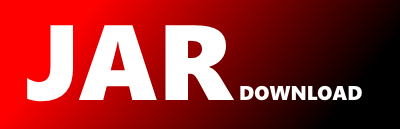
net.anotheria.anosite.gen.assitedata.data.Site Maven / Gradle / Ivy
/**
********************************************************************************
*** Site.java ***
*** generated by AnoSiteGenerator (ASG), Version: 4.2.2 ***
*** Copyright (C) 2005 - 2025 Anotheria.net, www.anotheria.net ***
*** All Rights Reserved. ***
********************************************************************************
*** Don't edit this code, if you aren't sure ***
*** that you do exactly know what you are doing! ***
*** It's better to invest time in the generator, as into the generated code. ***
********************************************************************************
*/
package net.anotheria.anosite.gen.assitedata.data;
import net.anotheria.asg.data.DataObject;
import java.util.List;
import net.anotheria.util.sorter.IComparable;
public interface Site extends DataObject, IComparable {
/**
* Constant property name for "id" for internal storage and queries.
*/
String PROP_ID = "id";
/**
* Constant property name for "name" for internal storage and queries.
*/
String PROP_NAME = "name";
/**
* Constant property name for "title" and domain "EN" for internal storage and queries.
*/
String PROP_TITLE_EN = "title_EN";
/**
* Constant property name for "title" and domain "DE" for internal storage and queries.
*/
String PROP_TITLE_DE = "title_DE";
/**
* Constant property name for "keywords" and domain "EN" for internal storage and queries.
*/
String PROP_KEYWORDS_EN = "keywords_EN";
/**
* Constant property name for "keywords" and domain "DE" for internal storage and queries.
*/
String PROP_KEYWORDS_DE = "keywords_DE";
/**
* Constant property name for "description" and domain "EN" for internal storage and queries.
*/
String PROP_DESCRIPTION_EN = "description_EN";
/**
* Constant property name for "description" and domain "DE" for internal storage and queries.
*/
String PROP_DESCRIPTION_DE = "description_DE";
/**
* Constant property name for "subtitle" and domain "EN" for internal storage and queries.
*/
String PROP_SUBTITLE_EN = "subtitle_EN";
/**
* Constant property name for "subtitle" and domain "DE" for internal storage and queries.
*/
String PROP_SUBTITLE_DE = "subtitle_DE";
/**
* Constant property name for "mainNavi" for internal storage and queries.
*/
String PROP_MAIN_NAVI = "mainNavi";
/**
* Constant property name for "topNavi" for internal storage and queries.
*/
String PROP_TOP_NAVI = "topNavi";
/**
* Constant property name for "languageselector" for internal storage and queries.
*/
String PROP_LANGUAGESELECTOR = "languageselector";
/**
* Constant property name for "startpage" for internal storage and queries.
*/
String LINK_PROP_STARTPAGE = "startpage";
/**
* Constant property name for "searchpage" for internal storage and queries.
*/
String LINK_PROP_SEARCHPAGE = "searchpage";
/**
* Constant property name for "headerBackground" for internal storage and queries.
*/
String LINK_PROP_HEADER_BACKGROUND = "headerBackground";
/**
* Constant property name for "siteLogo" for internal storage and queries.
*/
String LINK_PROP_SITE_LOGO = "siteLogo";
/**
* Returns the value of the name attribute.
*/
String getName();
/**
* Sets the value of the name attribute.
*/
void setName(String value);
/**
* Returns the value of the title attribute in the "EN" domain.
*/
String getTitleEN();
/**
* Returns the value of the title attribute in the "DE" domain.
*/
String getTitleDE();
/**
* Returns the current value of the title attribute.
* Current means in the currently selected domain.
*/
String getTitle();
/**
* Sets the value of the title attribute in the domain "EN"
*/
void setTitleEN(String value);
/**
* Sets the value of the title attribute in the domain "DE"
*/
void setTitleDE(String value);
/**
* Sets the value of the title attribute in the current domain. Current means in the currently selected domain.
*/
void setTitle(String value);
/**
* Returns the value of the keywords attribute in the "EN" domain.
*/
String getKeywordsEN();
/**
* Returns the value of the keywords attribute in the "DE" domain.
*/
String getKeywordsDE();
/**
* Returns the current value of the keywords attribute.
* Current means in the currently selected domain.
*/
String getKeywords();
/**
* Sets the value of the keywords attribute in the domain "EN"
*/
void setKeywordsEN(String value);
/**
* Sets the value of the keywords attribute in the domain "DE"
*/
void setKeywordsDE(String value);
/**
* Sets the value of the keywords attribute in the current domain. Current means in the currently selected domain.
*/
void setKeywords(String value);
/**
* Returns the value of the description attribute in the "EN" domain.
*/
String getDescriptionEN();
/**
* Returns the value of the description attribute in the "DE" domain.
*/
String getDescriptionDE();
/**
* Returns the current value of the description attribute.
* Current means in the currently selected domain.
*/
String getDescription();
/**
* Sets the value of the description attribute in the domain "EN"
*/
void setDescriptionEN(String value);
/**
* Sets the value of the description attribute in the domain "DE"
*/
void setDescriptionDE(String value);
/**
* Sets the value of the description attribute in the current domain. Current means in the currently selected domain.
*/
void setDescription(String value);
/**
* Returns the value of the subtitle attribute in the "EN" domain.
*/
String getSubtitleEN();
/**
* Returns the value of the subtitle attribute in the "DE" domain.
*/
String getSubtitleDE();
/**
* Returns the current value of the subtitle attribute.
* Current means in the currently selected domain.
*/
String getSubtitle();
/**
* Sets the value of the subtitle attribute in the domain "EN"
*/
void setSubtitleEN(String value);
/**
* Sets the value of the subtitle attribute in the domain "DE"
*/
void setSubtitleDE(String value);
/**
* Sets the value of the subtitle attribute in the current domain. Current means in the currently selected domain.
*/
void setSubtitle(String value);
/**
* Returns the value of the mainNavi attribute.
*/
List getMainNavi();
/**
* Sets the value of the mainNavi attribute.
*/
void setMainNavi(List value);
/**
* Returns the value of the topNavi attribute.
*/
List getTopNavi();
/**
* Sets the value of the topNavi attribute.
*/
void setTopNavi(List value);
/**
* Returns the value of the languageselector attribute.
*/
boolean getLanguageselector();
/**
* Sets the value of the languageselector attribute.
*/
void setLanguageselector(boolean value);
/**
* Returns the value of the startpage attribute.
*/
String getStartpage();
/**
* Sets the value of the startpage attribute.
*/
void setStartpage(String value);
/**
* Returns the value of the searchpage attribute.
*/
String getSearchpage();
/**
* Sets the value of the searchpage attribute.
*/
void setSearchpage(String value);
/**
* Returns the value of the headerBackground attribute.
*/
String getHeaderBackground();
/**
* Sets the value of the headerBackground attribute.
*/
void setHeaderBackground(String value);
/**
* Returns the value of the siteLogo attribute.
*/
String getSiteLogo();
/**
* Sets the value of the siteLogo attribute.
*/
void setSiteLogo(String value);
/**
* Returns the number of elements in the "mainNavi" container
*/
int getMainNaviSize();
/**
* Adds a new element to the list.
*/
void addMainNaviElement(String item);
/**
* Removes the element at position index from the list.
*/
void removeMainNaviElement(int index);
/**
* Swaps elements at positions index1 and index2 in the list.
*/
void swapMainNaviElement(int index1, int index2);
/**
* Returns the element at the position index in the list.
*/
String getMainNaviElement(int index);
/**
* Returns the number of elements in the "topNavi" container
*/
int getTopNaviSize();
/**
* Adds a new element to the list.
*/
void addTopNaviElement(String item);
/**
* Removes the element at position index from the list.
*/
void removeTopNaviElement(int index);
/**
* Swaps elements at positions index1 and index2 in the list.
*/
void swapTopNaviElement(int index1, int index2);
/**
* Returns the element at the position index in the list.
*/
String getTopNaviElement(int index);
/**
* Copies all multilingual properties from source language to destination language
*/
void copyLANG2LANG(String sourceLanguge, String destLanguage);
/**
* Copies all multilingual properties from language EN to language DE
*/
void copyEN2DE();
/**
* Copies all multilingual properties from language DE to language EN
*/
void copyDE2EN();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy