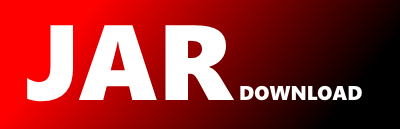
net.anwiba.commons.reflection.InjektionAnalyserResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anwiba-commons-reflaction Show documentation
Show all versions of anwiba-commons-reflaction Show documentation
anwiba commons reflaction project
/*
* #%L
* anwiba commons core
* %%
* Copyright (C) 2007 - 2016 Andreas Bartels
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 2.1 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package net.anwiba.commons.reflection;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@SuppressWarnings("rawtypes")
public final class InjektionAnalyserResult implements IInjektionAnalyserResult {
private final List results;
private final List types;
private final Map map;
private final Class type;
private final IInjectingFactory factory;
public static IInjektionAnalyserResult create(final Class type, final List results) {
return create(type, results, null);
}
public static IInjektionAnalyserResult create(
final Class type,
final List results,
final IInjectingFactory factory) {
final List types = new ArrayList<>();
final Map map = new HashMap<>();
for (final IInjektionAnalyserValueResult result : results) {
types.add(result.getType());
map.put(result.getType(), result);
}
return new InjektionAnalyserResult(type, results, types, map, factory);
}
private InjektionAnalyserResult(
final Class type,
final List results,
final List types,
final Map map,
final IInjectingFactory factory) {
this.type = type;
this.results = results;
this.types = types;
this.map = map;
this.factory = factory;
}
@Override
public Iterable getTypes() {
return this.types;
}
@Override
public boolean isNullable(final Class clazz) {
final IInjektionAnalyserValueResult result = this.map.get(clazz);
if (result == null) {
throw new IllegalStateException();
}
return result.isNullable();
}
@Override
public boolean isIterable(final Class clazz) {
final IInjektionAnalyserValueResult result = this.map.get(clazz);
if (result == null) {
throw new IllegalStateException();
}
return result.isIterable();
}
@Override
public boolean hasNullable() {
return this.results.stream().anyMatch(i -> i.isNullable());
}
@Override
public boolean hasIterable() {
return this.results.stream().anyMatch(i -> i.isIterable());
}
@Override
public boolean isIndependent() {
return this.types.isEmpty();
}
@Override
public Class getType() {
return this.type;
}
@Override
public IInjectingFactory getFactory() {
return this.factory;
}
@Override
public boolean isFactory() {
return this.factory != null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy