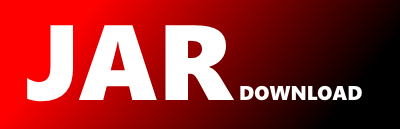
net.anotheria.util.datatable.DataTable Maven / Gradle / Ivy
package net.anotheria.util.datatable;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
/**
* This class represents a table with untyped data, similar to a csv file.
*
* @author lrosenberg
* @version $Id: $Id
*/
public class DataTable implements Iterable {
/**
* The header of the table.
*/
private DataHeader header;
/**
* A list with rows.
*/
private List rows;
/**
* Constructor for DataTable.
*/
public DataTable(){
this(10);
}
/**
* Constructor for DataTable.
*
* @param aInitialCapacity a int.
*/
public DataTable(int aInitialCapacity){
rows = new ArrayList<>(aInitialCapacity);
}
/**
* Getter for the field header
.
*
* @return a {@link net.anotheria.util.datatable.DataHeader} object.
*/
public DataHeader getHeader() {
return header;
}
/**
* Setter for the field header
.
*
* @param aHeader a {@link net.anotheria.util.datatable.DataHeader} object.
*/
public void setHeader(DataHeader aHeader) {
this.header = aHeader;
}
/**
* getRow.
*
* @param aIndex a int.
* @return a {@link net.anotheria.util.datatable.DataRow} object.
*/
public DataRow getRow(int aIndex){
return rows.get(aIndex);
}
/**
* setRow.
*
* @param aIndex a int.
* @param aRow a {@link net.anotheria.util.datatable.DataRow} object.
*/
public void setRow(int aIndex, DataRow aRow){
rows.set(aIndex, aRow);
}
/**
* addRow.
*
* @param aRow a {@link net.anotheria.util.datatable.DataRow} object.
*/
public void addRow(DataRow aRow){
rows.add(aRow);
}
/**
* removeRow.
*
* @param aIndex a int.
*/
public void removeRow(int aIndex){
rows.remove(aIndex);
}
/**
* removeRow.
*
* @param aRow a {@link net.anotheria.util.datatable.DataRow} object.
*/
public void removeRow(DataRow aRow){
rows.remove(aRow);
}
/**
* getRowIndex.
*
* @param aRow a {@link net.anotheria.util.datatable.DataRow} object.
* @return a int.
*/
public int getRowIndex(DataRow aRow){
return rows.indexOf(aRow);
}
/**
* getRowsSize.
*
* @return a int.
*/
public int getRowsSize(){
return rows.size();
}
/** {@inheritDoc} */
@Override
public Iterator iterator() {
return rows.iterator();
}
/** {@inheritDoc} */
@Override
public String toString(){
String ret = "";
ret += "HEADER:";
ret += "\n";
ret += header.toString();
ret += "\n";
ret += "ROWS:";
ret += "\n";
for(DataRow r: this){
ret += r.toString();
ret += "\n";
}
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy