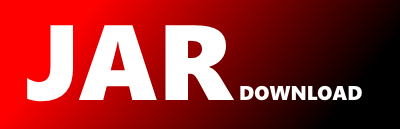
net.anotheria.util.xml.XMLNode Maven / Gradle / Ivy
package net.anotheria.util.xml;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.OutputStreamWriter;
import java.io.PrintStream;
import java.io.PrintWriter;
import java.io.Writer;
import java.util.ArrayList;
import java.util.List;
/**
* XMLNode. Actually 'Anotheria' custom XML representation.
*
* @author another
* @version $Id: $Id
*/
public class XMLNode {
private static final Logger log = LoggerFactory.getLogger(XMLNode.class);
/**
* SubNodes of this node.
*/
private List nodes;
/**
* Attributes of the node.
*/
private List attributes;
/**
* The name of the node.
*/
private String name;
/**
* The content of the node.
*/
private String content;
/**
* Constructor. Creates a new XMLNode.
*
* @param aName node name
*/
public XMLNode(String aName){
name = aName;
attributes = new ArrayList<>();
nodes = new ArrayList<>();
}
/**
* Getter for the field nodes
.
*
* @return a {@link java.util.List} object.
*/
public List getNodes() {
return nodes;
}
/**
* Set child nodes for current.
*
* @param aChildren xmlNodes list
*/
public void setChildren(List aChildren){
this.nodes = aChildren;
}
/**
* Setter for the field nodes
.
*
* @param aNodes a {@link java.util.List} object.
*/
public void setNodes(List aNodes) {
this.nodes = aNodes;
}
/**
* Allow add child node for current.
*
* @param aChild xmlNode
*/
public void addChildNode(XMLNode aChild){
nodes.add(aChild);
}
/**
* Getter for the field attributes
.
*
* @return a {@link java.util.List} object.
*/
public List getAttributes() {
return attributes;
}
/**
* Setter for the field attributes
.
*
* @param aAttributes a {@link java.util.List} object.
*/
public void setAttributes(List aAttributes) {
this.attributes = aAttributes;
}
/**
* addAttribute.
*
* @param aAttribute a {@link net.anotheria.util.xml.XMLAttribute} object.
*/
public void addAttribute(XMLAttribute aAttribute){
attributes.add(aAttribute);
}
/**
* Getter for the field name
.
*
* @return a {@link java.lang.String} object.
*/
public String getName() {
return name;
}
/**
* Setter for the field name
.
*
* @param aName a {@link java.lang.String} object.
*/
public void setName(String aName) {
this.name = aName;
}
/**
* Getter for the field content
.
*
* @return a {@link java.lang.String} object.
*/
public String getContent() {
return content;
}
/**
* Setter for the field content
.
*
* @param aContent a {@link java.lang.String} object.
*/
public void setContent(String aContent) {
this.content = aContent;
}
/**
* Setter for the field content
.
*
* @param aContent a boolean.
*/
public void setContent(boolean aContent){
this.content = String.valueOf(aContent);
}
/**
* Setter for the field content
.
*
* @param aContent a float.
*/
public void setContent(float aContent){
this.content = String.valueOf(aContent);
}
/**
* Setter for the field content
.
*
* @param aContent a double.
*/
public void setContent(double aContent){
this.content = String.valueOf(aContent);
}
/**
* Setter for the field content
.
*
* @param aContent a long.
*/
public void setContent(long aContent){
this.content = String.valueOf(aContent);
}
/**
* Setter for the field content
.
*
* @param aContent a int.
*/
public void setContent(int aContent){
this.content = String.valueOf(aContent);
}
/**
* Setter for the field content
.
*
* @param aL a {@link java.util.List} object.
*/
public void setContent(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy