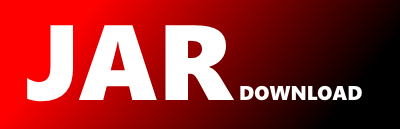
net.cadrian.jsonref.JsonConverter Maven / Gradle / Ivy
The newest version!
/*
Copyright 2015 Cyril Adrian
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package net.cadrian.jsonref;
import java.beans.PropertyDescriptor;
import java.lang.reflect.Field;
import java.util.Collection;
import java.util.Map;
/**
* Customizable JSON/R converter for "atomic" types
*/
public interface JsonConverter {
public static interface Context {
/**
* @return the property descriptor
*/
PropertyDescriptor getPropertyDescriptor();
/**
* @return the property field
*/
Field getPropertyField();
/**
* @param propertyDescriptor
* the new property descriptor
* @param propertyField
* the new property field
* @return a context identical to this
but for the property
* descriptor and field
*/
Context withProperty(PropertyDescriptor propertyDescriptor,
Field propertyField);
}
/**
* Get a new context to be passed around during the serialization
*
* @return the new context
*/
Context getNewContext();
/**
* Convert the atomic value to a valid JSON string
*
* @param value
* the value
* @return the converted value
*/
String toJson(Object value);
/**
* Convert the JSON string to an atomic value
*
* @param string
* the JSON string
* @param propertyType
* the expected class
* @param
* the returned object type
* @return the object
*/
T fromJson(String string, Class extends T> propertyType);
/**
* Is the type considered a type of atomic values?
*
* @param propertyType
* the type to check
* @return true
if the type is of atomic values,
* false
otherwise
*/
boolean isAtomicValue(Class> propertyType);
/**
* Create a new collection of the most appropriate type.
*
* @param wantedType
* the collection's required type
* @return the new collection
*/
Collection> newCollection(
@SuppressWarnings("rawtypes") Class wantedType);
/**
* Create a new map of the most appropriate type.
*
* @param wantedType
* the map's required type
* @return the new map
*/
Map, ?> newMap(@SuppressWarnings("rawtypes") Class
© 2015 - 2025 Weber Informatics LLC | Privacy Policy