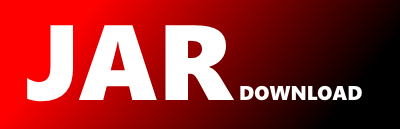
net.anwiba.commons.lang.optional.Optional Maven / Gradle / Ivy
/*
* #%L
* anwiba commons core
* %%
* Copyright (C) 2007 - 2016 Andreas Bartels
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 2.1 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package net.anwiba.commons.lang.optional;
import net.anwiba.commons.lang.functional.IAcceptor;
import net.anwiba.commons.lang.functional.IBlock;
import net.anwiba.commons.lang.functional.IConsumer;
import net.anwiba.commons.lang.functional.IConverter;
import net.anwiba.commons.lang.functional.ISupplier;
import net.anwiba.commons.lang.object.ObjectUtilities;
public class Optional implements IOptional {
private final T value;
private final IAcceptor acceptor;
private Optional(final IAcceptor acceptor, final T value) {
this.acceptor = acceptor;
this.value = value;
}
public static IOptional of(final T value) {
return create(i -> i != null, value);
}
public static IOptional of(final IAcceptor acceptor, final T value) {
return create(acceptor, value);
}
public static IOptional create(final T value) {
return create(i -> i != null, value);
}
public static IOptional create(final IAcceptor acceptor, final T value) {
return new Optional<>(acceptor, value);
}
@Override
public T get() throws E {
if (isAccepted()) {
return this.value;
}
return null;
}
@Override
public IOptional accept(final IAcceptor acceptor) throws E {
if (isAccepted()) {
return new Optional<>(acceptor, this.value);
}
return create(null);
}
@Override
public boolean isAccepted() {
return this.acceptor.accept(this.value);
}
@Override
public IOptional convert(final IConverter converter) throws E {
if (isAccepted()) {
return create(converter.convert(this.value));
}
return create(null);
}
@Override
public IOptional consum(final IConsumer consumer) throws E {
if (isAccepted()) {
consumer.consume(this.value);
}
return this;
}
// @Override
// public IOptional or(final IConsumer consumer) throws E {
// if (!isAccepted()) {
// consumer.consume(this.value);
// }
// return this;
// }
@Override
public IOptional or(final IBlock block) throws E {
if (!isAccepted()) {
block.execute();
}
return this;
}
@Override
public IOptional equals(final IConverter converter, final O other) throws E {
if (isAccepted() && ObjectUtilities.equals(converter.convert(this.value), other)) {
return this;
}
return create(null);
}
@Override
public T getOrThrow(final X throwable) throws E, X {
if (isAccepted()) {
return this.value;
}
throw throwable;
}
@Override
public T getOrThrow(final ISupplier supplier) throws E, X {
if (isAccepted()) {
return this.value;
}
throw supplier.supply();
}
@Override
public T getOr(final T value) throws E {
if (isAccepted()) {
return this.value;
}
return value;
}
@Override
public T getOr(final ISupplier supplier) throws E {
if (isAccepted()) {
return this.value;
}
return supplier.supply();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy