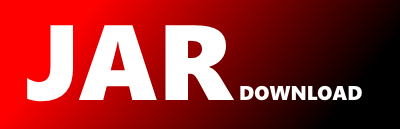
net.anwiba.commons.utilities.collection.IterableUtilities Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anwiba-commons-core Show documentation
Show all versions of anwiba-commons-core Show documentation
anwiba commons core library project
/*
* #%L
* anwiba commons core
* %%
* Copyright (C) 2007 - 2016 Andreas Bartels
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 2.1 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package net.anwiba.commons.utilities.collection;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import net.anwiba.commons.lang.functional.IAcceptor;
import net.anwiba.commons.lang.functional.IConverter;
import net.anwiba.commons.utilities.ArrayUtilities;
public class IterableUtilities {
public static Set asSet(final Iterable iterable) {
final Set result = new HashSet<>();
for (final T item : iterable) {
result.add(item);
}
return result;
}
public static Set asSet(final Iterable iterable, final IAcceptor validator) {
final Set result = new HashSet<>();
for (final T item : iterable) {
if (validator.accept(item)) {
result.add(item);
}
}
return result;
}
public static Iterable reverse(final Iterable iterable) {
final List list = asList(iterable);
Collections.reverse(list);
return list;
}
public static T[] toArray(final Iterable iterable, final Class clazz) {
final List list = asList(iterable);
@SuppressWarnings("unchecked")
final T[] array = (T[]) Array.newInstance(clazz, list.size());
return list.toArray(array);
}
public static int[] toPrimativArray(final Iterable iterable) {
final List list = asList(iterable);
final Integer[] dummy = (Integer[]) Array.newInstance(Integer.class, list.size());
final Integer[] array = list.toArray(dummy);
return ArrayUtilities.primitives(array);
}
public static List asList(final Iterable iterable) {
final List result = new ArrayList<>();
for (final T item : iterable) {
result.add(item);
}
return result;
}
public static List asList(final Iterable iterable, final IAcceptor validator) {
final List result = new ArrayList<>();
for (final T item : iterable) {
if (validator.accept(item)) {
result.add(item);
}
}
return result;
}
public static boolean containsAcceptedItems(final Iterable values, final IAcceptor validator) {
for (final T value : values) {
if (validator.accept(value)) {
return true;
}
}
return false;
}
public static Iterable convert(
final Iterable values,
final IConverter converter) throws E {
final List result = new ArrayList<>();
for (final I value : values) {
result.add(converter.convert(value));
}
return result;
}
@SuppressWarnings("unchecked")
public static Iterable concat(final Iterable... values) {
final List result = new ArrayList<>();
for (final Iterable value : values) {
for (final I item : value) {
result.add(item);
}
}
return result;
}
public static Iterable normalize(final Iterable values) {
final List result = new ArrayList<>();
for (final I item : values) {
if (item == null) {
continue;
}
result.add(item);
}
return result;
}
public static String toString(final Iterable iterable, final String seperator) {
final StringBuilder builder = new StringBuilder();
boolean flag = false;
for (final T parameter : iterable) {
if (flag) {
builder.append(seperator);
}
builder.append(parameter);
flag = true;
}
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy