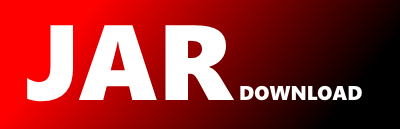
com.google.jstestdriver.server.handlers.FileSetPostHandler Maven / Gradle / Ivy
/*
* Copyright 2009 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.google.jstestdriver.server.handlers;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.reflect.TypeToken;
import com.google.inject.Inject;
import com.google.jstestdriver.CapturedBrowsers;
import com.google.jstestdriver.SlaveBrowser;
import com.google.jstestdriver.model.JstdTestCase;
import com.google.jstestdriver.requesthandlers.RequestHandler;
import com.google.jstestdriver.servlet.fileset.FileSetRequestHandler;
import java.io.IOException;
import java.util.Collection;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* @author [email protected] (Jeremie Lenfant-Engelmann)
*/
class FileSetPostHandler implements RequestHandler {
private final Gson gson = new GsonBuilder().create();
private final HttpServletRequest request;
private final HttpServletResponse response;
private final CapturedBrowsers capturedBrowsers;
private final List> handlers;
@Inject
public FileSetPostHandler(
HttpServletRequest request,
HttpServletResponse response,
CapturedBrowsers capturedBrowsers,
List> handlers) {
this.request = request;
this.response = response;
this.capturedBrowsers = capturedBrowsers;
this.handlers = handlers;
}
@Override
public void handleIt() throws IOException {
final FileSetRequestHandler> handler = handlerFromAction(request);
response.getOutputStream().print(
gson.toJson(handler.handle(browserFromId(request.getParameter("id")),
request.getParameter("data"))));
}
private SlaveBrowser browserFromId(String id) {
if (id == null) {
return null;
}
return capturedBrowsers.getBrowser(id);
}
private FileSetRequestHandler> handlerFromAction(HttpServletRequest req) {
final String action = req.getParameter("action");
for (FileSetRequestHandler> handler : handlers) {
if (handler.canHandle(action)) {
return handler;
}
}
throw new IllegalArgumentException("unknown action");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy