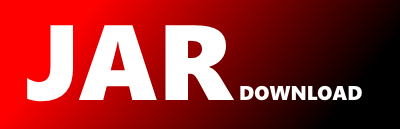
net.antidot.semantic.rdf.rdb2rdf.r2rml.model.StdPredicateObjectMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of db2triples Show documentation
Show all versions of db2triples Show documentation
RDB2RDF Implementation from Antidot
/*
* Copyright 2011 Antidot [email protected]
* https://github.com/antidot/db2triples
*
* DB2Triples is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License as
* published by the Free Software Foundation; either version 2 of
* the License, or (at your option) any later version.
*
* DB2Triples is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/***************************************************************************
*
* R2RML Model : Standard PredicateObjectMap Class
*
* A predicate-object map is a function
* that creates predicate-object pairs from logical
* table rows. It is used in conjunction with a subject
* map to generate RDF triples in a triples map.
*
****************************************************************************/
package net.antidot.semantic.rdf.rdb2rdf.r2rml.model;
import java.util.HashSet;
import java.util.Set;
public class StdPredicateObjectMap implements PredicateObjectMap {
private Set objectMaps;
private Set refObjectMaps;
private Set predicateMaps;
protected TriplesMap ownTriplesMap;
private HashSet graphMaps;
private StdPredicateObjectMap(Set predicateMaps) {
setPredicateMaps(predicateMaps);
}
public StdPredicateObjectMap(Set predicateMaps,
Set objectMaps) {
this(predicateMaps);
setObjectMaps(objectMaps);
}
public StdPredicateObjectMap(Set predicateMaps,
Set objectMaps, Set referencingObjectMaps) {
this(predicateMaps, objectMaps);
setReferencingObjectMap(referencingObjectMaps);
}
public void setReferencingObjectMap(Set refObjectMaps) {
if (refObjectMaps == null)
this.refObjectMaps = new HashSet();
else {
for (ReferencingObjectMap refObjectMap : refObjectMaps) {
if (refObjectMap != null)
refObjectMap.setPredicateObjectMap(this);
}
this.refObjectMaps = refObjectMaps;
}
}
public Set getObjectMaps() {
return objectMaps;
}
public Set getPredicateMaps() {
return predicateMaps;
}
public Set getReferencingObjectMaps() {
return refObjectMaps;
}
public boolean hasReferencingObjectMaps() {
return refObjectMaps != null && !refObjectMaps.isEmpty();
}
public TriplesMap getOwnTriplesMap() {
return ownTriplesMap;
}
public void setObjectMaps(Set objectMaps) {
if (objectMaps == null)
this.objectMaps = new HashSet();
else {
for (ObjectMap objectMap : objectMaps) {
if (objectMap != null)
objectMap.setPredicateObjectMap(this);
}
this.objectMaps = objectMaps;
}
}
public void setOwnTriplesMap(TriplesMap ownTriplesMap) {
// Update triples map if not contains this subject map
if (ownTriplesMap.getSubjectMap() != null)
if (!ownTriplesMap.getPredicateObjectMaps().contains(this))
ownTriplesMap.addPredicateObjectMap(this);
this.ownTriplesMap = ownTriplesMap;
}
public void setPredicateMaps(Set predicateMaps) {
if (predicateMaps == null)
this.predicateMaps = new HashSet();
else {
for (PredicateMap predicateMap : predicateMaps) {
if (predicateMap != null)
predicateMap.setPredicateObjectMap(this);
}
this.predicateMaps = predicateMaps;
}
}
public Set getGraphMaps() {
return graphMaps;
}
public void setGraphMaps(Set graphMaps) {
this.graphMaps = new HashSet(graphMaps);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy