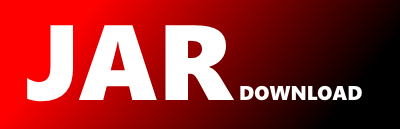
net.bytebuddy.utility.ByteBuddyCommons Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of byte-buddy Show documentation
Show all versions of byte-buddy Show documentation
Byte Buddy is a Java library for creating Java classes at run time.
This artifact is a build of Byte Buddy with all ASM dependencies repackaged into its own name space.
package net.bytebuddy.utility;
import net.bytebuddy.description.modifier.ModifierContributor;
import net.bytebuddy.description.type.TypeDescription;
import net.bytebuddy.description.type.generic.GenericTypeDescription;
import net.bytebuddy.jar.asm.Opcodes;
import java.lang.reflect.Modifier;
import java.util.*;
/**
* Represents a collection of common helper functions.
*/
public final class ByteBuddyCommons {
/**
* A mask for modifiers that represent a type's, method's or field's visibility.
*/
public static final int VISIBILITY_MODIFIER_MASK = Modifier.PUBLIC | Modifier.PROTECTED | Modifier.PRIVATE;
/**
* A mask for modifiers that are represented by types and members.
*/
public static final int GENERAL_MODIFIER_MASK = Opcodes.ACC_SYNTHETIC | Opcodes.ACC_DEPRECATED;
/**
* A mask for modifiers that represents types.
*/
public static final int TYPE_MODIFIER_MASK = VISIBILITY_MODIFIER_MASK | GENERAL_MODIFIER_MASK
| Modifier.ABSTRACT | Modifier.FINAL | Modifier.INTERFACE | Modifier.STRICT | Opcodes.ACC_ANNOTATION
| Opcodes.ACC_ENUM | Opcodes.ACC_STRICT | Opcodes.ACC_SUPER;
/**
* A mask for modifiers that represents type members.
*/
public static final int MEMBER_MODIFIER_MASK = VISIBILITY_MODIFIER_MASK | TYPE_MODIFIER_MASK
| Modifier.FINAL | Modifier.SYNCHRONIZED | Modifier.STATIC;
/**
* A mask for modifiers that represents fields.
*/
public static final int FIELD_MODIFIER_MASK = MEMBER_MODIFIER_MASK | Modifier.TRANSIENT | Modifier.VOLATILE;
/**
* A mask for modifiers that represents methods and constructors.
*/
public static final int METHOD_MODIFIER_MASK = MEMBER_MODIFIER_MASK | Modifier.ABSTRACT | Modifier.SYNCHRONIZED
| Modifier.NATIVE | Modifier.STRICT | Opcodes.ACC_BRIDGE | Opcodes.ACC_VARARGS;
/**
* A mask for modifiers that represents method parameters.
*/
public static final int PARAMETER_MODIFIER_MASK = Modifier.FINAL | Opcodes.ACC_MANDATED | Opcodes.ACC_SYNTHETIC;
/**
* A collection of all keywords of the Java programming language.
*/
private static final Set JAVA_KEYWORDS = Collections.unmodifiableSet(
new HashSet(Arrays.asList(
"abstract", "assert", "boolean", "break", "byte", "case",
"catch", "char", "class", "const", "continue", "default",
"double", "do", "else", "enum", "extends", "false",
"final", "finally", "float", "for", "goto", "if",
"implements", "import", "instanceof", "int", "interface", "long",
"native", "new", "null", "package", "private", "protected",
"public", "return", "short", "static", "strictfp", "super",
"switch", "synchronized", "this", "throw", "throws", "transient",
"true", "try", "void", "volatile", "while"))
);
/**
* A set of all generic type sorts that can possibly define an extendable type.
*/
private static final Set EXTENDABLE_TYPES = EnumSet.of(GenericTypeDescription.Sort.NON_GENERIC,
GenericTypeDescription.Sort.PARAMETERIZED);
/**
* This utility class is not supposed to be instantiated.
*/
private ByteBuddyCommons() {
throw new UnsupportedOperationException("This type describes a utility and is not supposed to be instantiated");
}
/**
* Validates that a value is not {@code null}.
*
* @param value The input value to be validated.
* @param The type of the input value.
* @return The input value.
*/
public static T nonNull(T value) {
if (value == null) {
throw new NullPointerException();
}
return value;
}
/**
* Validates that no value of an array is {@code null}.
*
* @param value The input value to be validated.
* @param The component type of the input value.
* @return The input value.
*/
public static T[] nonNull(T[] value) {
for (T object : value) {
nonNull(object);
}
return value;
}
/**
* Validates that a type is an annotation type.
*
* @param typeDescription The type to validate.
* @param The type of the input value.
* @return The input value.
*/
public static T isAnnotation(T typeDescription) {
if (!typeDescription.isAnnotation()) {
throw new IllegalArgumentException(typeDescription + " is not an annotation type");
}
return typeDescription;
}
/**
* Validates that a type is a throwable type.
*
* @param typeDescription The type to validate.
* @param The type of the input value.
* @return The input value.
*/
public static T isThrowable(T typeDescription) {
if (!isActualType(typeDescription).asErasure().isAssignableTo(Throwable.class)) {
throw new IllegalArgumentException("Cannot throw instances of: " + typeDescription);
}
return typeDescription;
}
/**
* Validates that a collection of types only contains throwable types.
*
* @param typeDescriptions The types to validate.
* @param The type of the input value.
* @return The input value.
*/
public static > T isThrowable(T typeDescriptions) {
for (GenericTypeDescription typeDescription : typeDescriptions) {
isThrowable(typeDescription);
}
return typeDescriptions;
}
/**
* Validates that a type is defineable, i.e. is represented as byte code.
*
* @param typeDescription The type to validate.
* @param The actual type of the validated instance.
* @return The input value.
*/
public static T isDefineable(T typeDescription) {
if (typeDescription.isArray()) {
throw new IllegalArgumentException("Cannot explicitly define an array type: " + typeDescription);
} else if (typeDescription.isPrimitive()) {
throw new IllegalArgumentException("Cannot explicitly define a primitive type: " + typeDescription);
}
return typeDescription;
}
/**
* Validates that a type is extendable, i.e. is not an array, a primitive type or a {@code final} type.
*
* @param typeDescription The type to validate.
* @param The actual type of the validated instance.
* @return The input value.
*/
public static T isExtendable(T typeDescription) {
if (!EXTENDABLE_TYPES.contains(typeDescription.getSort())) {
throw new IllegalArgumentException("Cannot extend generic type: " + typeDescription);
} else if (isDefineable(typeDescription.asErasure()).isFinal()) {
throw new IllegalArgumentException("Cannot extend a final type: " + typeDescription);
}
return typeDescription;
}
/**
* Verifies that a type can be implemented.
*
* @param typeDescription The type to verify.
* @param The actual type of the input.
* @return The input value.
*/
public static T isImplementable(T typeDescription) {
if (!isExtendable(typeDescription).asErasure().isInterface()) {
throw new IllegalArgumentException("Not an interface: " + typeDescription);
}
return typeDescription;
}
/**
* Verifies that a collection of types can be implemented.
*
* @param typeDescriptions The types to verify.
* @param The actual type of the input.
* @return The input value.
*/
public static > T isImplementable(T typeDescriptions) {
for (GenericTypeDescription typeDescription : typeDescriptions) {
isImplementable(typeDescription);
}
return typeDescriptions;
}
/**
* Validates that a type represents an actual type, i.e. not a wildcard and not {@code void}.
*
* @param typeDescription The type to validate.
* @param The actual type of the argument.
* @return The input value.
*/
public static T isActualType(T typeDescription) {
if (isActualTypeOrVoid(typeDescription).represents(void.class)) {
throw new IllegalArgumentException("The void non-type cannot be assigned a value");
}
return typeDescription;
}
/**
* Validates that a collection of types represents an actual type, i.e. not a wildcard and not {@code void}.
*
* @param typeDescriptions The types to validate.
* @param The actual type of the argument.
* @return The input value.
*/
public static > T isActualType(T typeDescriptions) {
for (GenericTypeDescription typeDescription : typeDescriptions) {
isActualType(typeDescription);
}
return typeDescriptions;
}
/**
* Validates that a type represents an actual type or {@code void}, i.e. not a wildcard.
*
* @param typeDescription The type to validate.
* @param The actual type of the argument.
* @return The input value.
*/
public static T isActualTypeOrVoid(T typeDescription) {
if (typeDescription.getSort().isWildcard()) {
throw new IllegalArgumentException("Not a top-level type: " + typeDescription);
}
return typeDescription;
}
/**
* Validates that a collection of types represents an actual type or {@code void}, i.e. not a wildcard.
*
* @param typeDescriptions The types to validate.
* @param The actual type of the argument.
* @return The input value.
*/
public static > T isActualTypeOrVoid(T typeDescriptions) {
for (GenericTypeDescription typeDescription : typeDescriptions) {
isActualTypeOrVoid(typeDescription);
}
return typeDescriptions;
}
/**
* Validates that the given collection of elements is unique.
*
* @param elements The elements to validate for being unique.
* @param The actual type.
* @return The given value.
*/
public static > T unique(T elements) {
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy