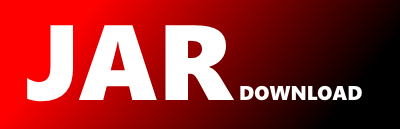
net.anwiba.spatial.osm.nominatim.schema.v01_0.Address Maven / Gradle / Ivy
//Copyright (c) 2017 by Andreas W. Bartels ([email protected])
package net.anwiba.spatial.osm.nominatim.schema.v01_0;
import java.lang.reflect.InvocationTargetException;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonProperty;
import net.anwiba.commons.reflection.OptionalReflectionMethodInvoker;
public class Address {
private String house_number = null;
private String road = null;
private String neighbourhood = null;
private String suburb = null;
private String city_district = null;
private String city = null;
private String county = null;
private String state_district = null;
private String state = null;
private String postcode = null;
private String country = null;
private String country_code = null;
private String continent = null;
private final Map _unknownMembers = new LinkedHashMap();
@JsonProperty("house_number")
public void setHouse_number(final String house_number) {
this.house_number = house_number;
}
@JsonProperty("house_number")
public String getHouse_number() {
return this.house_number;
}
@JsonProperty("road")
public void setRoad(final String road) {
this.road = road;
}
@JsonProperty("road")
public String getRoad() {
return this.road;
}
@JsonProperty("neighbourhood")
public void setNeighbourhood(final String neighbourhood) {
this.neighbourhood = neighbourhood;
}
@JsonProperty("neighbourhood")
public String getNeighbourhood() {
return this.neighbourhood;
}
@JsonProperty("suburb")
public void setSuburb(final String suburb) {
this.suburb = suburb;
}
@JsonProperty("suburb")
public String getSuburb() {
return this.suburb;
}
@JsonProperty("city_district")
public void setCity_district(final String city_district) {
this.city_district = city_district;
}
@JsonProperty("city_district")
public String getCity_district() {
return this.city_district;
}
@JsonProperty("city")
public void setCity(final String city) {
this.city = city;
}
@JsonProperty("city")
public String getCity() {
return this.city;
}
@JsonProperty("county")
public void setCounty(final String county) {
this.county = county;
}
@JsonProperty("county")
public String getCounty() {
return this.county;
}
@JsonProperty("state_district")
public void setState_district(final String state_district) {
this.state_district = state_district;
}
@JsonProperty("state_district")
public String getState_district() {
return this.state_district;
}
@JsonProperty("state")
public void setState(final String state) {
this.state = state;
}
@JsonProperty("state")
public String getState() {
return this.state;
}
@JsonProperty("postcode")
public void setPostcode(final String postcode) {
this.postcode = postcode;
}
@JsonProperty("postcode")
public String getPostcode() {
return this.postcode;
}
@JsonProperty("country")
public void setCountry(final String country) {
this.country = country;
}
@JsonProperty("country")
public String getCountry() {
return this.country;
}
@JsonProperty("country_code")
public void setCountry_code(final String country_code) {
this.country_code = country_code;
}
@JsonProperty("country_code")
public String getCountry_code() {
return this.country_code;
}
@JsonProperty("continent")
public void setContinent(final String continent) {
this.continent = continent;
}
@JsonProperty("continent")
public String getContinent() {
return this.continent;
}
private void _inject(java.lang.String name, Object value) {
try {
OptionalReflectionMethodInvoker setterInvoker = OptionalReflectionMethodInvoker.createSetter(this.getClass(), "JsonProperty", "value", name);
setterInvoker.invoke(this, value);
} catch (InvocationTargetException exception) {
throw new RuntimeException(exception);
}
}
@JsonAnySetter
public void set(final java.lang.String name, final Object value) {
Objects.requireNonNull(name);
_inject(name, value);
this._unknownMembers.put(name, value);
}
@JsonAnyGetter
public Map get() {
if (this._unknownMembers.isEmpty()) {
return null;
}
return this._unknownMembers;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy