(id);
addedDocuments.add("BoxType" + id);
String targetBoxTypeId = aBoxType.getId();
if (targetBoxTypeId.charAt(0) == 'G')
getASGenericDataService().fetchGenericBoxType(targetBoxTypeId.substring(2), addedDocuments, data);
if (targetBoxTypeId.charAt(0) == 'C')
getASCustomDataService().fetchCustomBoxType(targetBoxTypeId.substring(2), addedDocuments, data);
}catch(ASGenericDataServiceException e){
throw new ASFederatedDataServiceException ("Problem with getting GenericBoxType by id:" + id + "." + e.getMessage());
}catch(ASCustomDataServiceException e){
throw new ASFederatedDataServiceException ("Problem with getting CustomBoxType by id:" + id + "." + e.getMessage());
}
}
private BoxHandlerDef copy(GenericBoxHandlerDef d){
BoxHandlerDef ret = BoxHandlerDefFactory.createBoxHandlerDef(ID_PREFIX_G+d.getId());
ret.setName(d.getName());
ret.setClazz(d.getClazz());
((BoxHandlerDefVO)ret).setLastUpdateTimestamp(d.getLastUpdateTimestamp());
return ret;
}
private List copyBoxHandlerDefListG(List list){
List ret = new ArrayList(list.size());
for (GenericBoxHandlerDef d : list)
ret.add(copy(d));
return ret;
}
private BoxHandlerDef copy(CustomBoxHandlerDef d){
BoxHandlerDef ret = BoxHandlerDefFactory.createBoxHandlerDef(ID_PREFIX_C+d.getId());
ret.setName(d.getName());
ret.setClazz(d.getClazz());
((BoxHandlerDefVO)ret).setLastUpdateTimestamp(d.getLastUpdateTimestamp());
return ret;
}
private List copyBoxHandlerDefListC(List list){
List ret = new ArrayList(list.size());
for (CustomBoxHandlerDef d : list)
ret.add(copy(d));
return ret;
}
public List getBoxHandlerDefs() throws ASFederatedDataServiceException {
List boxhandlerdefs = new ArrayList();
try{
List sourceG = federatedG.getGenericBoxHandlerDefs();
boxhandlerdefs.addAll(copyBoxHandlerDefListG(sourceG));
}catch(ASGenericDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
try{
List sourceC = federatedC.getCustomBoxHandlerDefs();
boxhandlerdefs.addAll(copyBoxHandlerDefListC(sourceC));
}catch(ASCustomDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
return boxhandlerdefs;
}
public List getBoxHandlerDefs(SortType sortType) throws ASFederatedDataServiceException {
return StaticQuickSorter.sort(getBoxHandlerDefs(), sortType);
}
public void deleteBoxHandlerDef(BoxHandlerDef boxhandlerdef) throws ASFederatedDataServiceException {
deleteBoxHandlerDef(boxhandlerdef.getId());
}
public void deleteBoxHandlerDef(String id) throws ASFederatedDataServiceException {
throw new RuntimeException("not implemented.");
}
/**
* Deletes multiple BoxHandlerDef objects.
*/
public void deleteBoxHandlerDefs(List list) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
public BoxHandlerDef getBoxHandlerDef(String id) throws ASFederatedDataServiceException {
String tokens[] = StringUtils.tokenize(id, ID_DELIMITER);
if (tokens[0].equals("G")){
try{
return copy(federatedG.getGenericBoxHandlerDef(tokens[1]));
}catch(ASGenericDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
}
if (tokens[0].equals("C")){
try{
return copy(federatedC.getCustomBoxHandlerDef(tokens[1]));
}catch(ASCustomDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
}
throw new RuntimeException("Unknown federated key: "+tokens[0]+" in "+id);
}
public BoxHandlerDef importBoxHandlerDef(BoxHandlerDef boxhandlerdef) throws ASFederatedDataServiceException {
throw new RuntimeException("no import in federated services.");
}
public List importBoxHandlerDefs(List list) throws ASFederatedDataServiceException {
throw new RuntimeException("no import in federated services.");
}
public BoxHandlerDef createBoxHandlerDef(BoxHandlerDef boxhandlerdef) throws ASFederatedDataServiceException {
throw new AssertionError("not implemented.");
}
/**
* Creates multiple new BoxHandlerDef objects.
* Returns the created versions.
*/
public List createBoxHandlerDefs(List list) throws ASFederatedDataServiceException {
throw new AssertionError("not implemented.");
}
/**
* Updates multiple new BoxHandlerDef objects.
* Returns the updated versions.
*/
public List updateBoxHandlerDefs(List list) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
public BoxHandlerDef updateBoxHandlerDef(BoxHandlerDef boxhandlerdef) throws ASFederatedDataServiceException {
throw new AssertionError("not implemented.");
}
public List getBoxHandlerDefsByProperty(String propertyName, Object value) throws ASFederatedDataServiceException {
List boxhandlerdefs = new ArrayList();
try{
List sourceG = federatedG.getGenericBoxHandlerDefsByProperty(propertyName, value);
boxhandlerdefs.addAll(copyBoxHandlerDefListG(sourceG));
}catch(ASGenericDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
try{
List sourceC = federatedC.getCustomBoxHandlerDefsByProperty(propertyName, value);
boxhandlerdefs.addAll(copyBoxHandlerDefListC(sourceC));
}catch(ASCustomDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
return boxhandlerdefs;
}
public List getBoxHandlerDefsByProperty(String propertyName, Object value, SortType sortType) throws ASFederatedDataServiceException {
return StaticQuickSorter.sort(getBoxHandlerDefsByProperty(propertyName, value), sortType);
}
/**
* Executes a query on BoxHandlerDefs
*/
public QueryResult executeQueryOnBoxHandlerDefs(DocumentQuery query) throws ASFederatedDataServiceException {
List allBoxHandlerDefs = getBoxHandlerDefs();
QueryResult result = new QueryResult();
for (int i=0; i partialResult = query.match(allBoxHandlerDefs.get(i));
result.add(partialResult);
}
return result;
}
/**
* Returns all BoxHandlerDef objects, where property matches.
*/
public List getBoxHandlerDefsByProperty(QueryProperty... property) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
/**
* Returns all BoxHandlerDef objects, where property matches, sorted
*/
public List getBoxHandlerDefsByProperty(SortType sortType, QueryProperty... property) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
/**
* Returns BoxHandlerDef objects count.
*/
public int getBoxHandlerDefsCount() throws ASFederatedDataServiceException {
int pCount = 0;
try {
pCount = pCount + federatedG.getGenericBoxHandlerDefs().size();
} catch (ASGenericDataServiceException e) {
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
try {
pCount = pCount + federatedC.getCustomBoxHandlerDefs().size();
} catch (ASCustomDataServiceException e) {
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
return pCount;
}
/**
* Returns BoxHandlerDef objects segment.
*/
public List getBoxHandlerDefs(Segment aSegment) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
/**
* Returns BoxHandlerDef objects segment, where property matched.
*/
public List getBoxHandlerDefsByProperty(Segment aSegment, QueryProperty... aProperty) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
/**
* Returns BoxHandlerDef objects segment, where property matched, sorted.
*/
public List getBoxHandlerDefsByProperty(Segment aSegment, SortType aSortType, QueryProperty... aProperty) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
public XMLNode exportBoxHandlerDefsToXML(List listBoxHandlerDefs){;
return new XMLNode("unimplemented_federated_export_ASFederatedData");
}
public XMLNode exportBoxHandlerDefsToXML(String[] languages,List listBoxHandlerDefs) throws ASFederatedDataServiceException {
return new XMLNode("unimplemented_federated_export_ASFederatedData");
}
@Override
public void fetchBoxHandlerDef(final String id, Set addedDocuments, JSONArray data) throws ASFederatedDataServiceException {
if (id.isEmpty() || addedDocuments.contains("BoxHandlerDef" + id))
return;
try {
BoxHandlerDef aBoxHandlerDef = getBoxHandlerDef(id);
addedDocuments.add("BoxHandlerDef" + id);
String targetBoxHandlerDefId = aBoxHandlerDef.getId();
if (targetBoxHandlerDefId.charAt(0) == 'G')
getASGenericDataService().fetchGenericBoxHandlerDef(targetBoxHandlerDefId.substring(2), addedDocuments, data);
if (targetBoxHandlerDefId.charAt(0) == 'C')
getASCustomDataService().fetchCustomBoxHandlerDef(targetBoxHandlerDefId.substring(2), addedDocuments, data);
}catch(ASGenericDataServiceException e){
throw new ASFederatedDataServiceException ("Problem with getting GenericBoxHandlerDef by id:" + id + "." + e.getMessage());
}catch(ASCustomDataServiceException e){
throw new ASFederatedDataServiceException ("Problem with getting CustomBoxHandlerDef by id:" + id + "." + e.getMessage());
}
}
private GuardDef copy(GenericGuardDef d){
GuardDef ret = GuardDefFactory.createGuardDef(ID_PREFIX_G+d.getId());
ret.setName(d.getName());
ret.setClazz(d.getClazz());
ret.setParameter1(d.getParameter1());
ret.setParameter2(d.getParameter2());
ret.setParameter3(d.getParameter3());
ret.setParameter4(d.getParameter4());
ret.setParameter5(d.getParameter5());
ret.setDescription(d.getDescription());
((GuardDefVO)ret).setLastUpdateTimestamp(d.getLastUpdateTimestamp());
return ret;
}
private List copyGuardDefListG(List list){
List ret = new ArrayList(list.size());
for (GenericGuardDef d : list)
ret.add(copy(d));
return ret;
}
private GuardDef copy(CustomGuardDef d){
GuardDef ret = GuardDefFactory.createGuardDef(ID_PREFIX_C+d.getId());
ret.setName(d.getName());
ret.setClazz(d.getClazz());
ret.setParameter1(d.getParameter1());
ret.setParameter2(d.getParameter2());
ret.setParameter3(d.getParameter3());
ret.setParameter4(d.getParameter4());
ret.setParameter5(d.getParameter5());
ret.setDescription(d.getDescription());
((GuardDefVO)ret).setLastUpdateTimestamp(d.getLastUpdateTimestamp());
return ret;
}
private List copyGuardDefListC(List list){
List ret = new ArrayList(list.size());
for (CustomGuardDef d : list)
ret.add(copy(d));
return ret;
}
public List getGuardDefs() throws ASFederatedDataServiceException {
List guarddefs = new ArrayList();
try{
List sourceG = federatedG.getGenericGuardDefs();
guarddefs.addAll(copyGuardDefListG(sourceG));
}catch(ASGenericDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
try{
List sourceC = federatedC.getCustomGuardDefs();
guarddefs.addAll(copyGuardDefListC(sourceC));
}catch(ASCustomDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
return guarddefs;
}
public List getGuardDefs(SortType sortType) throws ASFederatedDataServiceException {
return StaticQuickSorter.sort(getGuardDefs(), sortType);
}
public void deleteGuardDef(GuardDef guarddef) throws ASFederatedDataServiceException {
deleteGuardDef(guarddef.getId());
}
public void deleteGuardDef(String id) throws ASFederatedDataServiceException {
throw new RuntimeException("not implemented.");
}
/**
* Deletes multiple GuardDef objects.
*/
public void deleteGuardDefs(List list) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
public GuardDef getGuardDef(String id) throws ASFederatedDataServiceException {
String tokens[] = StringUtils.tokenize(id, ID_DELIMITER);
if (tokens[0].equals("G")){
try{
return copy(federatedG.getGenericGuardDef(tokens[1]));
}catch(ASGenericDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
}
if (tokens[0].equals("C")){
try{
return copy(federatedC.getCustomGuardDef(tokens[1]));
}catch(ASCustomDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
}
throw new RuntimeException("Unknown federated key: "+tokens[0]+" in "+id);
}
public GuardDef importGuardDef(GuardDef guarddef) throws ASFederatedDataServiceException {
throw new RuntimeException("no import in federated services.");
}
public List importGuardDefs(List list) throws ASFederatedDataServiceException {
throw new RuntimeException("no import in federated services.");
}
public GuardDef createGuardDef(GuardDef guarddef) throws ASFederatedDataServiceException {
throw new AssertionError("not implemented.");
}
/**
* Creates multiple new GuardDef objects.
* Returns the created versions.
*/
public List createGuardDefs(List list) throws ASFederatedDataServiceException {
throw new AssertionError("not implemented.");
}
/**
* Updates multiple new GuardDef objects.
* Returns the updated versions.
*/
public List updateGuardDefs(List list) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
public GuardDef updateGuardDef(GuardDef guarddef) throws ASFederatedDataServiceException {
throw new AssertionError("not implemented.");
}
public List getGuardDefsByProperty(String propertyName, Object value) throws ASFederatedDataServiceException {
List guarddefs = new ArrayList();
try{
List sourceG = federatedG.getGenericGuardDefsByProperty(propertyName, value);
guarddefs.addAll(copyGuardDefListG(sourceG));
}catch(ASGenericDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
try{
List sourceC = federatedC.getCustomGuardDefsByProperty(propertyName, value);
guarddefs.addAll(copyGuardDefListC(sourceC));
}catch(ASCustomDataServiceException e){
// TODO Add logging?
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
return guarddefs;
}
public List getGuardDefsByProperty(String propertyName, Object value, SortType sortType) throws ASFederatedDataServiceException {
return StaticQuickSorter.sort(getGuardDefsByProperty(propertyName, value), sortType);
}
/**
* Executes a query on GuardDefs
*/
public QueryResult executeQueryOnGuardDefs(DocumentQuery query) throws ASFederatedDataServiceException {
List allGuardDefs = getGuardDefs();
QueryResult result = new QueryResult();
for (int i=0; i partialResult = query.match(allGuardDefs.get(i));
result.add(partialResult);
}
return result;
}
/**
* Returns all GuardDef objects, where property matches.
*/
public List getGuardDefsByProperty(QueryProperty... property) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
/**
* Returns all GuardDef objects, where property matches, sorted
*/
public List getGuardDefsByProperty(SortType sortType, QueryProperty... property) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
/**
* Returns GuardDef objects count.
*/
public int getGuardDefsCount() throws ASFederatedDataServiceException {
int pCount = 0;
try {
pCount = pCount + federatedG.getGenericGuardDefs().size();
} catch (ASGenericDataServiceException e) {
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
try {
pCount = pCount + federatedC.getCustomGuardDefs().size();
} catch (ASCustomDataServiceException e) {
throw new ASFederatedDataServiceException("Undelying service failed: "+e.getMessage());
}
return pCount;
}
/**
* Returns GuardDef objects segment.
*/
public List getGuardDefs(Segment aSegment) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
/**
* Returns GuardDef objects segment, where property matched.
*/
public List getGuardDefsByProperty(Segment aSegment, QueryProperty... aProperty) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
/**
* Returns GuardDef objects segment, where property matched, sorted.
*/
public List getGuardDefsByProperty(Segment aSegment, SortType aSortType, QueryProperty... aProperty) throws ASFederatedDataServiceException {
throw new RuntimeException("Not yet implemented");
}
public XMLNode exportGuardDefsToXML(List listGuardDefs){;
return new XMLNode("unimplemented_federated_export_ASFederatedData");
}
public XMLNode exportGuardDefsToXML(String[] languages,List listGuardDefs) throws ASFederatedDataServiceException {
return new XMLNode("unimplemented_federated_export_ASFederatedData");
}
@Override
public void fetchGuardDef(final String id, Set addedDocuments, JSONArray data) throws ASFederatedDataServiceException {
if (id.isEmpty() || addedDocuments.contains("GuardDef" + id))
return;
try {
GuardDef aGuardDef = getGuardDef(id);
addedDocuments.add("GuardDef" + id);
String targetGuardDefId = aGuardDef.getId();
if (targetGuardDefId.charAt(0) == 'G')
getASGenericDataService().fetchGenericGuardDef(targetGuardDefId.substring(2), addedDocuments, data);
if (targetGuardDefId.charAt(0) == 'C')
getASCustomDataService().fetchCustomGuardDef(targetGuardDefId.substring(2), addedDocuments, data);
}catch(ASGenericDataServiceException e){
throw new ASFederatedDataServiceException ("Problem with getting GenericGuardDef by id:" + id + "." + e.getMessage());
}catch(ASCustomDataServiceException e){
throw new ASFederatedDataServiceException ("Problem with getting CustomGuardDef by id:" + id + "." + e.getMessage());
}
}
public void executeParsingForDocument (final DocumentName documentName, final JSONObject data) throws ASFederatedDataServiceException {
throw new UnsupportedOperationException(" not implemented and should not BE!");
}
/**
* Executes a query on all data objects (documents, vo) which are part of this module and managed by this service
*/
public QueryResult executeQueryOnAllObjects(DocumentQuery query) throws ASFederatedDataServiceException {
QueryResult ret = new QueryResult();
ret.add(executeQueryOnBoxTypes(query).getEntries());
ret.add(executeQueryOnBoxHandlerDefs(query).getEntries());
ret.add(executeQueryOnGuardDefs(query).getEntries());
return ret;
} //executeQueryOnAllObjects
public XMLNode exportToXML() throws ASFederatedDataServiceException {
return new XMLNode("unimplemented_federated_export_ASFederatedData");
}
public XMLNode exportToXML(String[] languages) throws ASFederatedDataServiceException {
return new XMLNode("unimplemented_federated_export_ASFederatedData");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy