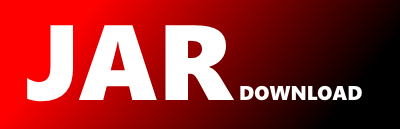
net.automatalib.automata.vpda.DefaultOneSEVPA Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of automata-core Show documentation
Show all versions of automata-core Show documentation
The core artifact of AutomataLib. This library contains concrete implementations for many
of the automaton model interfaces defined in the API artifact, as well as abstract
base classes that facilitate implementing new automaton model classes. Note that
concrete algorithms (traversal, reachability analysis etc.) are not in the scope of this
artifact.
/* Copyright (C) 2013-2018 TU Dortmund
* This file is part of AutomataLib, http://www.automatalib.net/.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.automatalib.automata.vpda;
import java.util.ArrayList;
import java.util.List;
import net.automatalib.words.VPDAlphabet;
/**
* Default implementation for 1-SEVPA.
*
* @param
* input symbol type
*
* @author Malte Isberner
*/
public class DefaultOneSEVPA extends AbstractOneSEVPA {
private final List locations;
private Location initLoc;
public DefaultOneSEVPA(final VPDAlphabet alphabet) {
super(alphabet);
this.locations = new ArrayList<>();
}
public DefaultOneSEVPA(final VPDAlphabet alphabet, final int capacity) {
super(alphabet);
this.locations = new ArrayList<>(capacity);
}
public Location addInitialLocation(final boolean accepting) {
final Location loc = addLocation(accepting);
setInitialLocation(loc);
return loc;
}
public Location addLocation(final boolean accepting) {
final Location loc = new Location(alphabet, locations.size(), accepting);
locations.add(loc);
return loc;
}
public int size() {
return locations.size();
}
public void setInternalSuccessor(final Location loc, final I intSym, final Location succ) {
loc.setInternalSuccessor(alphabet.getInternalSymbolIndex(intSym), succ);
}
public void setReturnSuccessor(final Location loc, final I retSym, final int stackSym, final Location succ) {
loc.setReturnSuccessor(alphabet.getReturnSymbolIndex(retSym), stackSym, succ);
}
@Override
public Location getInternalSuccessor(final Location loc, final I intSym) {
return loc.getInternalSuccessor(alphabet.getInternalSymbolIndex(intSym));
}
@Override
public Location getLocation(final int id) {
return locations.get(id);
}
public int getLocationId(final Location loc) {
return loc.getIndex();
}
public List getLocations() {
return locations;
}
@Override
public Location getReturnSuccessor(final Location loc, final I retSym, final int stackSym) {
return loc.getReturnSuccessor(alphabet.getReturnSymbolIndex(retSym), stackSym);
}
@Override
public boolean isAcceptingLocation(final Location loc) {
return loc.isAccepting();
}
public Location getInitialLocation() {
return initLoc;
}
public void setInitialLocation(final Location loc) {
this.initLoc = loc;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy