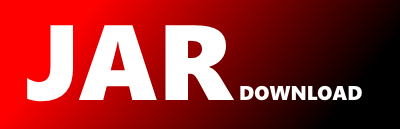
net.anotheria.anosite.gen.aswebdata.bean.EditPagexFB Maven / Gradle / Ivy
/**
********************************************************************************
*** EditPagexFB.java ***
*** generated by AnoSiteGenerator (ASG), Version: 4.2.2 ***
*** Copyright (C) 2005 - 2025 Anotheria.net, www.anotheria.net ***
*** All Rights Reserved. ***
********************************************************************************
*** Don't edit this code, if you aren't sure ***
*** that you do exactly know what you are doing! ***
*** It's better to invest time in the generator, as into the generated code. ***
********************************************************************************
*/
package net.anotheria.anosite.gen.aswebdata.bean;
import net.anotheria.maf.bean.FormBean;
import java.util.List;
import net.anotheria.asg.util.bean.LabelValueBean;
public class EditPagexFB implements FormBean{
// Generated by: class net.anotheria.asg.generator.view.action.ModuleBeanGenerator.generateDialogForm
// Generated by: class net.anotheria.asg.generator.view.action.ModuleBeanGenerator.generateDialogForm
private String id;
private String name;
private String localizedNameEN;
private String localizedNameDE;
private String titleEN;
private String titleDE;
private String keywordsEN;
private String keywordsDE;
private String descriptionEN;
private String descriptionDE;
private int mediaLinks;
private int scripts;
private int localizations;
private String template;
private List templateCollection;
private String templateCurrentValue;
private String templateIdOfCurrentValue;
private int attributes;
private int c1;
private int c2;
private int c3;
private int header;
private int footer;
private boolean httpsonly;
private String accessOperation;
private List accessOperationCollection;
private String accessOperationCurrentValue;
private String accessOperationIdOfCurrentValue;
private String feature;
private List featureCollection;
private String featureCurrentValue;
private String featureIdOfCurrentValue;
public void setId(String id ){
this.id = id;
}
public String getId(){
return id;
}
public void setName(String name ){
this.name = name;
}
public String getName(){
return name;
}
public void setLocalizedNameEN(String localizedName ){
this.localizedNameEN = localizedName;
}
public String getLocalizedNameEN(){
return localizedNameEN;
}
public void setLocalizedNameDE(String localizedName ){
this.localizedNameDE = localizedName;
}
public String getLocalizedNameDE(){
return localizedNameDE;
}
public void setTitleEN(String title ){
this.titleEN = title;
}
public String getTitleEN(){
return titleEN;
}
public void setTitleDE(String title ){
this.titleDE = title;
}
public String getTitleDE(){
return titleDE;
}
public void setKeywordsEN(String keywords ){
this.keywordsEN = keywords;
}
public String getKeywordsEN(){
return keywordsEN;
}
public void setKeywordsDE(String keywords ){
this.keywordsDE = keywords;
}
public String getKeywordsDE(){
return keywordsDE;
}
public void setDescriptionEN(String description ){
this.descriptionEN = description;
}
public String getDescriptionEN(){
return descriptionEN;
}
public void setDescriptionDE(String description ){
this.descriptionDE = description;
}
public String getDescriptionDE(){
return descriptionDE;
}
public void setMediaLinks(int mediaLinks ){
this.mediaLinks = mediaLinks;
}
public int getMediaLinks(){
return mediaLinks;
}
public void setScripts(int scripts ){
this.scripts = scripts;
}
public int getScripts(){
return scripts;
}
public void setLocalizations(int localizations ){
this.localizations = localizations;
}
public int getLocalizations(){
return localizations;
}
public void setTemplateCollection(List templateCollection ){
this.templateCollection = templateCollection;
}
public List getTemplateCollection(){
return templateCollection;
}
public void setTemplateCurrentValue(String templateCurrentValue ){
this.templateCurrentValue = templateCurrentValue;
}
public String getTemplateCurrentValue(){
return templateCurrentValue;
}
public void setTemplateIdOfCurrentValue(String templateIdOfCurrentValue ){
this.templateIdOfCurrentValue = templateIdOfCurrentValue;
}
public String getTemplateIdOfCurrentValue(){
return templateIdOfCurrentValue;
}
public void setTemplate(String template ){
this.template = template;
}
public String getTemplate(){
return template;
}
public void setAttributes(int attributes ){
this.attributes = attributes;
}
public int getAttributes(){
return attributes;
}
public void setC1(int c1 ){
this.c1 = c1;
}
public int getC1(){
return c1;
}
public void setC2(int c2 ){
this.c2 = c2;
}
public int getC2(){
return c2;
}
public void setC3(int c3 ){
this.c3 = c3;
}
public int getC3(){
return c3;
}
public void setHeader(int header ){
this.header = header;
}
public int getHeader(){
return header;
}
public void setFooter(int footer ){
this.footer = footer;
}
public int getFooter(){
return footer;
}
public void setHttpsonly(boolean httpsonly ){
this.httpsonly = httpsonly;
}
public boolean isHttpsonly(){
return httpsonly;
}
public void setAccessOperationCollection(List accessOperationCollection ){
this.accessOperationCollection = accessOperationCollection;
}
public List getAccessOperationCollection(){
return accessOperationCollection;
}
public void setAccessOperationCurrentValue(String accessOperationCurrentValue ){
this.accessOperationCurrentValue = accessOperationCurrentValue;
}
public String getAccessOperationCurrentValue(){
return accessOperationCurrentValue;
}
public void setAccessOperationIdOfCurrentValue(String accessOperationIdOfCurrentValue ){
this.accessOperationIdOfCurrentValue = accessOperationIdOfCurrentValue;
}
public String getAccessOperationIdOfCurrentValue(){
return accessOperationIdOfCurrentValue;
}
public void setAccessOperation(String accessOperation ){
this.accessOperation = accessOperation;
}
public String getAccessOperation(){
return accessOperation;
}
public void setFeatureCollection(List featureCollection ){
this.featureCollection = featureCollection;
}
public List getFeatureCollection(){
return featureCollection;
}
public void setFeatureCurrentValue(String featureCurrentValue ){
this.featureCurrentValue = featureCurrentValue;
}
public String getFeatureCurrentValue(){
return featureCurrentValue;
}
public void setFeatureIdOfCurrentValue(String featureIdOfCurrentValue ){
this.featureIdOfCurrentValue = featureIdOfCurrentValue;
}
public String getFeatureIdOfCurrentValue(){
return featureIdOfCurrentValue;
}
public void setFeature(String feature ){
this.feature = feature;
}
public String getFeature(){
return feature;
}
private boolean multilingualInstanceDisabled;
public void setMultilingualInstanceDisabled(boolean multilingualInstanceDisabled ){
this.multilingualInstanceDisabled = multilingualInstanceDisabled;
}
public boolean isMultilingualInstanceDisabled(){
return multilingualInstanceDisabled;
}
/**
* LockableObject "locked" property. For object Locking.
*/
private boolean locked;
public void setLocked(boolean locked ){
this.locked = locked;
}
public boolean isLocked(){
return locked;
}
/**
* LockableObject "lockerId" property. For userName containing.
*/
private String lockerId;
public void setLockerId(String lockerId ){
this.lockerId = lockerId;
}
public String getLockerId(){
return lockerId;
}
/**
* LockableObject "lockingTime" property.
*/
private String lockingTime;
public void setLockingTime(String lockingTime ){
this.lockingTime = lockingTime;
}
public String getLockingTime(){
return lockingTime;
}
/**
* Link to template
*/
private String templatelink;
public void setTemplatelink(String templatelink ){
this.templatelink = templatelink;
}
public String getTemplatelink(){
return templatelink;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy