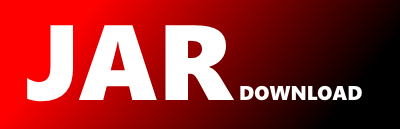
io.guixer.maven.AbstractGuixerOutMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of guixer-maven-plugin Show documentation
Show all versions of guixer-maven-plugin Show documentation
Maven plugin to handle logs emitted by tests run over guixer-tools.
package io.guixer.maven;
import static com.google.common.base.Preconditions.checkNotNull;
import java.io.File;
import java.io.IOException;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.project.MavenProject;
import io.guixer.logs.GuixerOut;
import io.guixer.logs.GuixerOutLoader;
public abstract class AbstractGuixerOutMojo extends AbstractMojo {
protected static File getTargetDir(
final MavenProject project
) throws MojoExecutionException {
final String targetDirPath = project.getBuild().getDirectory();
final File plainTargetDir = new File(targetDirPath);
if (plainTargetDir.isDirectory()) {
return plainTargetDir;
}
final File stupidTargetDir = new File("target");
if (stupidTargetDir.isDirectory()) {
return stupidTargetDir;
}
throw new MojoExecutionException("\"target\" (build) directory cannot be found");
}
protected final File getGuixerOutDirectory(
final MavenProject project
) throws MojoExecutionException {
checkNotNull(project, "project");
final File targetDir = getTargetDir(project);
final File guixerOutDir = new File(targetDir, "guixer_out");
if (!guixerOutDir.exists()) {
throw new MojoExecutionException("Cannot find guixer_out directory: " + guixerOutDir.getAbsolutePath());
} else if (!guixerOutDir.isDirectory()) {
throw new MojoExecutionException(
"guixer_out should be a directory, but was: " + guixerOutDir.getAbsolutePath());
}
return guixerOutDir;
}
protected final GuixerOut loadGuixerOut(
final MavenProject project
) throws MojoExecutionException {
checkNotNull(project, "project");
final File guixerOutDir = getGuixerOutDirectory(project);
final String guixerOutDirPath;
try {
guixerOutDirPath = guixerOutDir.getCanonicalPath();
} catch (final IOException e) {
throw new MojoExecutionException(
"Unable to work with guixer_out directory: " + guixerOutDir.getAbsolutePath(), e);
}
final GuixerOut guixerOut;
try {
guixerOut = new GuixerOutLoader(guixerOutDir).getGuixerOut();
} catch (final IOException e) {
throw new MojoExecutionException("Cannot read guixer_out directory: " + guixerOutDirPath);
}
return guixerOut;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy