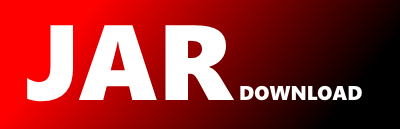
net.automatalib.ts.simple.SimpleTS Maven / Gradle / Ivy
/* Copyright (C) 2013 TU Dortmund
* This file is part of AutomataLib, http://www.automatalib.net/.
*
* AutomataLib is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License version 3.0 as published by the Free Software Foundation.
*
* AutomataLib is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with AutomataLib; if not, see
* http://www.gnu.de/documents/lgpl.en.html.
*/
package net.automatalib.ts.simple;
import java.util.Collection;
import java.util.Set;
import net.automatalib.commons.util.mappings.MutableMapping;
/**
* A simple transition system. A transition system is a (not necessarily finite) collection
* of states. For an arbitrary input symbol, each state has a set of successors.
*
* @author Malte Isberner
*
* @param state class.
* @param symbol class.
*/
public interface SimpleTS {
/**
* Retrieves the set of initial states of the transition system.
* @return the initial states.
*/
public Set getInitialStates();
/**
* Retrieves the set of successors for the given input symbol.
*
* @param state the source state.
* @param input the input symbol.
* @return the set of successors reachable by this input, or
* null
if no successor states are reachable by this input.
*/
public Set getSuccessors(S state, I input);
/**
* Retrieves the set of successors for the given sequence of input symbols.
*
* @param state the source state.
* @param input the sequence of input symbols.
* @return the set of successors reachable by this input, or
* null
if no successor states are reachable by this input.
*/
public Set getSuccessors(S state, Iterable input);
/**
* Retrieves the set of all successors that can be reached from any
* of the given source states by the specified sequence of input symbols.
*
* @param states the source states.
* @param input the sequence of input symbols.
* @return the set of successors reachable by this input, or null
* if no successor states are reachable.
*/
public Set getSuccessors(Collection states, Iterable input);
/**
* Retrieves the set of all states reachable by the given sequence of input
* symbols from an initial state. Calling this method is equivalent to
* getSuccessors(getInitialStates(), input)
.
*
* @param input the sequence of input symbols.
* @return the set of states reachable by this input from an initial state,
* or null
if no successor state is reachable.
*/
public Set getStates(Iterable input);
/**
* Creates a {@link MutableMapping} allowing to associate arbitrary data
* with this transition system's states. The returned mapping is however
* only guaranteed to work correctly if the transition system is not
* modified.
* @return the mutable mapping
*/
public MutableMapping createStaticStateMapping();
/**
* Creates a {@link MutableMapping} allowing to associate arbitrary data
* with this transition system's states. The returned mapping maintains
* the association even when the transition system is modified.
* @return the mutable mapping
*/
public MutableMapping createDynamicStateMapping();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy