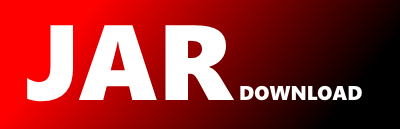
net.bootsfaces.component.navLink.AbstractNavLink Maven / Gradle / Ivy
package net.bootsfaces.component.navLink;
import java.util.Collection;
import java.util.Map;
import net.bootsfaces.component.ajax.IAJAXComponent;
import net.bootsfaces.component.navLink.NavLinkCore.PropertyKeys;
import net.bootsfaces.render.IHasTooltip;
import net.bootsfaces.render.IResponsive;
public interface AbstractNavLink extends IHasTooltip, IAJAXComponent, IResponsive {
String getFamily();
boolean getRendersChildren();
/**
* returns the subset of AJAX requests that are implemented by jQuery
* callback or other non-standard means (such as the onclick event of
* b:tabView, which has to be implemented manually).
*
* @return
*/
Map getJQueryEvents();
Collection getEventNames();
String getDefaultEventName();
/**
* Adds the active state to the link.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
boolean isActive();
/**
* Adds the active state to the link.
*
* Usually this method is called internally by the JSF engine.
*/
void setActive(boolean _active);
/**
* Whether the Button submits the form with AJAX.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
boolean isAjax();
/**
* Whether the Button submits the form with AJAX.
*
* Usually this method is called internally by the JSF engine.
*/
void setAjax(boolean _ajax);
/**
* An el expression referring to a server side UIComponent instance in a
* backing bean.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
javax.faces.component.UIComponent getBinding();
/**
* An el expression referring to a server side UIComponent instance in a
* backing bean.
*
* Usually this method is called internally by the JSF engine.
*/
void setBinding(javax.faces.component.UIComponent _binding);
/**
* contentClass is optional: if specified, the content (i.e. the anchor tag)
* will be displayed with this specific class
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getContentClass();
/**
* contentClass is optional: if specified, the content (i.e. the anchor tag)
* will be displayed with this specific class
*
* Usually this method is called internally by the JSF engine.
*/
void setContentClass(String _contentClass);
/**
* Inline style of the content area (i.e the anchor tag).
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getContentStyle();
/**
* Inline style of the content area (i.e the anchor tag).
*
* Usually this method is called internally by the JSF engine.
*/
void setContentStyle(String _contentStyle);
/**
* The fragment that is to be appended to the target URL. The # separator is
* applied automatically and needs not be included.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getFragment();
/**
* The fragment that is to be appended to the target URL. The # separator is
* applied automatically and needs not be included.
*
* Usually this method is called internally by the JSF engine.
*/
void setFragment(String _fragment);
/**
* If present, this element is rendered as Header in a menu with the text
* specifide by this attribute value: all other attributes will be ignored.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getHeader();
/**
* If present, this element is rendered as Header in a menu with the text
* specifide by this attribute value: all other attributes will be ignored.
*
* Usually this method is called internally by the JSF engine.
*/
void setHeader(String _header);
/**
* URL to link directly to implement anchor behavior.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getHref();
/**
* URL to link directly to implement anchor behavior.
*
* Usually this method is called internally by the JSF engine.
*/
void setHref(String _href);
/**
* Navigation Link Icon, can be one of the Bootstrap's Glyphicons icon
* names. Alignment can be specified with the iconAlign attribute.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getIcon();
/**
* Navigation Link Icon, can be one of the Bootstrap's Glyphicons icon
* names. Alignment can be specified with the iconAlign attribute.
*
* Usually this method is called internally by the JSF engine.
*/
void setIcon(String _icon);
/**
* Alignment can right or left.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getIconAlign();
/**
* Alignment can right or left.
*
* Usually this method is called internally by the JSF engine.
*/
void setIconAlign(String _iconAlign);
/**
* Navigation Link Font Awesome Icon, can be one of the Font Awesome icon
* names. Alignment can be specified with the iconAlign attribute.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getIconAwesome();
/**
* Navigation Link Font Awesome Icon, can be one of the Font Awesome icon
* names. Alignment can be specified with the iconAlign attribute.
*
* Usually this method is called internally by the JSF engine.
*/
void setIconAwesome(String _iconAwesome);
/**
* Flag indicating that, if this component is activated by the user,
* notifications should be delivered to interested listeners and actions
* immediately (that is, during Apply Request Values phase) rather than
* waiting until Invoke Application phase. Default is false.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
boolean isImmediate();
/**
* Flag indicating that, if this component is activated by the user,
* notifications should be delivered to interested listeners and actions
* immediately (that is, during Apply Request Values phase) rather than
* waiting until Invoke Application phase. Default is false.
*
* Usually this method is called internally by the JSF engine.
*/
void setImmediate(boolean _immediate);
/**
*
* Return whether or not the view parameters should be encoded into the
* target url.
*
*
* @since 2.0
*/
boolean isIncludeViewParams();
/**
*
* Set whether or not the page parameters should be encoded into the target
* url.
*
*
* @param includeViewParams
* The state of the switch for encoding page parameters
*
* @since 2.0
*/
void setIncludeViewParams(boolean includeViewParams);
/**
* Client side callback to execute when input element loses focus.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOnblur();
/**
* Client side callback to execute when input element loses focus.
*
* Usually this method is called internally by the JSF engine.
*/
void setOnblur(String _onblur);
/**
* Client side callback to execute when input element loses focus and its
* value has been modified since gaining focus.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOnchange();
/**
* Client side callback to execute when input element loses focus and its
* value has been modified since gaining focus.
*
* Usually this method is called internally by the JSF engine.
*/
void setOnchange(String _onchange);
/**
* The onclick attribute.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOnclick();
/**
* The onclick attribute.
*
* Usually this method is called internally by the JSF engine.
*/
void setOnclick(String _onclick);
/**
* Javascript to be executed when ajax completes with success.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOncomplete();
/**
* Javascript to be executed when ajax completes with success.
*
* Usually this method is called internally by the JSF engine.
*/
void setOncomplete(String _oncomplete);
/**
* Client side callback to execute when input element is double clicked.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOndblclick();
/**
* Client side callback to execute when input element is double clicked.
*
* Usually this method is called internally by the JSF engine.
*/
void setOndblclick(String _ondblclick);
/**
* Client side callback to execute when input element receives focus.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOnfocus();
/**
* Client side callback to execute when input element receives focus.
*
* Usually this method is called internally by the JSF engine.
*/
void setOnfocus(String _onfocus);
/**
* Client side callback to execute when a key is pressed down over input
* element.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOnkeydown();
/**
* Client side callback to execute when a key is pressed down over input
* element.
*
* Usually this method is called internally by the JSF engine.
*/
void setOnkeydown(String _onkeydown);
/**
* Client side callback to execute when a key is pressed and released over
* input element.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOnkeypress();
/**
* Client side callback to execute when a key is pressed and released over
* input element.
*
* Usually this method is called internally by the JSF engine.
*/
void setOnkeypress(String _onkeypress);
/**
* Client side callback to execute when a key is released over input
* element.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOnkeyup();
/**
* Client side callback to execute when a key is released over input
* element.
*
* Usually this method is called internally by the JSF engine.
*/
void setOnkeyup(String _onkeyup);
/**
* Client side callback to execute when a pointer input element is pressed
* down over input element.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOnmousedown();
/**
* Client side callback to execute when a pointer input element is pressed
* down over input element.
*
* Usually this method is called internally by the JSF engine.
*/
void setOnmousedown(String _onmousedown);
/**
* Client side callback to execute when a pointer input element is moved
* within input element.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOnmousemove();
/**
* Client side callback to execute when a pointer input element is moved
* within input element.
*
* Usually this method is called internally by the JSF engine.
*/
void setOnmousemove(String _onmousemove);
/**
* Client side callback to execute when a pointer input element is moved
* away from input element.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOnmouseout();
/**
* Client side callback to execute when a pointer input element is moved
* away from input element.
*
* Usually this method is called internally by the JSF engine.
*/
void setOnmouseout(String _onmouseout);
/**
* Client side callback to execute when a pointer input element is moved
* onto input element.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOnmouseover();
/**
* Client side callback to execute when a pointer input element is moved
* onto input element.
*
* Usually this method is called internally by the JSF engine.
*/
void setOnmouseover(String _onmouseover);
/**
* Client side callback to execute when a pointer input element is released
* over input element.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOnmouseup();
/**
* Client side callback to execute when a pointer input element is released
* over input element.
*
* Usually this method is called internally by the JSF engine.
*/
void setOnmouseup(String _onmouseup);
/**
* Client side callback to execute when text within input element is
* selected by user.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOnselect();
/**
* Client side callback to execute when text within input element is
* selected by user.
*
* Usually this method is called internally by the JSF engine.
*/
void setOnselect(String _onselect);
/**
* The outcome to navigate to.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getOutcome();
/**
* The outcome to navigate to.
*
* Usually this method is called internally by the JSF engine.
*/
void setOutcome(String _outcome);
/**
* Comma or space separated list of ids or search expressions denoting which
* values are to be sent to the server.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getProcess();
/**
* Comma or space separated list of ids or search expressions denoting which
* values are to be sent to the server.
*
* Usually this method is called internally by the JSF engine.
*/
void setProcess(String _process);
/**
* Inline style
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getStyle();
/**
* Inline style
*
* Usually this method is called internally by the JSF engine.
*/
void setStyle(String _style);
/**
* CSS style class
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getStyleClass();
/**
* CSS style class
*
* Usually this method is called internally by the JSF engine.
*/
void setStyleClass(String _styleClass);
/**
* Optional target of the HTML anchor tag that's rendered. E.g. # opens the link in a new tag. This attribute is only evaluated if you provide an href.
* @return Returns the value of the attribute, or null, if it hasn't been set by the JSF file.
*/
String getTarget();
/**
* The text of the tooltip.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getTooltip();
/**
* The text of the tooltip.
*
* Usually this method is called internally by the JSF engine.
*/
void setTooltip(String _tooltip);
/**
* Where is the tooltip div generated? That's primarily a technical value
* that can be used to fix rendering error in special cases. Also see
* data-container in the documentation of Bootstrap. The default value is
* body.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getTooltipContainer();
/**
* Where is the tooltip div generated? That's primarily a technical value
* that can be used to fix rendering error in special cases. Also see
* data-container in the documentation of Bootstrap. The default value is
* body.
*
* Usually this method is called internally by the JSF engine.
*/
void setTooltipContainer(String _tooltipContainer);
/**
* The tooltip is shown and hidden with a delay. This value is the delay in
* milliseconds. Defaults to 0 (no delay).
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
int getTooltipDelay();
/**
* The tooltip is shown and hidden with a delay. This value is the delay in
* milliseconds. Defaults to 0 (no delay).
*
* Usually this method is called internally by the JSF engine.
*/
void setTooltipDelay(int _tooltipDelay);
/**
* The tooltip is hidden with a delay. This value is the delay in
* milliseconds. Defaults to 0 (no delay).
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
int getTooltipDelayHide();
/**
* The tooltip is hidden with a delay. This value is the delay in
* milliseconds. Defaults to 0 (no delay).
*
* Usually this method is called internally by the JSF engine.
*/
void setTooltipDelayHide(int _tooltipDelayHide);
/**
* The tooltip is shown with a delay. This value is the delay in
* milliseconds. Defaults to 0 (no delay).
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
int getTooltipDelayShow();
/**
* The tooltip is shown with a delay. This value is the delay in
* milliseconds. Defaults to 0 (no delay).
*
* Usually this method is called internally by the JSF engine.
*/
void setTooltipDelayShow(int _tooltipDelayShow);
/**
* Where is the tooltip to be displayed? Possible values: "top", "bottom",
* "right", "left", "auto", "auto top", "auto bottom", "auto right" and
* "auto left". Default to "bottom".
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getTooltipPosition();
/**
* Where is the tooltip to be displayed? Possible values: "top", "bottom",
* "right", "left", "auto", "auto top", "auto bottom", "auto right" and
* "auto left". Default to "bottom".
*
* Usually this method is called internally by the JSF engine.
*/
void setTooltipPosition(String _tooltipPosition);
/**
* Component(s) to be updated with ajax.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
String getUpdate();
/**
* Component(s) to be updated with ajax.
*
* Usually this method is called internally by the JSF engine.
*/
void setUpdate(String _update);
/**
* Boolean value to specify if the button is disabled.
*
*
* @return Returns the value of the attribute, or null, if it hasn't been
* set by the JSF file.
*/
boolean isDisabled();
/**
* Boolean value to specify if the button is disabled.
*
* Usually this method is called internally by the JSF engine.
*/
void setDisabled(boolean _disabled);
public Object getValue();
public boolean isRendered();
/**
* Boolean value: if true the icon will spin.
* @return Returns the value of the attribute, or null, if it hasn't been set by the JSF file.
*/
public boolean isIconSpin();
/**
* Flip the icon: can be H (horizontal) or V (vertical).
* @return Returns the value of the attribute, or null, if it hasn't been set by the JSF file.
*/
public String getIconFlip();
/**
* Rotate 90 degrees the icon: Can be L,R.
* @return Returns the value of the attribute, or null, if it hasn't been set by the JSF file.
*/
public String getIconRotate();
/**
* Icon Size: legal values are lg, 2x, 3x, 4x, 5x.
* @return Returns the value of the attribute, or null, if it hasn't been set by the JSF file.
*/
public String getIconSize();
/**
* Use the free brand font of FontAwesome 5. As a side effect, every FontAwesome icon on the same page is switched to FontAwesome 5.2.0. By default, the icon set is the older version 4.7.0.
* @return Returns the value of the attribute, or , false, if it hasn't been set by the JSF file.
*/
public boolean isIconBrand();
/**
* Switch the icon from black-on-white to white-on-black.
* @return Returns the value of the attribute, or , false, if it hasn't been set by the JSF file.
*/
public boolean isIconInverse();
/**
* Use the paid 'light' font of FontAwesome 5. As a side effect, every FontAwesome icon on the same page is switched to FontAwesome 5.2.0. By default, the icon set is the older version 4.7.0.
* @return Returns the value of the attribute, or , false, if it hasn't been set by the JSF file.
*/
public boolean isIconLight();
/**
* Boolean value: if true the icon will rotate with 8 discrete steps.
* @return Returns the value of the attribute, or , false, if it hasn't been set by the JSF file.
*/
public boolean isIconPulse();
/**
* Use the paid 'regular' font of FontAwesome 5. As a side effect, every FontAwesome icon on the same page is switched to FontAwesome 5.2.0. By default, the icon set is the older version 4.7.0.
* @return Returns the value of the attribute, or , false, if it hasn't been set by the JSF file.
*/
public boolean isIconRegular();
/**
* Use the free font of FontAwesome 5. As a side effect, every FontAwesome icon on the same page is switched to FontAwesome 5.2.0. By default, the icon set is the older version 4.7.0.
* @return Returns the value of the attribute, or , false, if it hasn't been set by the JSF file.
*/
public boolean isIconSolid();
/**
* Position of this element in the tabbing order for the current document. This value must be an integer between -1 and 32767. By default, Bootstrap uses 0, which means the tab order is relative to the position of the element in the document.
* @return Returns the value of the attribute, or "0", if it hasn't been set by the JSF file.
*/
public String getTabindex();
}