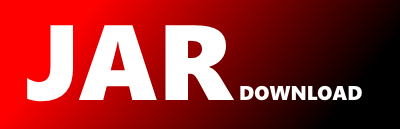
net.anwiba.spatial.osm.overpass.schema.v00_6.OverpassObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anwiba-spatial-data-osm Show documentation
Show all versions of anwiba-spatial-data-osm Show documentation
anwiba spatial open street map json io project
//Copyright (c) 2017 by Andreas W. Bartels
package net.anwiba.spatial.osm.overpass.schema.v00_6;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSubTypes;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME, include = JsonTypeInfo.As.PROPERTY, property = "type")
@JsonSubTypes({
@JsonSubTypes.Type(Node.class),
@JsonSubTypes.Type(Relation.class),
@JsonSubTypes.Type(Way.class)
})
public class OverpassObject {
private final String type = "OverpassObject";
private Object id = null;
private Bounds bounds = null;
private Double lat = null;
private Double lon = null;
private String timestamp = null;
private Integer version = null;
private Integer changeset = null;
private String user = null;
private Object uid = null;
private Tags tags = null;
private final Map _unknownMembers = new LinkedHashMap();
@JsonIgnore
public void setType(final String type) {
}
@JsonIgnore
public String getType() {
return this.type;
}
@JsonProperty("id")
public void setId(final Object id) {
this.id = id;
}
@JsonProperty("id")
public Object getId() {
return this.id;
}
@JsonProperty("bounds")
public void setBounds(final Bounds bounds) {
this.bounds = bounds;
}
@JsonProperty("bounds")
public Bounds getBounds() {
return this.bounds;
}
@JsonProperty("lat")
public void setLat(final Double lat) {
this.lat = lat;
}
@JsonProperty("lat")
public Double getLat() {
return this.lat;
}
@JsonProperty("lon")
public void setLon(final Double lon) {
this.lon = lon;
}
@JsonProperty("lon")
public Double getLon() {
return this.lon;
}
@JsonProperty("timestamp")
public void setTimestamp(final String timestamp) {
this.timestamp = timestamp;
}
@JsonProperty("timestamp")
public String getTimestamp() {
return this.timestamp;
}
@JsonProperty("version")
public void setVersion(final Integer version) {
this.version = version;
}
@JsonProperty("version")
public Integer getVersion() {
return this.version;
}
@JsonProperty("changeset")
public void setChangeset(final Integer changeset) {
this.changeset = changeset;
}
@JsonProperty("changeset")
public Integer getChangeset() {
return this.changeset;
}
@JsonProperty("user")
public void setUser(final String user) {
this.user = user;
}
@JsonProperty("user")
public String getUser() {
return this.user;
}
@JsonProperty("uid")
public void setUid(final Object uid) {
this.uid = uid;
}
@JsonProperty("uid")
public Object getUid() {
return this.uid;
}
@JsonProperty("tags")
public void setTags(final Tags tags) {
this.tags = tags;
}
@JsonProperty("tags")
public Tags getTags() {
return this.tags;
}
@JsonAnySetter
public void set(final java.lang.String name, final java.lang.Object value) {
Objects.requireNonNull(name);
this._unknownMembers.put(name, value);
}
@JsonAnyGetter
public Map get() {
if (this._unknownMembers.isEmpty()) {
return null;
}
return this._unknownMembers;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy