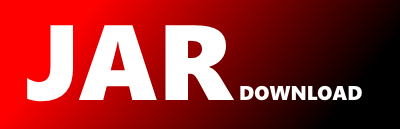
net.anwiba.commons.swing.table.ObjectTableConfiguration Maven / Gradle / Ivy
/*
* #%L
* anwiba commons swing
* %%
* Copyright (C) 2007 - 2016 Andreas Bartels
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 2.1 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package net.anwiba.commons.swing.table;
import java.awt.event.KeyListener;
import java.awt.event.MouseListener;
import java.util.List;
import net.anwiba.commons.model.IBooleanDistributor;
import net.anwiba.commons.model.ISelectionModel;
import net.anwiba.commons.swing.table.action.ITableActionConfiguration;
public class ObjectTableConfiguration implements IObjectTableConfiguration {
private final List extends IColumnConfiguration> columnConfigurations;
private final int selectionMode;
private final ITableActionConfiguration actionConfiguration;
private final int preferredVisibleRowCount;
private final IMouseListenerFactory mouseListenerFactory;
private final IKeyListenerFactory keyListenerFactory;
private final int autoizeMode;
ObjectTableConfiguration(
final int autoizeMode,
final int selectionMode,
final int preferredVisibleRowCount,
final List extends IColumnConfiguration> columnConfigurations,
final IMouseListenerFactory mouseListenerFactory,
final IKeyListenerFactory keyListenerFactory,
final ITableActionConfiguration actionConfiguration) {
this.autoizeMode = autoizeMode;
this.selectionMode = selectionMode;
this.preferredVisibleRowCount = preferredVisibleRowCount;
this.columnConfigurations = columnConfigurations;
this.keyListenerFactory = keyListenerFactory == null ? new IKeyListenerFactory() {
@Override
public KeyListener create(
final IObjectTableModel tableModel,
final ISelectionIndexModel selectionIndicesProvider,
final ISelectionModel selectionModel,
final IBooleanDistributor sortStateModel) {
return null;
}
} : keyListenerFactory;
this.mouseListenerFactory = mouseListenerFactory == null ? new IMouseListenerFactory() {
@Override
public MouseListener create(
final IObjectTableModel tableModel,
final ISelectionIndexModel selectionIndicesProvider,
final ISelectionModel selectionModel,
final IBooleanDistributor sortStateModel) {
return null;
}
} : mouseListenerFactory;
this.actionConfiguration = actionConfiguration;
}
@Override
public IColumnConfiguration getColumnConfiguration(final int columnIndex) {
if (columnIndex < 0 || this.columnConfigurations.size() <= columnIndex) {
return null;
}
return this.columnConfigurations.get(columnIndex);
}
@Override
public int getAutoizeMode() {
return this.autoizeMode;
}
@Override
public int getSelectionMode() {
return this.selectionMode;
}
@Override
public ObjectTableRowSorter getRowSorter(final IObjectTableModel tableModel) {
boolean flag = false;
final ObjectTableRowSorter tableRowSorter = new ObjectTableRowSorter<>(tableModel);
for (int i = 0; i < this.columnConfigurations.size(); i++) {
final IColumnConfiguration columnConfiguration = this.columnConfigurations.get(i);
final boolean isSortable = columnConfiguration.isSortable();
tableRowSorter.setSortable(i, isSortable);
if (columnConfiguration.getComparator() != null) {
tableRowSorter.setComparator(i, columnConfiguration.getComparator());
}
flag |= isSortable;
}
return flag ? tableRowSorter : null;
}
@Override
public ITableActionConfiguration getTableActionConfiguration() {
return this.actionConfiguration;
}
@Override
public int getPreferredVisibleRowCount() {
return this.preferredVisibleRowCount;
}
@Override
public IMouseListenerFactory getMouseListenerFactory() {
return this.mouseListenerFactory;
}
@Override
public IKeyListenerFactory getKeyListenerFactory() {
return this.keyListenerFactory;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy