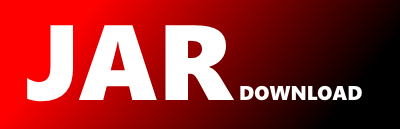
net.anwiba.commons.reflection.privileged.OptionalPrivilegedMethodInvokeAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anwiba-commons-reflaction Show documentation
Show all versions of anwiba-commons-reflaction Show documentation
anwiba commons reflaction project
/*
* #%L
* anwiba commons core
* %%
* Copyright (C) 2007 - 2016 Andreas Bartels
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 2.1 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package net.anwiba.commons.reflection.privileged;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.function.BiFunction;
import java.util.function.Function;
import java.util.stream.Stream;
final public class OptionalPrivilegedMethodInvokeAction extends AbstractPrivilegedAction {
private final Object object;
private final Object[] arguments;
private final Class extends C> clazz;
private final Function methodNameExtractor;
private final Function[]> argumentTypesExtractor;
private final BiFunction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy