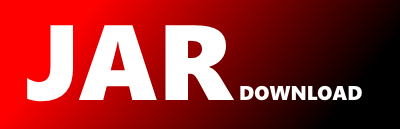
net.anotheria.anosite.gen.assitedata.data.PageTemplate Maven / Gradle / Ivy
The newest version!
/**
********************************************************************************
*** PageTemplate.java ***
*** generated by AnoSiteGenerator (ASG), Version: 4.2.2 ***
*** Copyright (C) 2005 - 2025 Anotheria.net, www.anotheria.net ***
*** All Rights Reserved. ***
********************************************************************************
*** Don't edit this code, if you aren't sure ***
*** that you do exactly know what you are doing! ***
*** It's better to invest time in the generator, as into the generated code. ***
********************************************************************************
*/
package net.anotheria.anosite.gen.assitedata.data;
import net.anotheria.asg.data.DataObject;
import java.util.List;
import net.anotheria.util.sorter.IComparable;
public interface PageTemplate extends DataObject, IComparable {
/**
* Constant property name for "id" for internal storage and queries.
*/
String PROP_ID = "id";
/**
* Constant property name for "name" for internal storage and queries.
*/
String PROP_NAME = "name";
/**
* Constant property name for "mediaLinks" for internal storage and queries.
*/
String PROP_MEDIA_LINKS = "mediaLinks";
/**
* Constant property name for "scripts" for internal storage and queries.
*/
String PROP_SCRIPTS = "scripts";
/**
* Constant property name for "c1first" for internal storage and queries.
*/
String PROP_C1FIRST = "c1first";
/**
* Constant property name for "c2first" for internal storage and queries.
*/
String PROP_C2FIRST = "c2first";
/**
* Constant property name for "c3first" for internal storage and queries.
*/
String PROP_C3FIRST = "c3first";
/**
* Constant property name for "c1last" for internal storage and queries.
*/
String PROP_C1LAST = "c1last";
/**
* Constant property name for "c2last" for internal storage and queries.
*/
String PROP_C2LAST = "c2last";
/**
* Constant property name for "c3last" for internal storage and queries.
*/
String PROP_C3LAST = "c3last";
/**
* Constant property name for "meta" for internal storage and queries.
*/
String PROP_META = "meta";
/**
* Constant property name for "header" for internal storage and queries.
*/
String PROP_HEADER = "header";
/**
* Constant property name for "footer" for internal storage and queries.
*/
String PROP_FOOTER = "footer";
/**
* Constant property name for "localizations" for internal storage and queries.
*/
String PROP_LOCALIZATIONS = "localizations";
/**
* Constant property name for "description" for internal storage and queries.
*/
String PROP_DESCRIPTION = "description";
/**
* Constant property name for "layout" for internal storage and queries.
*/
String LINK_PROP_LAYOUT = "layout";
/**
* Constant property name for "site" for internal storage and queries.
*/
String LINK_PROP_SITE = "site";
/**
* Returns the value of the name attribute.
*/
String getName();
/**
* Sets the value of the name attribute.
*/
void setName(String value);
/**
* Returns the value of the mediaLinks attribute.
*/
List getMediaLinks();
/**
* Sets the value of the mediaLinks attribute.
*/
void setMediaLinks(List value);
/**
* Returns the value of the scripts attribute.
*/
List getScripts();
/**
* Sets the value of the scripts attribute.
*/
void setScripts(List value);
/**
* Returns the value of the c1first attribute.
*/
List getC1first();
/**
* Sets the value of the c1first attribute.
*/
void setC1first(List value);
/**
* Returns the value of the c2first attribute.
*/
List getC2first();
/**
* Sets the value of the c2first attribute.
*/
void setC2first(List value);
/**
* Returns the value of the c3first attribute.
*/
List getC3first();
/**
* Sets the value of the c3first attribute.
*/
void setC3first(List value);
/**
* Returns the value of the c1last attribute.
*/
List getC1last();
/**
* Sets the value of the c1last attribute.
*/
void setC1last(List value);
/**
* Returns the value of the c2last attribute.
*/
List getC2last();
/**
* Sets the value of the c2last attribute.
*/
void setC2last(List value);
/**
* Returns the value of the c3last attribute.
*/
List getC3last();
/**
* Sets the value of the c3last attribute.
*/
void setC3last(List value);
/**
* Returns the value of the meta attribute.
*/
List getMeta();
/**
* Sets the value of the meta attribute.
*/
void setMeta(List value);
/**
* Returns the value of the header attribute.
*/
List getHeader();
/**
* Sets the value of the header attribute.
*/
void setHeader(List value);
/**
* Returns the value of the footer attribute.
*/
List getFooter();
/**
* Sets the value of the footer attribute.
*/
void setFooter(List value);
/**
* Returns the value of the localizations attribute.
*/
List getLocalizations();
/**
* Sets the value of the localizations attribute.
*/
void setLocalizations(List value);
/**
* Returns the value of the description attribute.
*/
String getDescription();
/**
* Sets the value of the description attribute.
*/
void setDescription(String value);
/**
* Returns the value of the layout attribute.
*/
String getLayout();
/**
* Sets the value of the layout attribute.
*/
void setLayout(String value);
/**
* Returns the value of the site attribute.
*/
String getSite();
/**
* Sets the value of the site attribute.
*/
void setSite(String value);
/**
* Returns the number of elements in the "mediaLinks" container
*/
int getMediaLinksSize();
/**
* Adds a new element to the list.
*/
void addMediaLinksElement(String mediaLinks);
/**
* Removes the element at position index from the list.
*/
void removeMediaLinksElement(int index);
/**
* Swaps elements at positions index1 and index2 in the list.
*/
void swapMediaLinksElement(int index1, int index2);
/**
* Returns the element at the position index in the list.
*/
String getMediaLinksElement(int index);
/**
* Returns the number of elements in the "scripts" container
*/
int getScriptsSize();
/**
* Adds a new element to the list.
*/
void addScriptsElement(String scripts);
/**
* Removes the element at position index from the list.
*/
void removeScriptsElement(int index);
/**
* Swaps elements at positions index1 and index2 in the list.
*/
void swapScriptsElement(int index1, int index2);
/**
* Returns the element at the position index in the list.
*/
String getScriptsElement(int index);
/**
* Returns the number of elements in the "c1first" container
*/
int getC1firstSize();
/**
* Adds a new element to the list.
*/
void addC1firstElement(String box);
/**
* Removes the element at position index from the list.
*/
void removeC1firstElement(int index);
/**
* Swaps elements at positions index1 and index2 in the list.
*/
void swapC1firstElement(int index1, int index2);
/**
* Returns the element at the position index in the list.
*/
String getC1firstElement(int index);
/**
* Returns the number of elements in the "c2first" container
*/
int getC2firstSize();
/**
* Adds a new element to the list.
*/
void addC2firstElement(String box);
/**
* Removes the element at position index from the list.
*/
void removeC2firstElement(int index);
/**
* Swaps elements at positions index1 and index2 in the list.
*/
void swapC2firstElement(int index1, int index2);
/**
* Returns the element at the position index in the list.
*/
String getC2firstElement(int index);
/**
* Returns the number of elements in the "c3first" container
*/
int getC3firstSize();
/**
* Adds a new element to the list.
*/
void addC3firstElement(String box);
/**
* Removes the element at position index from the list.
*/
void removeC3firstElement(int index);
/**
* Swaps elements at positions index1 and index2 in the list.
*/
void swapC3firstElement(int index1, int index2);
/**
* Returns the element at the position index in the list.
*/
String getC3firstElement(int index);
/**
* Returns the number of elements in the "c1last" container
*/
int getC1lastSize();
/**
* Adds a new element to the list.
*/
void addC1lastElement(String box);
/**
* Removes the element at position index from the list.
*/
void removeC1lastElement(int index);
/**
* Swaps elements at positions index1 and index2 in the list.
*/
void swapC1lastElement(int index1, int index2);
/**
* Returns the element at the position index in the list.
*/
String getC1lastElement(int index);
/**
* Returns the number of elements in the "c2last" container
*/
int getC2lastSize();
/**
* Adds a new element to the list.
*/
void addC2lastElement(String box);
/**
* Removes the element at position index from the list.
*/
void removeC2lastElement(int index);
/**
* Swaps elements at positions index1 and index2 in the list.
*/
void swapC2lastElement(int index1, int index2);
/**
* Returns the element at the position index in the list.
*/
String getC2lastElement(int index);
/**
* Returns the number of elements in the "c3last" container
*/
int getC3lastSize();
/**
* Adds a new element to the list.
*/
void addC3lastElement(String box);
/**
* Removes the element at position index from the list.
*/
void removeC3lastElement(int index);
/**
* Swaps elements at positions index1 and index2 in the list.
*/
void swapC3lastElement(int index1, int index2);
/**
* Returns the element at the position index in the list.
*/
String getC3lastElement(int index);
/**
* Returns the number of elements in the "meta" container
*/
int getMetaSize();
/**
* Adds a new element to the list.
*/
void addMetaElement(String box);
/**
* Removes the element at position index from the list.
*/
void removeMetaElement(int index);
/**
* Swaps elements at positions index1 and index2 in the list.
*/
void swapMetaElement(int index1, int index2);
/**
* Returns the element at the position index in the list.
*/
String getMetaElement(int index);
/**
* Returns the number of elements in the "header" container
*/
int getHeaderSize();
/**
* Adds a new element to the list.
*/
void addHeaderElement(String box);
/**
* Removes the element at position index from the list.
*/
void removeHeaderElement(int index);
/**
* Swaps elements at positions index1 and index2 in the list.
*/
void swapHeaderElement(int index1, int index2);
/**
* Returns the element at the position index in the list.
*/
String getHeaderElement(int index);
/**
* Returns the number of elements in the "footer" container
*/
int getFooterSize();
/**
* Adds a new element to the list.
*/
void addFooterElement(String box);
/**
* Removes the element at position index from the list.
*/
void removeFooterElement(int index);
/**
* Swaps elements at positions index1 and index2 in the list.
*/
void swapFooterElement(int index1, int index2);
/**
* Returns the element at the position index in the list.
*/
String getFooterElement(int index);
/**
* Returns the number of elements in the "localizations" container
*/
int getLocalizationsSize();
/**
* Adds a new element to the list.
*/
void addLocalizationsElement(String localization);
/**
* Removes the element at position index from the list.
*/
void removeLocalizationsElement(int index);
/**
* Swaps elements at positions index1 and index2 in the list.
*/
void swapLocalizationsElement(int index1, int index2);
/**
* Returns the element at the position index in the list.
*/
String getLocalizationsElement(int index);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy