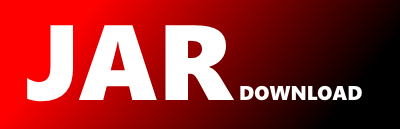
org.distributeme.test.list.ListServiceImpl Maven / Gradle / Ivy
package org.distributeme.test.list;
import org.distributeme.core.routing.RoutingAware;
import org.distributeme.core.util.ServerSideUtils;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
/**
* TODO comment this class
*
* @author lrosenberg
* @since 22.09.15 23:44
*/
public class ListServiceImpl implements ListService, RoutingAware{
private HashMap objects;
public ListServiceImpl(){
objects = new HashMap();
for (int i=0; i<100;i++){
objects.put(new ListObjectId(i), new ListObject(i));
}
}
@Override
public ListObject getListObject(ListObjectId id) {
System.out.println(failoverInfoString()+"%%% Called getListObject("+id+")");
return objects.get(id);
}
@Override
public Collection getListObjects() {
System.out.println(failoverInfoString()+"%%% Called getListObjects()");
ArrayList ret = new ArrayList();
Collection all = objects.values();
ret.addAll(all);
return ret;
}
@Override
public Collection getSomeListObjects(Collection ids) {
System.out.println(failoverInfoString()+"%%% Called getListObjects() with "+ids.size()+" params, "+ids);
ArrayList ret = new ArrayList();
for (ListObjectId id : ids){
ret.add(objects.get(id));
}
System.out.println("%%% Returning " + ret);
return ret;
}
@Override
public Collection getListObjectsSharded() {
System.out.println(failoverInfoString()+"%%% Called getListObjectsSharded()");
return null;
}
@Override
public Collection getSomeListObjectsSharded(Collection ids) {
System.out.println(failoverInfoString()+"%%% Called getSomeListObjectsSharded() with "+ids.size()+" params, "+ids);
return null;
}
private String failoverInfoString(){
return isFailoverCall() ? "FAILOVER " +
(ServerSideUtils.isBlacklisted() ? "-BLACKLIST-" : "")
+ " - "
: "";
}
private boolean isFailoverCall(){
return ServerSideUtils.isFailoverCall();
}
@Override
public void notifyServiceId(String definedServiceId, String registeredAsServiceId, String routingParameter, String configurationName) {
System.out.println("I am "+definedServiceId+" registered as "+registeredAsServiceId);
System.out.println("My Routing Param is "+routingParameter+", myConfig name "+configurationName);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy