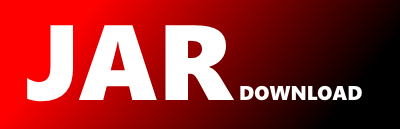
net.anwiba.spatial.ckan.json.schema.v1_0.Resource Maven / Gradle / Ivy
//Copyright (c) 2017 by Andreas W. Bartels
package net.anwiba.spatial.ckan.json.schema.v1_0;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonProperty;
public class Resource
extends Named
{
private String resource_group_id = null;
private net.anwiba.spatial.ckan.json.types.DateString cache_last_updated = null;
private String package_id = null;
private String webstore_last_updated = null;
private Boolean datastore_active = Boolean.valueOf(true);
private String id = null;
private String size = null;
private String state = null;
private String shape_info = null;
private String hash = null;
private String format = null;
private String hdx_rel_url = null;
private String mimetype_inner = null;
private String url_type = null;
private String mimetype = null;
private String cache_url = null;
private net.anwiba.spatial.ckan.json.types.DateString created = null;
private String url = null;
private String webstore_url = null;
private net.anwiba.spatial.ckan.json.types.DateString last_modified = null;
private Integer position = Integer.valueOf(0);
private String revision_id = null;
private String resource_type = null;
private final Map _unknownMembers = new LinkedHashMap();
@JsonProperty("resource_group_id")
public void setResource_group_id(final String resource_group_id) {
this.resource_group_id = resource_group_id;
}
@JsonProperty("resource_group_id")
public String getResource_group_id() {
return this.resource_group_id;
}
@JsonProperty("cache_last_updated")
public void setCache_last_updated(final net.anwiba.spatial.ckan.json.types.DateString cache_last_updated) {
this.cache_last_updated = cache_last_updated;
}
@JsonProperty("cache_last_updated")
public net.anwiba.spatial.ckan.json.types.DateString getCache_last_updated() {
return this.cache_last_updated;
}
@JsonProperty("package_id")
public void setPackage_id(final String package_id) {
this.package_id = package_id;
}
@JsonProperty("package_id")
public String getPackage_id() {
return this.package_id;
}
@JsonProperty("webstore_last_updated")
public void setWebstore_last_updated(final String webstore_last_updated) {
this.webstore_last_updated = webstore_last_updated;
}
@JsonProperty("webstore_last_updated")
public String getWebstore_last_updated() {
return this.webstore_last_updated;
}
@JsonProperty("datastore_active")
public void setDatastore_active(final Boolean datastore_active) {
this.datastore_active = datastore_active;
}
@JsonProperty("datastore_active")
public Boolean isDatastore_active() {
return this.datastore_active;
}
@JsonProperty("id")
public void setId(final String id) {
this.id = id;
}
@JsonProperty("id")
public String getId() {
return this.id;
}
@JsonProperty("size")
public void setSize(final String size) {
this.size = size;
}
@JsonProperty("size")
public String getSize() {
return this.size;
}
@JsonProperty("state")
public void setState(final String state) {
this.state = state;
}
@JsonProperty("state")
public String getState() {
return this.state;
}
@JsonProperty("shape_info")
public void setShape_info(final String shape_info) {
this.shape_info = shape_info;
}
@JsonProperty("shape_info")
public String getShape_info() {
return this.shape_info;
}
@JsonProperty("hash")
public void setHash(final String hash) {
this.hash = hash;
}
@JsonProperty("hash")
public String getHash() {
return this.hash;
}
@JsonProperty("format")
public void setFormat(final String format) {
this.format = format;
}
@JsonProperty("format")
public String getFormat() {
return this.format;
}
@JsonProperty("hdx_rel_url")
public void setHdx_rel_url(final String hdx_rel_url) {
this.hdx_rel_url = hdx_rel_url;
}
@JsonProperty("hdx_rel_url")
public String getHdx_rel_url() {
return this.hdx_rel_url;
}
@JsonProperty("mimetype_inner")
public void setMimetype_inner(final String mimetype_inner) {
this.mimetype_inner = mimetype_inner;
}
@JsonProperty("mimetype_inner")
public String getMimetype_inner() {
return this.mimetype_inner;
}
@JsonProperty("url_type")
public void setUrl_type(final String url_type) {
this.url_type = url_type;
}
@JsonProperty("url_type")
public String getUrl_type() {
return this.url_type;
}
@JsonProperty("mimetype")
public void setMimetype(final String mimetype) {
this.mimetype = mimetype;
}
@JsonProperty("mimetype")
public String getMimetype() {
return this.mimetype;
}
@JsonProperty("cache_url")
public void setCache_url(final String cache_url) {
this.cache_url = cache_url;
}
@JsonProperty("cache_url")
public String getCache_url() {
return this.cache_url;
}
@JsonProperty("created")
public void setCreated(final net.anwiba.spatial.ckan.json.types.DateString created) {
this.created = created;
}
@JsonProperty("created")
public net.anwiba.spatial.ckan.json.types.DateString getCreated() {
return this.created;
}
@JsonProperty("url")
public void setUrl(final String url) {
this.url = url;
}
@JsonProperty("url")
public String getUrl() {
return this.url;
}
@JsonProperty("webstore_url")
public void setWebstore_url(final String webstore_url) {
this.webstore_url = webstore_url;
}
@JsonProperty("webstore_url")
public String getWebstore_url() {
return this.webstore_url;
}
@JsonProperty("last_modified")
public void setLast_modified(final net.anwiba.spatial.ckan.json.types.DateString last_modified) {
this.last_modified = last_modified;
}
@JsonProperty("last_modified")
public net.anwiba.spatial.ckan.json.types.DateString getLast_modified() {
return this.last_modified;
}
@JsonProperty("position")
public void setPosition(final Integer position) {
this.position = position;
}
@JsonProperty("position")
public Integer getPosition() {
return this.position;
}
@JsonProperty("revision_id")
public void setRevision_id(final String revision_id) {
this.revision_id = revision_id;
}
@JsonProperty("revision_id")
public String getRevision_id() {
return this.revision_id;
}
@JsonProperty("resource_type")
public void setResource_type(final String resource_type) {
this.resource_type = resource_type;
}
@JsonProperty("resource_type")
public String getResource_type() {
return this.resource_type;
}
@JsonAnySetter
public void set(final java.lang.String name, final Object value) {
Objects.requireNonNull(name);
this._unknownMembers.put(name, value);
}
@JsonAnyGetter
public Map get() {
if (this._unknownMembers.isEmpty()) {
return null;
}
return this._unknownMembers;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy