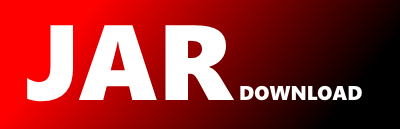
net.anwiba.eclipse.project.dependency.internal.java.Workspace Maven / Gradle / Ivy
/*
* #%L
* anwiba commons core
* %%
* Copyright (C) 2007 - 2017 Andreas Bartels
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 2.1 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package net.anwiba.eclipse.project.dependency.internal.java;
import net.anwiba.eclipse.project.dependency.java.IImport;
import net.anwiba.eclipse.project.dependency.java.ILibrary;
import net.anwiba.eclipse.project.dependency.java.IPackage;
import net.anwiba.eclipse.project.dependency.java.IPath;
import net.anwiba.eclipse.project.dependency.java.IProject;
import net.anwiba.eclipse.project.dependency.java.IType;
import net.anwiba.eclipse.project.dependency.java.IWorkspace;
import java.net.URI;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
public class Workspace implements IWorkspace {
private final Map projects;
private final Map packages;
private final Map> duplicates;
private final Map libraries;
private final Map types;
private final URI uri;
public Workspace(
final URI uri,
final Map projects,
final Map libraries,
final Map packages,
final Map types,
final Map> duplicates) {
this.uri = uri;
this.types = Collections.unmodifiableMap(types);
this.projects = Collections.unmodifiableMap(projects);
this.libraries = Collections.unmodifiableMap(libraries);
this.packages = Collections.unmodifiableMap(packages);
this.duplicates = Collections.unmodifiableMap(duplicates);
}
@Override
public IType[] getTypes(final IPath path) {
final List types = new ArrayList<>();
for (final ILibrary library : this.libraries.values()) {
if (library.containts(path)) {
types.add(library.getType(path));
}
}
for (final IProject project : this.projects.values()) {
if (project.containts(path)) {
types.add(project.getType(path));
}
}
return types.toArray(new IType[types.size()]);
}
@Override
public Map getTypes() {
return this.types;
}
@Override
public Map getPackages() {
return this.packages;
}
@Override
public Map getProjects() {
return this.projects;
}
@Override
public Map getLibraries() {
return this.libraries;
}
@Override
public Map> getDuplicates() {
return this.duplicates;
}
@Override
public URI getUri() {
return this.uri;
}
@Override
public IProject getProject(final String name) {
return this.projects.get("/" + name);
}
@Override
public IPackage getPackage(final String name) {
return this.packages.get(name);
}
@Override
public List getUsed(final IType type) {
final Set result = new HashSet<>();
for (final IImport impcrt : type.getImports()) {
result.addAll(Arrays.asList(getTypes(impcrt.getPath())));
}
for (final IPath path : type.getMethodTypes()) {
result.addAll(Arrays.asList(getTypes(path)));
}
for (final IPath path : type.getAnnotationTypes()) {
result.addAll(Arrays.asList(getTypes(path)));
}
return new ArrayList<>(result);
}
@Override
public List getImplemented(final IType type) {
final List result = new ArrayList<>();
for (final IPath path : type.getSuperTypes()) {
result.addAll(Arrays.asList(getTypes(path)));
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy