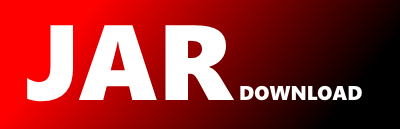
net.anwiba.commons.lang.functional.IIterable Maven / Gradle / Ivy
/*
* #%L
* anwiba commons core
* %%
* Copyright (C) 2007 - 2016 Andreas Bartels
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 2.1 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package net.anwiba.commons.lang.functional;
import java.util.NoSuchElementException;
public interface IIterable {
IIterator iterator();
default IIterable iterable(final IAcceptor acceptor) {
return new IIterable() {
@Override
public IIterator iterator() {
return iterator(acceptor);
}
};
}
default IIterator iterator(final IAcceptor acceptor) {
return new IIterator() {
private final IIterator iterator = iterator();
private O item = null;
@Override
public boolean hasNext() throws E {
while (this.iterator.hasNext()) {
final O i = this.iterator.next();
if (acceptor.accept(i) && (this.item = i) != null) {
return true;
}
}
return false;
}
@Override
public O next() throws E {
try {
if (this.item != null || hasNext()) {
return this.item;
}
throw new NoSuchElementException();
} finally {
this.item = null;
}
}
};
}
default O first(final IAcceptor acceptor) throws E {
final IIterator iterator = this.iterator();
while (iterator.hasNext()) {
final O value = iterator.next();
if (acceptor.accept(value)) {
return value;
}
}
return null;
}
default void foreach(final IConsumer consumer) throws E {
final IIterator iterator = this.iterator();
while (iterator.hasNext()) {
final O value = iterator.next();
consumer.consume(value);
}
}
default R aggregate(final R identity, final IAggregator adder) throws E {
final IIterator iterator = this.iterator();
R result = identity;
while (iterator.hasNext()) {
result = adder.aggregate(result, iterator.next());
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy