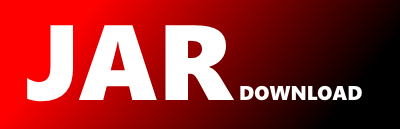
net.apexes.commons.i18n.I18nMsg Maven / Gradle / Ivy
/*
* Copyright (c) 2018, apexes.net. All rights reserved.
*
* http://www.apexes.net
*
*/
package net.apexes.commons.i18n;
import net.apexes.commons.lang.Strings;
import java.text.MessageFormat;
import java.util.Enumeration;
import java.util.Locale;
/**
* @author HeDYn
*/
public class I18nMsg {
protected final Logger logger;
protected final I18nResource resource;
public I18nMsg(I18nResource resource) {
this(resource, new IgnoreLogger());
}
public I18nMsg(I18nResource resource, Logger logger) {
this.resource = resource;
this.logger = logger;
}
public Locale getLocale() {
return resource.getLocale();
}
public Enumeration getKeys() {
return resource.getKeys();
}
public boolean containsKey(String key) {
return resource.containsKey(key);
}
public String getMessage(String key) {
return getMessage(key, key);
}
public String getMessage(String key, String defaultValue) {
// 保持与旧版本兼容,旧版本在 key 为 null 时会返回 defaultValue
if (key == null) {
logger.logNotFoundKey(resource.getLocale(), key);
return defaultValue;
}
if (containsKey(key)) {
return resource.getString(key);
} else {
logger.logNotFoundKey(resource.getLocale(), key);
return defaultValue;
}
}
public String formatKey(String key, Object... params) {
return format(getMessage(key), params);
}
/**
* 使用 fmt 做模板格式化给定的参数,作用与 {@link MessageFormat#format(String, Object...)} 相同
* @param fmt 模板
* @param params 参数
* @return 返回格式化后的字符串
*/
private String format(String fmt, Object... params) {
try {
return Strings.format(fmt, params);
} catch (Exception e) {
logger.logFormatError(resource.getLocale(), e, fmt, params);
}
return fmt;
}
/**
* @author HeDYn
*/
public interface Logger {
void logNotFoundKey(Locale locale, String key);
void logFormatError(Locale locale, Exception e, String format, Object... params);
}
/**
*
* @author HeDYn
*/
private static class IgnoreLogger implements Logger {
@Override
public void logNotFoundKey(Locale locale, String key) {
}
@Override
public void logFormatError(Locale locale, Exception e, String format, Object... params) {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy