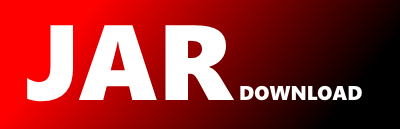
net.anwiba.commons.json.schema.v1_0.Property Maven / Gradle / Ivy
//Copyright (c) 2016 by Andreas W. Bartels ([email protected])
package net.anwiba.commons.json.schema.v1_0;
import java.lang.reflect.InvocationTargetException;
import java.text.MessageFormat;
import java.util.HashMap;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import net.anwiba.commons.reflection.ReflectionConstructorInvoker;
import net.anwiba.commons.utilities.string.StringUtilities;
public class Property {
private String name = null;
private String title = null;
private String description = null;
private final String type = "property";
private final static HashMap _classes = new HashMap();
@JsonProperty("name")
public void setName(final String name) {
this.name = name;
}
@JsonProperty("name")
public String getName() {
return this.name;
}
@JsonProperty("title")
public void setTitle(final String title) {
this.title = title;
}
@JsonProperty("title")
public String getTitle() {
return this.title;
}
@JsonProperty("description")
public void setDescription(final String description) {
this.description = description;
}
@JsonProperty("description")
public String getDescription() {
return this.description;
}
@JsonProperty("type")
public String getType() {
return this.type;
}
@JsonCreator
public static Property create(
@JsonProperty("type")
String type) {
if (StringUtilities.isNullOrTrimmedEmpty(type)) {
return new Property();
}
Class extends Property> clazz = _createClass(type);
if (clazz!= null) {
return _createBean(clazz);
}
clazz = _createClass(type.toLowerCase());
if (clazz!= null) {
return _createBean(clazz);
}
String className = MessageFormat.format("{0}{1}", type, "Property");
clazz = _createClass(className);
if (clazz!= null) {
return _createBean(clazz);
}
className = MessageFormat.format("{0}{1}", type.toLowerCase(), "Property");
clazz = _createClass(className);
if (clazz!= null) {
return _createBean(clazz);
}
return new Property();
}
private static Property _createBean(Class extends Property> clazz) {
try {
ReflectionConstructorInvoker invoker = new ReflectionConstructorInvoker(clazz);
return invoker.invoke();
} catch (InvocationTargetException exception) {
throw new RuntimeException(exception);
}
}
private static synchronized Class extends Property> _createClass(String type) {
if (_classes.containsKey(type)) {
return ((Class extends Property> ) _classes.get(type));
}
try {
String packageName = Property.class.getPackage().getName();
String typeName = StringUtilities.setFirstTrimedCharacterToUpperCase(type);
String className = MessageFormat.format("{0}.{1}", packageName, typeName);
Class> clazz = Class.forName(className);
if (!Property.class.isAssignableFrom(clazz)) {
_classes.put(type, null);
return null;
}
_classes.put(type, clazz);
return ((Class extends Property> ) clazz);
} catch (ClassNotFoundException exception) {
_classes.put(type, null);
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy