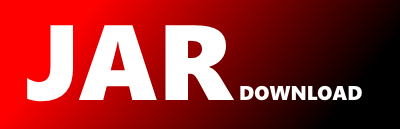
net.apexes.commons.lang.Resources Maven / Gradle / Ivy
/*
* Copyright (c) 2018, apexes.net. All rights reserved.
*
* http://www.apexes.net
*
*/
package net.apexes.commons.lang;
import java.io.Closeable;
import java.io.File;
import java.io.UnsupportedEncodingException;
import java.lang.reflect.Method;
import java.net.URLDecoder;
/**
* @author HeDYn
*/
public final class Resources {
private Resources() {}
public static void close(Closeable... closeables) {
for (Closeable closeable : closeables) {
close(closeable);
}
}
public static void close(Closeable closeable) {
if (closeable != null) {
try {
closeable.close();
} catch (Exception e) {
// Ignore it.
}
}
}
public static void close(Object... objects) {
for (Object object : objects) {
close(object);
}
}
public static void close(Object object) {
if (object != null) {
if (object instanceof Closeable) {
close((Closeable) object);
} else {
try {
Method method = object.getClass().getMethod("close");
method.invoke(object, new Object[0]);
} catch (Exception e) {
// Ignore it.
}
}
}
}
/**
* 获取包含指定类的jar文件
*
* @param classType
* @return 返回包含指定类的jar文件,如果指定类未打包成jar则返回null
*/
public static File getJarFile(Class> classType) {
File file = getProjectFile(classType);
if (file != null && file.getName().endsWith(".jar") && file.isFile()) {
return file;
}
return null;
}
/**
* 获取指定类的工程路径或所在jar文件路径
*
* @param classType
* @return 返回指定类的工程路径或所在jar文件路径(不包含类所在的包路径)
*/
public static String getProjectPath(Class> classType) {
File file = getProjectFile(classType);
if (file != null) {
return file.getAbsolutePath();
}
return null;
}
/**
* 获取指定类的工程目录或所在jar文件
*
* @param classType
* @return 返回指定类的工程目录或所在jar文件(不包含类所在的包路径)
*/
public static File getProjectFile(Class> classType) {
File file = null;
if (classType.getClassLoader() == null) {
String className = classType.getSimpleName() + ".class";
String filePath = classType.getResource(className).getPath();
try {
filePath = URLDecoder.decode(filePath, "utf-8");
} catch (UnsupportedEncodingException e) {
// ignore
}
if (filePath.startsWith("file:/")) {
filePath = filePath.substring(6, filePath.indexOf(".jar!") + 4);
file = new File(filePath);
}
} else {
String filePath = classType.getProtectionDomain().getCodeSource().getLocation().getPath();
try {
filePath = URLDecoder.decode(filePath, "utf-8");
} catch (UnsupportedEncodingException e) {
// ignore
}
file = new File(filePath);
}
return file;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy