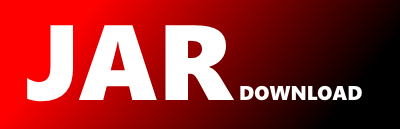
net.anwiba.commons.json.AbstractJsonObjectsUnmarshaller Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anwiba-commons-advanced Show documentation
Show all versions of anwiba-commons-advanced Show documentation
anwiba commons advanced library project
/*
* #%L anwiba commons advanced %% Copyright (C) 2007 - 2016 Andreas Bartels %% This program is free
* software: you can redistribute it and/or modify it under the terms of the GNU Lesser General
* Public License as published by the Free Software Foundation, either version 2.1 of the License,
* or (at your option) any later version. This program is distributed in the hope that it will be
* useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Lesser Public License for more details. You should
* have received a copy of the GNU General Lesser Public License along with this program. If not,
* see . #L%
*/
package net.anwiba.commons.json;
import java.io.IOException;
import java.io.InputStream;
import java.text.MessageFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import net.anwiba.commons.resource.utilities.IoUtilities;
import com.fasterxml.jackson.core.JsonFactory;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.JsonToken;
import com.fasterxml.jackson.databind.InjectableValues;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.ObjectReader;
public abstract class AbstractJsonObjectsUnmarshaller {
private final ObjectMapper mapper = new ObjectMapper();
private final Class errorResponseClass;
private final IJsonObjectMarshallingExceptionFactory exceptionFactory;
private final Class clazz;
@SuppressWarnings("rawtypes")
private final Map injectionValues = new HashMap<>();
public AbstractJsonObjectsUnmarshaller(
final Class clazz,
final Class errorResponseClass,
@SuppressWarnings("rawtypes") final Map injectionValues,
final IJsonObjectMarshallingExceptionFactory exceptionFactory) {
this.clazz = clazz;
this.errorResponseClass = errorResponseClass;
this.injectionValues.putAll(injectionValues);
this.exceptionFactory = exceptionFactory;
this.mapper.getFactory().configure(JsonParser.Feature.ALLOW_NON_NUMERIC_NUMBERS, true);
}
public List unmarshal(final InputStream inputStream) throws IOException, E {
return unmarshal(IoUtilities.toString(inputStream, "UTF-8")); //$NON-NLS-1$
}
public List unmarshal(final String body) throws IOException, E {
final T result = validate(body);
if (result != null) {
return Arrays.asList(result);
}
try {
final InjectableValues.Std injectableValues = new InjectableValues.Std();
for (@SuppressWarnings("rawtypes")
final Class key : this.injectionValues.keySet()) {
injectableValues.addValue(key, this.injectionValues.get(key));
}
final JsonFactory f = new JsonFactory();
try (final JsonParser parser = f.createParser(body)) {
final ObjectReader reader = this.mapper.readerFor(this.clazz).with(injectableValues);
final JsonToken token = parser.nextToken();
if (token != JsonToken.START_ARRAY) {
return reader.readValue(parser);
}
// and then each time, advance to opening START_OBJECT
final List results = new ArrayList<>();
while (parser.nextToken() == JsonToken.START_OBJECT) {
final T value = reader.readValue(parser);
results.add(value);
}
return results;
}
} catch (final JsonParseException exception) {
throw createIOException(body, exception);
} catch (final JsonMappingException exception) {
throw createIOException(body, exception);
}
}
private X check(final String body, final Class type)
throws IOException,
JsonParseException,
JsonMappingException,
JsonProcessingException {
final InjectableValues.Std injectableValues = new InjectableValues.Std();
for (@SuppressWarnings("rawtypes")
final Class key : this.injectionValues.keySet()) {
injectableValues.addValue(key, this.injectionValues.get(key));
}
return this.mapper.readerFor(type).with(injectableValues).readValue(body);
}
@SuppressWarnings("unchecked")
private T validate(final String body) throws IOException, E {
if (Void.class.equals(this.errorResponseClass)) {
return null;
}
try {
final R response = check(body, this.errorResponseClass);
if (this.clazz.isInstance(response)) {
return (T) response;
}
throw this.exceptionFactory.create(response);
} catch (final JsonParseException e) {
return null;
} catch (final JsonMappingException e) {
return null;
}
}
private static IOException createIOException(final String content, final Exception exception) {
return new IOException(MessageFormat.format(
"Error during mapping json resource, coudn''t map the content:\n {0}", content), //$NON-NLS-1$
exception);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy