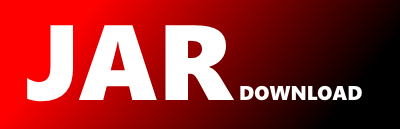
net.anwiba.commons.reflection.ReflectionValueInjector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anwiba-commons-reflaction Show documentation
Show all versions of anwiba-commons-reflaction Show documentation
anwiba commons reflaction project
/*
* #%L
* anwiba commons core
* %%
* Copyright (C) 2007 - 2016 Andreas Bartels
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 2.1 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package net.anwiba.commons.reflection;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.lang.reflect.Parameter;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import net.anwiba.commons.reflection.annotation.Injection;
import net.anwiba.commons.reflection.annotation.Nullable;
import net.anwiba.commons.reflection.privileged.PrivilegedActionInvoker;
import net.anwiba.commons.reflection.privileged.PrivilegedFieldSetterAction;
public class ReflectionValueInjector implements IReflectionValueInjector {
final private InjectableElementGetter injectableElementGetter = new InjectableElementGetter();
private final PrivilegedActionInvoker invoker = new PrivilegedActionInvoker<>(System.getSecurityManager());
private final IReflectionValueProvider values;
public ReflectionValueInjector(final IReflectionValueProvider values) {
this.values = values;
}
@Override
@SuppressWarnings("nls")
public T create(final IInjectingFactory factory) throws CreationException {
final Method[] methods = factory.getClass().getMethods();
final List createMethods = Stream
.of(methods) //
.filter(m -> "create".equals(m.getName()))
.filter(m -> m.getAnnotation(Injection.class) != null)
.collect(Collectors.toList());
if (createMethods.isEmpty()) {
throw new CreationException("");
}
if (createMethods.size() > 1) {
throw new CreationException("");
}
try {
final Method method = createMethods.get(0);
final Class>[] parameterTypes = method.getParameterTypes();
@SuppressWarnings("unchecked")
final Class extends IInjectingFactory> factoryClass = (Class extends IInjectingFactory>) factory
.getClass();
final ReflectionMethodInvoker extends IInjectingFactory, T> methodInvoker = new ReflectionMethodInvoker<>(
factoryClass,
"create",
parameterTypes);
final Object[] methodValues = getValues(method.getParameters());
return methodInvoker.invoke(factory, methodValues);
} catch (final IllegalStateException exception) {
throw new CreationException("Couldn't invoke method create instance of class '" //$NON-NLS-1$
+ factory.getClass().getName()
+ "'", exception); //$NON-NLS-1$
} catch (final InvocationTargetException exception) {
throw new CreationException("Couldn't invoke method create instance of class '" //$NON-NLS-1$
+ factory.getClass().getName()
+ "'", exception.getCause()); //$NON-NLS-1$
}
}
@Override
public T create(final Class clazz) throws CreationException {
try {
final Constructor constructor = this.injectableElementGetter.getConstructor(clazz);
final ReflectionConstructorInvoker constructorInvoker = new ReflectionConstructorInvoker<>(
clazz,
constructor.getParameterTypes());
final Object[] constructorValues = getValues(constructor.getParameters());
final T object = constructorInvoker.invoke(constructorValues);
inject(object);
return object;
} catch (final InvocationTargetException exception) {
throw new CreationException("Couldn't create instance for class '" //$NON-NLS-1$
+ clazz.getName()
+ "'", exception.getCause()); //$NON-NLS-1$
} catch (final InjectionException | IllegalArgumentException | IllegalStateException exception) {
throw new CreationException("Couldn't create instance for class '" //$NON-NLS-1$
+ clazz.getName()
+ "'", exception); //$NON-NLS-1$
}
}
private Object[] getValues(final Parameter[] parameters) {
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy