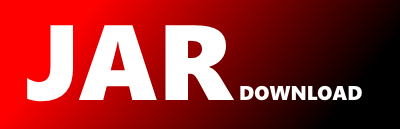
net.anotheria.anosite.gen.aslayoutdata.service.ASLayoutDataServiceImpl Maven / Gradle / Ivy
The newest version!
/**
********************************************************************************
*** ASLayoutDataServiceImpl.java ***
*** The implementation of the IASLayoutDataService. ***
*** generated by AnoSiteGenerator (ASG), Version: 4.2.2 ***
*** Copyright (C) 2005 - 2025 Anotheria.net, www.anotheria.net ***
*** All Rights Reserved. ***
********************************************************************************
*** Don't edit this code, if you aren't sure ***
*** that you do exactly know what you are doing! ***
*** It's better to invest time in the generator, as into the generated code. ***
********************************************************************************
*/
package net.anotheria.anosite.gen.aslayoutdata.service;
import java.nio.charset.Charset;
import java.util.List;
import java.util.ArrayList;
import java.util.Set;
import net.anotheria.anodoc.data.Module;
import net.anotheria.anodoc.data.Property;
import net.anotheria.anodoc.data.NoSuchPropertyException;
import net.anotheria.util.sorter.SortType;
import net.anotheria.util.sorter.StaticQuickSorter;
import net.anotheria.util.slicer.Segment;
import net.anotheria.util.slicer.Slicer;
import net.anotheria.anosite.gen.aslayoutdata.data.ModuleASLayoutData;
import net.anotheria.anosite.gen.shared.service.BasicCMSService;
import net.anotheria.anodoc.query2.DocumentQuery;
import net.anotheria.anodoc.query2.QueryResult;
import net.anotheria.anodoc.query2.QueryResultEntry;
import net.anotheria.anodoc.query2.QueryProperty;
import net.anotheria.util.StringUtils;
import net.anotheria.util.xml.XMLNode;
import net.anotheria.util.xml.XMLAttribute;
import net.anotheria.asg.util.listener.IModuleListener;
import org.codehaus.jettison.json.JSONObject;
import org.codehaus.jettison.json.JSONArray;
import org.codehaus.jettison.json.JSONException;
import com.fasterxml.jackson.core.JsonProcessingException;
import java.io.IOException;
import net.anotheria.anodoc.util.mapper.ObjectMapperUtil;
import net.anotheria.anosite.gen.shared.util.DocumentName;
import net.anotheria.anosite.gen.aslayoutdata.data.PageStyle;
import net.anotheria.anosite.gen.aslayoutdata.data.PageStyleXMLHelper;
import net.anotheria.anosite.gen.aslayoutdata.data.PageStyleDocument;
import net.anotheria.anosite.gen.aslayoutdata.data.PageLayout;
import net.anotheria.anosite.gen.aslayoutdata.data.PageLayoutXMLHelper;
import net.anotheria.anosite.gen.aslayoutdata.data.PageLayoutDocument;
import net.anotheria.anosite.gen.aslayoutdata.service.ASLayoutDataServiceException;
public class ASLayoutDataServiceImpl extends BasicCMSService implements IASLayoutDataService, IModuleListener{
// Generated by: class net.anotheria.asg.generator.model.docs.CMSBasedServiceGenerator.generateImplementation
private static ASLayoutDataServiceImpl instance;
private ASLayoutDataServiceImpl(){
addServiceListener(new net.anotheria.anosite.cms.listener.CRUDLogListener());
addServiceListener(new net.anotheria.anosite.cms.listener.AutoTransferLayoutListener());
addModuleListener(ModuleASLayoutData.MODULE_ID, this);
}
static final ASLayoutDataServiceImpl getInstance(){
if (instance==null){
instance = new ASLayoutDataServiceImpl();
}
return instance;
}
private ModuleASLayoutData _getModuleASLayoutData(){
return (ModuleASLayoutData) getModule(ModuleASLayoutData.MODULE_ID);
}
@Override
public void moduleLoaded(Module module){
firePersistenceChangedEvent();
}
@Override
public List getPageStyles(){
List pagestyles = new ArrayList<>();
pagestyles.addAll(_getModuleASLayoutData().getPageStyles());
return pagestyles;
}
@Override
public List getPageStyles(SortType sortType){
return StaticQuickSorter.sort(getPageStyles(), sortType);
}
/**
* Returns the PageStyle objects with the specified ids.
*/
public List getPageStyles(List ids){
if (ids==null || ids.size()==0)
return new ArrayList<>(0);
List all = getPageStyles();
List ret = new ArrayList<>();
for (PageStyle pagestyle : all){
if(ids.contains(pagestyle.getId())){
ret.add(pagestyle);
}
}
return ret;
}
/**
* Returns the PageStyle objects with the specified ids, sorted by given sorttype.
*/
public List getPageStyles(List ids, SortType sortType){
return StaticQuickSorter.sort(getPageStyles(ids), sortType);
}
@Override
public void deletePageStyle(PageStyle pagestyle){
deletePageStyle(pagestyle.getId());
if (hasServiceListeners()){
fireObjectDeletedEvent(pagestyle);
}
}
@Override
public void deletePageStyle(String id){
ModuleASLayoutData module = _getModuleASLayoutData();
PageStyle varValue = hasServiceListeners()?module.getPageStyle(id):null;
module.deletePageStyle(id);
updateModule(module);
if(varValue!=null){
fireObjectDeletedEvent(varValue);
}
}
@Override
public void deletePageStyles(List list){
ModuleASLayoutData module = _getModuleASLayoutData();
for (PageStyle pagestyle : list){
module.deletePageStyle(pagestyle.getId());
}
updateModule(module);
if (hasServiceListeners()){
for (int t=0; t importPageStyles(List list){
ModuleASLayoutData module = _getModuleASLayoutData();
List ret = new ArrayList<>();
for (PageStyle pagestyle : list){
PageStyle imported = module.importPageStyle((PageStyleDocument)pagestyle);
ret.add(imported);
}
updateModule(module);
if (hasServiceListeners()){
for (PageStyle pagestyle : ret)
fireObjectImportedEvent(pagestyle);
}
return ret;
}
@Override
public PageStyle createPageStyle(PageStyle pagestyle){
ModuleASLayoutData module = _getModuleASLayoutData();
module.createPageStyle((PageStyleDocument)pagestyle);
updateModule(module);
fireObjectCreatedEvent(pagestyle);
return pagestyle;
}
@Override
/**
* Creates multiple new PageStyle objects.
* Returns the created versions.
*/
public List createPageStyles(List list){
ModuleASLayoutData module = _getModuleASLayoutData();
List ret = new ArrayList<>();
for (PageStyle pagestyle : list){
PageStyle created = module.createPageStyle((PageStyleDocument)pagestyle);
ret.add(created);
}
updateModule(module);
if (hasServiceListeners()){
for (PageStyle pagestyle : ret)
fireObjectCreatedEvent(pagestyle);
}
return ret;
}
@Override
public PageStyle updatePageStyle(PageStyle pagestyle){
PageStyle oldVersion = null;
ModuleASLayoutData module = _getModuleASLayoutData();
if (hasServiceListeners())
oldVersion = module.getPageStyle(pagestyle.getId());
module.updatePageStyle((PageStyleDocument)pagestyle);
updateModule(module);
if (oldVersion != null){
fireObjectUpdatedEvent(oldVersion, pagestyle);
}
return pagestyle;
}
@Override
public List updatePageStyles(List list){
List oldList = null;
if (hasServiceListeners())
oldList = new ArrayList<>(list.size());
ModuleASLayoutData module = _getModuleASLayoutData();
for (PageStyle pagestyle : list){
if (oldList!=null)
oldList.add(module.getPageStyle(pagestyle.getId()));
module.updatePageStyle((PageStyleDocument)pagestyle);
}
updateModule(module);
if (oldList!=null){
for (int t=0; t getPageStylesByProperty(String propertyName, Object value){
List allPageStyles = getPageStyles();
List ret = new ArrayList<>();
for (int i=0; i getPageStylesByProperty(String propertyName, Object value, SortType sortType){
return StaticQuickSorter.sort(getPageStylesByProperty(propertyName, value), sortType);
}
/**
* Executes a query on PageStyles
*/
public QueryResult executeQueryOnPageStyles(DocumentQuery query){
List allPageStyles = getPageStyles();
QueryResult result = new QueryResult();
for (int i=0; i partialResult = query.match(allPageStyles.get(i));
result.add(partialResult);
}
return result;
}
/**
* Returns all PageStyle objects, where property matches.
*/
public List getPageStylesByProperty(QueryProperty... property){
//first the slow version, the fast version is a todo.
List ret = new ArrayList<>();
List src = getPageStyles();
for ( PageStyle pagestyle : src){
boolean mayPass = true;
for (QueryProperty qp : property){
mayPass = mayPass && qp.doesMatch(pagestyle.getPropertyValue(qp.getName()));
}
if (mayPass)
ret.add(pagestyle);
}
return ret;
}
/**
* Returns all PageStyle objects, where property matches, sorted
*/
public List getPageStylesByProperty(SortType sortType, QueryProperty... property){
return StaticQuickSorter.sort(getPageStylesByProperty(property), sortType);
}
/**
* Returns PageStyle objects count.
*/
public int getPageStylesCount() {
return _getModuleASLayoutData().getPageStyles().size();
}
/**
* Returns PageStyle objects segment.
*/
public List getPageStyles(Segment aSegment) {
return Slicer.slice(aSegment, getPageStyles()).getSliceData();
}
/**
* Returns PageStyle objects segment, where property matched.
*/
public List getPageStylesByProperty(Segment aSegment, QueryProperty... property) {
int pLimit = aSegment.getElementsPerSlice();
int pOffset = aSegment.getSliceNumber() * aSegment.getElementsPerSlice() - aSegment.getElementsPerSlice();
List ret = new ArrayList<>();
List src = getPageStyles();
for (PageStyle pagestyle : src) {
boolean mayPass = true;
for (QueryProperty qp : property) {
mayPass = mayPass && qp.doesMatch(pagestyle.getPropertyValue(qp.getName()));
}
if (mayPass)
ret.add(pagestyle);
if (ret.size() > pOffset + pLimit)
break;
}
return Slicer.slice(aSegment, ret).getSliceData();
}
/**
* Returns PageStyle objects segment, where property matched, sorted.
*/
public List getPageStylesByProperty(Segment aSegment, SortType aSortType, QueryProperty... aProperty){
return StaticQuickSorter.sort(getPageStylesByProperty(aSegment, aProperty), aSortType);
}
@Override
public void fetchPageStyle(final String id, Set addedDocuments, JSONArray data) throws ASLayoutDataServiceException {
if (id.isEmpty() || addedDocuments.contains("PageStyle" + id))
return;
try {
final PageStyleDocument pagestyle = _getModuleASLayoutData().getPageStyle(id);
addedDocuments.add("PageStyle" + id);
JSONObject dataObject = new JSONObject();
String jsonObject = ObjectMapperUtil.getMapperInstance().writeValueAsString(pagestyle);
dataObject.put("object", jsonObject);
dataObject.put("service", "ASLayoutData");
dataObject.put("document", "ASLayoutData_PageStyle");
data.put(dataObject);
}catch(IOException e){
throw new ASLayoutDataServiceException ("Problem with fetching data for this PageStyle instance object:" + e.getMessage());
}catch(JSONException e){
throw new ASLayoutDataServiceException ("Problem with fetching data for this PageStyle instance in json :" + e.getMessage());
}
}
private void saveTransferredPageStyle(final JSONObject data) throws ASLayoutDataServiceException {
try {
String objectData = data.getString("object");
PageStyle pagestyle = ObjectMapperUtil.getMapperInstance().readValue(objectData.getBytes(Charset.forName("UTF-8")), PageStyleDocument.class);
try {
updatePageStyle(pagestyle);
}catch(Exception e){
importPageStyle(pagestyle);
}
}catch(JSONException e){
throw new ASLayoutDataServiceException("Problem with getting data from json PageStyle instance :" + e.getMessage());
}catch(IOException e){
throw new ASLayoutDataServiceException("Problem with parsing data for this PageStyle instance :" + e.getMessage());
}
}
@Override
public List getPageLayouts(){
List pagelayouts = new ArrayList<>();
pagelayouts.addAll(_getModuleASLayoutData().getPageLayouts());
return pagelayouts;
}
@Override
public List getPageLayouts(SortType sortType){
return StaticQuickSorter.sort(getPageLayouts(), sortType);
}
/**
* Returns the PageLayout objects with the specified ids.
*/
public List getPageLayouts(List ids){
if (ids==null || ids.size()==0)
return new ArrayList<>(0);
List all = getPageLayouts();
List ret = new ArrayList<>();
for (PageLayout pagelayout : all){
if(ids.contains(pagelayout.getId())){
ret.add(pagelayout);
}
}
return ret;
}
/**
* Returns the PageLayout objects with the specified ids, sorted by given sorttype.
*/
public List getPageLayouts(List ids, SortType sortType){
return StaticQuickSorter.sort(getPageLayouts(ids), sortType);
}
@Override
public void deletePageLayout(PageLayout pagelayout){
deletePageLayout(pagelayout.getId());
if (hasServiceListeners()){
fireObjectDeletedEvent(pagelayout);
}
}
@Override
public void deletePageLayout(String id){
ModuleASLayoutData module = _getModuleASLayoutData();
PageLayout varValue = hasServiceListeners()?module.getPageLayout(id):null;
module.deletePageLayout(id);
updateModule(module);
if(varValue!=null){
fireObjectDeletedEvent(varValue);
}
}
@Override
public void deletePageLayouts(List list){
ModuleASLayoutData module = _getModuleASLayoutData();
for (PageLayout pagelayout : list){
module.deletePageLayout(pagelayout.getId());
}
updateModule(module);
if (hasServiceListeners()){
for (int t=0; t importPageLayouts(List list){
ModuleASLayoutData module = _getModuleASLayoutData();
List ret = new ArrayList<>();
for (PageLayout pagelayout : list){
PageLayout imported = module.importPageLayout((PageLayoutDocument)pagelayout);
ret.add(imported);
}
updateModule(module);
if (hasServiceListeners()){
for (PageLayout pagelayout : ret)
fireObjectImportedEvent(pagelayout);
}
return ret;
}
@Override
public PageLayout createPageLayout(PageLayout pagelayout){
ModuleASLayoutData module = _getModuleASLayoutData();
module.createPageLayout((PageLayoutDocument)pagelayout);
updateModule(module);
fireObjectCreatedEvent(pagelayout);
return pagelayout;
}
@Override
/**
* Creates multiple new PageLayout objects.
* Returns the created versions.
*/
public List createPageLayouts(List list){
ModuleASLayoutData module = _getModuleASLayoutData();
List ret = new ArrayList<>();
for (PageLayout pagelayout : list){
PageLayout created = module.createPageLayout((PageLayoutDocument)pagelayout);
ret.add(created);
}
updateModule(module);
if (hasServiceListeners()){
for (PageLayout pagelayout : ret)
fireObjectCreatedEvent(pagelayout);
}
return ret;
}
@Override
public PageLayout updatePageLayout(PageLayout pagelayout){
PageLayout oldVersion = null;
ModuleASLayoutData module = _getModuleASLayoutData();
if (hasServiceListeners())
oldVersion = module.getPageLayout(pagelayout.getId());
module.updatePageLayout((PageLayoutDocument)pagelayout);
updateModule(module);
if (oldVersion != null){
fireObjectUpdatedEvent(oldVersion, pagelayout);
}
return pagelayout;
}
@Override
public List updatePageLayouts(List list){
List oldList = null;
if (hasServiceListeners())
oldList = new ArrayList<>(list.size());
ModuleASLayoutData module = _getModuleASLayoutData();
for (PageLayout pagelayout : list){
if (oldList!=null)
oldList.add(module.getPageLayout(pagelayout.getId()));
module.updatePageLayout((PageLayoutDocument)pagelayout);
}
updateModule(module);
if (oldList!=null){
for (int t=0; t getPageLayoutsByProperty(String propertyName, Object value){
List allPageLayouts = getPageLayouts();
List ret = new ArrayList<>();
for (int i=0; i getPageLayoutsByProperty(String propertyName, Object value, SortType sortType){
return StaticQuickSorter.sort(getPageLayoutsByProperty(propertyName, value), sortType);
}
/**
* Executes a query on PageLayouts
*/
public QueryResult executeQueryOnPageLayouts(DocumentQuery query){
List allPageLayouts = getPageLayouts();
QueryResult result = new QueryResult();
for (int i=0; i partialResult = query.match(allPageLayouts.get(i));
result.add(partialResult);
}
return result;
}
/**
* Returns all PageLayout objects, where property matches.
*/
public List getPageLayoutsByProperty(QueryProperty... property){
//first the slow version, the fast version is a todo.
List ret = new ArrayList<>();
List src = getPageLayouts();
for ( PageLayout pagelayout : src){
boolean mayPass = true;
for (QueryProperty qp : property){
mayPass = mayPass && qp.doesMatch(pagelayout.getPropertyValue(qp.getName()));
}
if (mayPass)
ret.add(pagelayout);
}
return ret;
}
/**
* Returns all PageLayout objects, where property matches, sorted
*/
public List getPageLayoutsByProperty(SortType sortType, QueryProperty... property){
return StaticQuickSorter.sort(getPageLayoutsByProperty(property), sortType);
}
/**
* Returns PageLayout objects count.
*/
public int getPageLayoutsCount() {
return _getModuleASLayoutData().getPageLayouts().size();
}
/**
* Returns PageLayout objects segment.
*/
public List getPageLayouts(Segment aSegment) {
return Slicer.slice(aSegment, getPageLayouts()).getSliceData();
}
/**
* Returns PageLayout objects segment, where property matched.
*/
public List getPageLayoutsByProperty(Segment aSegment, QueryProperty... property) {
int pLimit = aSegment.getElementsPerSlice();
int pOffset = aSegment.getSliceNumber() * aSegment.getElementsPerSlice() - aSegment.getElementsPerSlice();
List ret = new ArrayList<>();
List src = getPageLayouts();
for (PageLayout pagelayout : src) {
boolean mayPass = true;
for (QueryProperty qp : property) {
mayPass = mayPass && qp.doesMatch(pagelayout.getPropertyValue(qp.getName()));
}
if (mayPass)
ret.add(pagelayout);
if (ret.size() > pOffset + pLimit)
break;
}
return Slicer.slice(aSegment, ret).getSliceData();
}
/**
* Returns PageLayout objects segment, where property matched, sorted.
*/
public List getPageLayoutsByProperty(Segment aSegment, SortType aSortType, QueryProperty... aProperty){
return StaticQuickSorter.sort(getPageLayoutsByProperty(aSegment, aProperty), aSortType);
}
@Override
public void fetchPageLayout(final String id, Set addedDocuments, JSONArray data) throws ASLayoutDataServiceException {
if (id.isEmpty() || addedDocuments.contains("PageLayout" + id))
return;
try {
final PageLayoutDocument pagelayout = _getModuleASLayoutData().getPageLayout(id);
addedDocuments.add("PageLayout" + id);
if (!StringUtils.isEmpty(pagelayout.getStyle()))
getASLayoutDataService().fetchPageStyle(pagelayout.getStyle(), addedDocuments, data);
JSONObject dataObject = new JSONObject();
String jsonObject = ObjectMapperUtil.getMapperInstance().writeValueAsString(pagelayout);
dataObject.put("object", jsonObject);
dataObject.put("service", "ASLayoutData");
dataObject.put("document", "ASLayoutData_PageLayout");
data.put(dataObject);
}catch(ASLayoutDataServiceException e){
throw new ASLayoutDataServiceException("Problem with getting document from ASLayoutData" + e.getMessage());
}catch(IOException e){
throw new ASLayoutDataServiceException ("Problem with fetching data for this PageLayout instance object:" + e.getMessage());
}catch(JSONException e){
throw new ASLayoutDataServiceException ("Problem with fetching data for this PageLayout instance in json :" + e.getMessage());
}
}
private void saveTransferredPageLayout(final JSONObject data) throws ASLayoutDataServiceException {
try {
String objectData = data.getString("object");
PageLayout pagelayout = ObjectMapperUtil.getMapperInstance().readValue(objectData.getBytes(Charset.forName("UTF-8")), PageLayoutDocument.class);
try {
updatePageLayout(pagelayout);
}catch(Exception e){
importPageLayout(pagelayout);
}
}catch(JSONException e){
throw new ASLayoutDataServiceException("Problem with getting data from json PageLayout instance :" + e.getMessage());
}catch(IOException e){
throw new ASLayoutDataServiceException("Problem with parsing data for this PageLayout instance :" + e.getMessage());
}
}
public void executeParsingForDocument (final DocumentName documentName, final JSONObject data) throws ASLayoutDataServiceException {
switch(documentName) {
case DOCUMENT_ASLAYOUTDATA_PAGESTYLE:
saveTransferredPageStyle(data);
break;
case DOCUMENT_ASLAYOUTDATA_PAGELAYOUT:
saveTransferredPageLayout(data);
break;
default:
log.info("There is no correct document: " + documentName + "in this service");
throw new ASLayoutDataServiceException("No such document");
}
}
/**
* Executes a query on all data objects (documents, vo) which are part of this module and managed by this service
*/
public QueryResult executeQueryOnAllObjects(DocumentQuery query){
QueryResult ret = new QueryResult();
ret.add(executeQueryOnPageStyles(query).getEntries());
ret.add(executeQueryOnPageLayouts(query).getEntries());
return ret;
} //executeQueryOnAllObjects
public XMLNode exportPageStylesToXML(){
XMLNode ret = new XMLNode("PageStyles");
List list = getPageStyles();
ret.addAttribute(new XMLAttribute("count", list.size()));
for (PageStyle object : list)
ret.addChildNode(PageStyleXMLHelper.toXML(object));
return ret;
}
public XMLNode exportPageStylesToXML(List list){
XMLNode ret = new XMLNode("PageStyles");
ret.addAttribute(new XMLAttribute("count", list.size()));
for (PageStyle object : list)
ret.addChildNode(PageStyleXMLHelper.toXML(object));
return ret;
}
public XMLNode exportPageLayoutsToXML(){
XMLNode ret = new XMLNode("PageLayouts");
List list = getPageLayouts();
ret.addAttribute(new XMLAttribute("count", list.size()));
for (PageLayout object : list)
ret.addChildNode(PageLayoutXMLHelper.toXML(object));
return ret;
}
public XMLNode exportPageLayoutsToXML(List list){
XMLNode ret = new XMLNode("PageLayouts");
ret.addAttribute(new XMLAttribute("count", list.size()));
for (PageLayout object : list)
ret.addChildNode(PageLayoutXMLHelper.toXML(object));
return ret;
}
public XMLNode exportToXML(){
XMLNode ret = new XMLNode("ASLayoutData");
ret.addChildNode(exportPageStylesToXML());
ret.addChildNode(exportPageLayoutsToXML());
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy