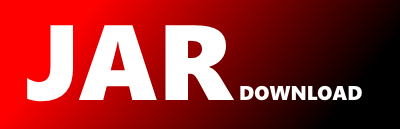
net.anwiba.commons.ensure.Conditions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anwiba-commons-core Show documentation
Show all versions of anwiba-commons-core Show documentation
anwiba commons core library project
/*
* #%L
* anwiba commons core
* %%
* Copyright (C) 2007 - 2016 Andreas W. Bartels ([email protected])
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 2.1 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package net.anwiba.commons.ensure;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
public class Conditions {
public static ICondition equalTo(final T value) {
return new EqualToCondition<>(value);
}
public static ICondition notNull() {
return new NotCondition<>(new IsNullCondition());
}
public static ICondition isNull() {
return new IsNullCondition<>();
}
public static ICondition isTrue() {
return new IsTrueCondition();
}
public static ICondition isFalse() {
return new NotCondition<>(new IsTrueCondition());
}
@SafeVarargs
public static ICondition in(final T... values) {
return in(Arrays.asList(values));
}
public static ICondition in(final Collection collection) {
return new InCondition<>(collection == null ? new ArrayList() : collection);
}
public static ICondition not(final ICondition validation) {
Ensure.ensureArgumentNotNull(validation);
return new NotCondition<>(validation);
}
public static ICondition
© 2015 - 2025 Weber Informatics LLC | Privacy Policy