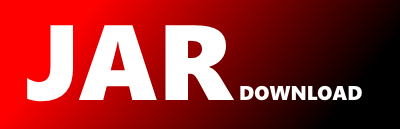
net.anthavio.cache.CacheLoadRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hatatitla Show documentation
Show all versions of hatatitla Show documentation
Compact but tweakable REST client library you have been dreaming of
The newest version!
package net.anthavio.cache;
import java.util.concurrent.TimeUnit;
import net.anthavio.cache.Builders.CacheRequestLoaderBuilder;
/**
*
* @author martin.vanek
*
*/
public class CacheLoadRequest {
public static CacheRequestLoaderBuilder With(CacheEntryLoader loader) {
return new CacheRequestLoaderBuilder(loader);
}
protected final CachingSettings caching;
protected final LoadingSettings loading;
/**
* All field constructor
*/
public CacheLoadRequest(K userKey, long hardTtl, long softTtl, TimeUnit unit, CacheEntryLoader loader,
boolean missingAsyncLoad, boolean expiredAsyncLoad) {
this.caching = new CachingSettings(userKey, hardTtl, softTtl, unit);
this.loading = new LoadingSettings(loader, missingAsyncLoad, expiredAsyncLoad);
}
public CacheLoadRequest(CachingSettings caching, LoadingSettings loading) {
if (caching == null) {
throw new IllegalArgumentException("Null caching settings");
}
this.caching = caching;
if (loading == null) {
throw new IllegalArgumentException("Null loading settings");
}
this.loading = loading;
}
public CachingSettings getCaching() {
return caching;
}
public LoadingSettings getLoading() {
return loading;
}
public K getUserKey() {
return caching.getUserKey();
}
public long getHardTtl() {
return caching.getHardTtl();
}
public long getSoftTtl() {
return caching.getSoftTtl();
}
public CacheEntryLoader getLoader() {
return loading.getLoader();
}
public boolean isExpiredLoadAsync() {
return loading.isExpiredLoadAsync();
}
public boolean isMissingLoadAsync() {
return loading.isMissingLoadAsync();
}
@Override
public String toString() {
return "CacheLoadRequest key=" + getUserKey();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy