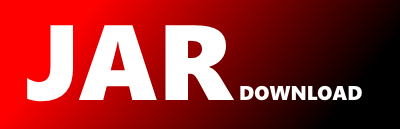
net.anotheria.moskito.webui.journey.api.JourneyAPIImpl Maven / Gradle / Ivy
package net.anotheria.moskito.webui.journey.api;
import net.anotheria.anoplass.api.APIException;
import net.anotheria.anoplass.api.APIInitException;
import net.anotheria.moskito.core.calltrace.CurrentlyTracedCall;
import net.anotheria.moskito.core.calltrace.TraceStep;
import net.anotheria.moskito.core.journey.Journey;
import net.anotheria.moskito.core.journey.JourneyManager;
import net.anotheria.moskito.core.journey.JourneyManagerFactory;
import net.anotheria.moskito.core.journey.NoSuchJourneyException;
import net.anotheria.moskito.core.stats.TimeUnit;
import net.anotheria.moskito.webui.shared.api.AbstractMoskitoAPIImpl;
import net.anotheria.util.NumberUtils;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* TODO comment this class
*
* @author lrosenberg
* @since 14.02.13 10:00
*/
public class JourneyAPIImpl extends AbstractMoskitoAPIImpl implements JourneyAPI {
private JourneyManager journeyManager;
@Override
public void init() throws APIInitException {
super.init();
journeyManager = JourneyManagerFactory.getJourneyManager();
}
@Override
public List getJourneys() throws APIException {
List journeys = journeyManager.getJourneys();
List beans = new ArrayList(journeys.size());
for (Journey j : journeys){
JourneyListItemAO bean = new JourneyListItemAO();
bean.setName(j.getName());
bean.setActive(j.isActive());
bean.setCreated(NumberUtils.makeISO8601TimestampString(j.getCreatedTimestamp()));
bean.setLastActivity(NumberUtils.makeISO8601TimestampString(j.getLastActivityTimestamp()));
bean.setNumberOfCalls(j.getTracedCalls().size());
beans.add(bean);
}
return beans;
}
@Override
public JourneyAO getJourney(String name) throws APIException {
JourneyAO ret = new JourneyAO();
Journey journey;
try{
journey = journeyManager.getJourney(name);
}catch(NoSuchJourneyException e){
throw new APIException("Journey not found.");
}
ret.setName(journey.getName());
ret.setActive(journey.isActive());
ret.setCreatedTimestamp(journey.getCreatedTimestamp());
ret.setLastActivityTimestamp(journey.getLastActivityTimestamp());
List recorded = journey.getTracedCalls();
List calls = new ArrayList(recorded.size());
for (int i=0; i tags = useCase.getTags();
if (tags!=null && tags.size()>0){
for (Map.Entry entry : tags.entrySet()){
TagAO tagAO = new TagAO(entry.getKey(), entry.getValue());
ret.getTags().add(tagAO);
}
}
JourneyCallIntermediateContainer container = new JourneyCallIntermediateContainer();
fillUseCasePathElementBeanList(container, root,0, unit);
ret.setElements(container.getElements());
//check for duplicates
List dupSteps = new ArrayList<>();
Map stepsReversed = container.getReversedSteps();
for (Map.Entry entry : stepsReversed.entrySet()){
if (entry.getValue()!=null && entry.getValue().getPositions().size()>1){
//duplicate found
TracedCallDuplicateStepsAO dupStep = new TracedCallDuplicateStepsAO();
dupStep.setCall(entry.getKey());
dupStep.setPositions(entry.getValue().getPositions());
dupStep.setTimespent(entry.getValue().getTimespent());
dupStep.setDuration(entry.getValue().getDuration());
dupSteps.add(dupStep);
}
}
ret.setDuplicateSteps(dupSteps);
return ret;
}
@Override
public TracedCallAO getTracedCallByName(String journeyName, String traceName, TimeUnit unit) throws APIException{
Journey journey = getJourneyByName(journeyName);
CurrentlyTracedCall call = journey.getStepByName(traceName);
try {
return mapTracedCall(call, unit);
}catch(IllegalArgumentException e){
throw new IllegalStateException("Can' lookup call by name: "+journeyName+", "+traceName+", "+unit);
}
}
@Override
public TracedCallAO getTracedCall(String journeyName, int callPosition, TimeUnit unit) throws APIException {
Journey journey = getJourneyByName(journeyName);
CurrentlyTracedCall call = journey.getTracedCalls().get(callPosition);
return mapTracedCall(call, unit);
}
private void fillUseCasePathElementBeanList(JourneyCallIntermediateContainer container, TraceStep element, int recursion, TimeUnit unit){
TracedCallStepAO b = new TracedCallStepAO();
b.setCall(recursion == 0 ? "ROOT" : element.getCall());
b.setRoot(recursion == 0);
b.setLayer(recursion);
b.setDuration(unit.transformNanos(element.getDuration()));
b.setLevel(recursion);
StringBuilder ident = new StringBuilder();
for (int i=0; i analyzeJourney(String journeyName) throws APIException {
Journey journey = getJourneyByName(journeyName);
List tracedCalls = journey.getTracedCalls();
List callsList = new ArrayList<>(tracedCalls.size() + 1);
AnalyzedProducerCallsMapAO overallCallsMap = new AnalyzedProducerCallsMapAO(journey.getName()+" - TOTAL");
callsList.add(overallCallsMap);
for (CurrentlyTracedCall tc : tracedCalls){
if (tc==null){
log.warn("TracedCall is null!");
continue;
}
AnalyzedProducerCallsMapAO singleCallMap = new AnalyzedProducerCallsMapAO(tc.getName());
for (TraceStep step : tc.getRootStep().getChildren()){
addStep(step, singleCallMap, overallCallsMap);
}
callsList.add(singleCallMap);
}
return callsList;
}
private Journey getJourneyByName(String name) throws APIException{
Journey journey = null;
try{
journey = journeyManager.getJourney(name);
return journey;
}catch(NoSuchJourneyException e){
throw new APIException("Journey " + name+ " not found.");
}
}
private void addStep(TraceStep step, AnalyzedProducerCallsMapAO... maps){
String producerName = step.getProducer() == null ?
"UNKNOWN" : step.getProducer().getProducerId();
for (AnalyzedProducerCallsMapAO map : maps){
map.addProducerCall(producerName, step.getNetDuration());
}
for (TraceStep childStep : step.getChildren()){
addStep(childStep, maps);
}
}
@Override
public void deleteJourney(String journeyName) throws APIException {
journeyManager.removeJourney(journeyName);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy