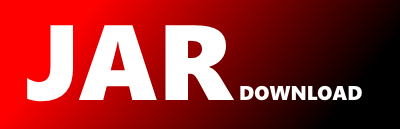
net.bbmsoft.iocfx.Platform Maven / Gradle / Ivy
package net.bbmsoft.iocfx;
import java.util.concurrent.ScheduledFuture;
import java.util.concurrent.TimeUnit;
import javafx.application.Application;
import javafx.application.ConditionalFeature;
import javafx.beans.property.ReadOnlyBooleanProperty;
/**
* Wraps the functionality of {@link javafx.application.Platform} into an OSGi
* service. IoCFX will make sure this service does not become available before
* the JavaFX platform has been launched, so any DS declaring a dependency on
* {@link net.bbmsoft.iocfx.Platform} will only be activated when it is safe to
* use the JavaFX Platform.
*
* @author Michael Bachmann
*
*/
public interface Platform {
/**
* Indicates whether or not accessibility is active. This property is typically
* set to true the first time an assistive technology, such as a screen reader,
* requests information about any JavaFX window or its children.
*
*
* This method may be called from any thread.
*
*
* @return the read-only boolean property indicating if accessibility is active
*
*/
public ReadOnlyBooleanProperty accessibilityActiveProperty();
/**
* Causes the JavaFX application to terminate. If this method is called after
* the Application start method is called, then the JavaFX launcher will call
* the Application stop method and terminate the JavaFX application thread. The
* launcher thread will then shutdown. If there are no other non-daemon threads
* that are running, the Java VM will exit. If this method is called from the
* Preloader or the Application init method, then the Application stop method
* may not be called.
*
*
* This method may be called from any thread.
*
*
*
* Note: if the application is embedded in a browser, then this method may have
* no effect.
*/
public void exit();
public boolean isAccessibilityActive();
/**
* Returns true if the calling thread is the JavaFX Application Thread. Use this
* call the ensure that a given task is being executed (or not being executed)
* on the JavaFX Application Thread.
*
* @return true if running on the JavaFX Application Thread
*/
public boolean isFxApplicationThread();
/**
* Gets the value of the implicitExit attribute.
*
*
* This method may be called from any thread.
*
*
* @return the implicitExit attribute
*/
public boolean isImplicitExit();
/**
* Queries whether a specific conditional feature is supported by the platform.
*
* For example:
*
*
* // Query whether filter effects are supported
* if (Platform.isSupported(ConditionalFeature.EFFECT)) {
* // use effects
* }
*
*
* @param feature
* the conditional feature in question.
* @return {@code true} iff the feature is supported, {@code false} otherwise
*/
public boolean isSupported(ConditionalFeature feature);
/**
* Run the specified Runnable on the JavaFX Application Thread at some
* unspecified time in the future. This method, which may be called from any
* thread, will post the Runnable to an event queue and then return immediately
* to the caller. The Runnables are executed in the order they are posted. A
* runnable passed into the runLater method will be executed before any Runnable
* passed into a subsequent call to runLater. If this method is called after the
* JavaFX runtime has been shutdown, the call will be ignored: the Runnable will
* not be executed and no exception will be thrown.
*
*
* NOTE: applications should avoid flooding JavaFX with too many pending
* Runnables. Otherwise, the application may become unresponsive. Applications
* are encouraged to batch up multiple operations into fewer runLater calls.
* Additionally, long-running operations should be done on a background thread
* where possible, freeing up the JavaFX Application Thread for GUI operations.
*
*
*
* This method must not be called before the FX runtime has been initialized.
* For standard JavaFX applications that extend {@link Application}, and use
* either the Java launcher or one of the launch methods in the Application
* class to launch the application, the FX runtime is initialized by the
* launcher before the Application class is loaded. For Swing applications that
* use JFXPanel to display FX content, the FX runtime is initialized when the
* first JFXPanel instance is constructed. For SWT application that use FXCanvas
* to display FX content, the FX runtime is initialized when the first FXCanvas
* instance is constructed.
*
* Other than {@link javafx.application.Platform#runLater(Runnable)}, this
* method will never throw an IllegalStateException, because the Platform
* service is only created once the JavaFX Platform has been initialized.
*
* @param runnable
* the Runnable whose run method will be executed on the JavaFX
* Application Thread
*/
public void runLater(Runnable runnable);
/**
* Run the specified Runnable on the JavaFX Application Thread at some
* unspecified time in the future. This method does basically the same thing as
* {@link #runLater(Runnable)}, except that when it is called from the JavaFX
* Application thread, it will not add the {@code Runnable} to the thread's
* queue, but instead execute it immediately.
*
* @param runnable
* the Runnable whose run method will be executed on the JavaFX
* Application Thread
*/
public void runOnFxApplicationThread(Runnable runnable);
/**
* Run the specified Runnable on the JavaFX Application Thread at some
* unspecified time in the future. This method does basically the same thing as
* {@link #runLater(Runnable)}, except that it blocks the calling thread until
* the provided {@code Runnable} has been executed.
*
* @param runnable
* the Runnable whose run method will be executed on the JavaFX
* Application Thread
* @throws InterruptedException
* if the calling thread is interrupted while waiting for the
* specified Runnable to complete execution on the JavaFX
* Application Thread
*/
public void runAndWait(Runnable runnable) throws InterruptedException;
/**
* Assert that the current code is running on the JavaFX Application Thread.
* When called from the JavaFX Application Thread, this method will return
* without doing anything, when called from any other thread, it will throw an
* {@link IllegalStateException}.
*
* This method is mainly intended to be used to validate the behavior of an
* Application in development. It should not be abused for control flow! Future
* versions of IoCFX may provide a way to turn this function into a no-op via
* configuration to avoid the overhead of checking the thread on sufficiently
* tested production systems.
*
* @throws IllegalStateException
* if the calling thread is not the JavaFX Application Thread
*/
public void assertFxApplicationThread();
/**
* Sets the implicitExit attribute to the specified value. If this attribute is
* true, the JavaFX runtime will implicitly shutdown when the last window is
* closed; the JavaFX launcher will call the {@link Application#stop} method and
* terminate the JavaFX application thread. If this attribute is false, the
* application will continue to run normally even after the last window is
* closed, until the application calls {@link #exit}. The default value is true.
*
*
* This method may be called from any thread.
*
*
* @param implicitExit
* a flag indicating whether or not to implicitly exit when the last
* window is closed.
*/
public void setImplicitExit(boolean implicitExit);
/**
* Will dispatch the provided {@link Runnable} to the JavaFX Application Thread
* after the given delay. Note that the actual time between calling this method
* and the execution of the provided Runnable may be significantly longer than
* the specified delay, if the JavaFX Application Thread is under heavy load.
*
* @param runnable
* a runnable to be dispatched to the JavaFX Application Thread
* @param delay
* time after which the runnable will be dispatched
* @param unit
* the time unit of the delay parameter
* @return a ScheduledFuture that can be used to cancel
*/
public ScheduledFuture runOnFxApplicationThread(Runnable runnable, long delay, TimeUnit unit);
}