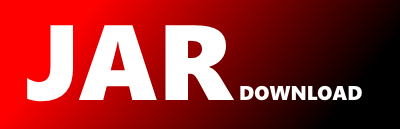
org.distributeme.goldminer.generated.AsynchGoldMinerServiceStub Maven / Gradle / Ivy
package org.distributeme.goldminer.generated;
//CHECKSTYLE:OFF
import java.util.List;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.concurrent.ConcurrentMap;
import java.util.concurrent.ConcurrentHashMap;
import org.slf4j.Logger;
import org.distributeme.goldminer.GoldMinerService;
import java.lang.InterruptedException;
import org.distributeme.core.exception.DistributemeRuntimeException;
import org.distributeme.core.exception.NoConnectionToServerException;
import org.distributeme.core.exception.ServiceUnavailableException;
import org.distributeme.core.Defaults;
import org.distributeme.core.asynch.CallBackHandler;
import org.distributeme.core.asynch.SingleCallHandler;
import org.distributeme.core.asynch.CallTimeoutedException;
import java.lang.IllegalStateException;
import org.distributeme.core.ServiceLocator;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.atomic.AtomicLong;
public class AsynchGoldMinerServiceStub implements AsynchGoldMinerService{
private final GoldMinerService diMeTarget;
private final ExecutorService diMeExecutor;
private final AtomicLong diMeRequestCounter = new AtomicLong();
public AsynchGoldMinerServiceStub(){
this(ServiceLocator.getRemote(GoldMinerService.class));
}
public AsynchGoldMinerServiceStub(GoldMinerService aTarget){
diMeTarget = aTarget;
diMeExecutor = Executors.newFixedThreadPool(Defaults.getAsynchExecutorPoolSize());
}
public boolean searchForGold(){
SingleCallHandler diMeCallHandler = new SingleCallHandler();
asynchSearchForGold(diMeCallHandler);
try{
diMeCallHandler.waitForResults(2500);
}catch(InterruptedException e){
}
if (!diMeCallHandler.isFinished())
throw new CallTimeoutedException();
if (diMeCallHandler.isSuccess())
return ((Boolean)diMeCallHandler.getReturnValue()).booleanValue();
if (diMeCallHandler.isError()){
Exception exceptionInMethod = diMeCallHandler.getReturnException();
if (exceptionInMethod instanceof RuntimeException)
throw (RuntimeException)exceptionInMethod;
throw new RuntimeException("Shouldn't happen, unexpected exception of class "+exceptionInMethod.getClass().getName()+" thrown by method", exceptionInMethod);
} //...if isError
throw new IllegalStateException("You can't be here ;-)");
} //...boolean searchForGold()
public void asynchSearchForGold(final CallBackHandler... diMeHandlers){
diMeExecutor.execute(new Runnable() {
@Override
public void run() {
long diMeRequestNumber = diMeRequestCounter.incrementAndGet();
try{
// make the real call here
boolean diMeReturnValue = diMeTarget.searchForGold();
if (diMeHandlers!=null){
for (CallBackHandler diMeHandler : diMeHandlers){
try{
diMeHandler.success(diMeReturnValue);
}catch(Exception ignored){
// add exception warn here
}
} //...for
} //...if
}catch(Exception e){
if (diMeHandlers!=null){
for (CallBackHandler diMeHandler : diMeHandlers){
try{
diMeHandler.error(e);
}catch(Exception ignored){
// add exception warn here
}
} //...for
} //...if
} //...catch
}
});
} //...public void asynchSearchForGold(final CallBackHandler... diMeHandlers)
public int washGold(long duration){
SingleCallHandler diMeCallHandler = new SingleCallHandler();
asynchWashGold(duration, diMeCallHandler);
try{
diMeCallHandler.waitForResults(2500);
}catch(InterruptedException e){
}
if (!diMeCallHandler.isFinished())
throw new CallTimeoutedException();
if (diMeCallHandler.isSuccess())
return ((Integer)diMeCallHandler.getReturnValue()).intValue();
if (diMeCallHandler.isError()){
Exception exceptionInMethod = diMeCallHandler.getReturnException();
if (exceptionInMethod instanceof RuntimeException)
throw (RuntimeException)exceptionInMethod;
throw new RuntimeException("Shouldn't happen, unexpected exception of class "+exceptionInMethod.getClass().getName()+" thrown by method", exceptionInMethod);
} //...if isError
throw new IllegalStateException("You can't be here ;-)");
} //...int washGold(long duration)
public void asynchWashGold(final long duration, final CallBackHandler... diMeHandlers){
diMeExecutor.execute(new Runnable() {
@Override
public void run() {
long diMeRequestNumber = diMeRequestCounter.incrementAndGet();
try{
// make the real call here
int diMeReturnValue = diMeTarget.washGold(duration);
if (diMeHandlers!=null){
for (CallBackHandler diMeHandler : diMeHandlers){
try{
diMeHandler.success(diMeReturnValue);
}catch(Exception ignored){
// add exception warn here
}
} //...for
} //...if
}catch(Exception e){
if (diMeHandlers!=null){
for (CallBackHandler diMeHandler : diMeHandlers){
try{
diMeHandler.error(e);
}catch(Exception ignored){
// add exception warn here
}
} //...for
} //...if
} //...catch
}
});
} //...public void asynchWashGold(final long duration, final CallBackHandler... diMeHandlers)
public void shutdown(){
diMeExecutor.shutdown();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy